在Python字符串里添加字符的方法包括使用字符串插值、字符串拼接、列表转换等。 在这些方法中,字符串插值是最灵活和强大的,它使得代码更具可读性和维护性。下面将详细描述如何使用字符串插值来添加字符。
字符串插值(即f-string)是Python 3.6及其以上版本的新特性,使用大括号 {} 包裹变量或表达式,然后用f前缀标记字符串。它提供了一个简单而灵活的方式来格式化字符串,并且比传统的字符串拼接和格式化方法更高效。
name = "world"
greeting = f"Hello, {name}!"
print(greeting)
在这个示例中,{name}
被替换为变量 name
的值,使得最终的字符串变为 "Hello, world!"
。这种方法不仅支持变量替换,还支持表达式计算。
一、字符串插值
字符串插值是一种将变量和表达式嵌入到字符串中的方法。在Python中,最常用的字符串插值方法是f-string。f-string是一种更简洁、更高效的字符串格式化方法。
name = "Alice"
age = 30
introduction = f"My name is {name} and I am {age} years old."
print(introduction)
在这个例子中,f-string {name}
和 {age}
被替换为变量 name
和 age
的值,使得最终的字符串变为 "My name is Alice and I am 30 years old."
。
除了简单的变量替换,f-string还支持表达式计算。例如:
a = 5
b = 10
result = f"The result of {a} + {b} is {a + b}."
print(result)
这种方法不仅简洁,而且提高了代码的可读性和可维护性。
二、字符串拼接
字符串拼接是将两个或多个字符串连接在一起的方法。最常用的字符串拼接方法是使用加号(+)运算符。
part1 = "Hello"
part2 = "World"
greeting = part1 + " " + part2 + "!"
print(greeting)
在这个例子中,字符串 part1
和 part2
被加号运算符连接在一起,使得最终的字符串变为 "Hello World!"
。
虽然这种方法简单易懂,但当需要拼接的字符串很多时,代码会变得冗长且不易读。因此,在这种情况下,使用join方法可能会更合适。
words = ["Hello", "World", "!"]
greeting = " ".join(words)
print(greeting)
使用join方法可以将列表中的字符串按指定的分隔符连接在一起,代码更简洁。
三、使用列表转换
有时候,字符串拼接或插值可能无法满足复杂的需求。这时,可以将字符串转换为列表进行操作,然后再将其转换回字符串。
original_string = "HelloWorld"
string_list = list(original_string)
string_list.insert(5, " ")
modified_string = "".join(string_list)
print(modified_string)
在这个例子中,原始字符串 original_string
被转换为列表 string_list
,然后在索引5的位置插入一个空格字符,最后再将列表转换回字符串 modified_string
。
这种方法虽然灵活,但代码较为复杂,不推荐在简单情况下使用。
四、正则表达式
正则表达式是一种强大的字符串处理工具。通过正则表达式,可以在字符串中匹配、替换特定的模式。
import re
original_string = "HelloWorld"
pattern = re.compile(r"(\w{5})")
modified_string = pattern.sub(r"\1 ", original_string)
print(modified_string)
在这个例子中,正则表达式 (\w{5})
匹配前5个字符,并在匹配的字符后面添加一个空格。
正则表达式虽然强大,但语法复杂,不建议在简单情况下使用。
五、格式化字符串
格式化字符串是一种将变量值插入到字符串中的方法。最常用的格式化字符串方法是使用百分号(%)运算符和format方法。
name = "Alice"
age = 30
introduction = "My name is %s and I am %d years old." % (name, age)
print(introduction)
在这个例子中,格式化字符串 %s
和 %d
被变量 name
和 age
的值替换,使得最终的字符串变为 "My name is Alice and I am 30 years old."
。
虽然这种方法简单易懂,但不如f-string灵活和高效。因此,推荐使用f-string代替格式化字符串。
在这篇文章中,我们详细讨论了几种在Python字符串里添加字符的方法,包括字符串插值、字符串拼接、列表转换、正则表达式和格式化字符串。每种方法都有其优缺点,具体选择哪种方法取决于具体的使用场景和需求。总体来说,字符串插值(f-string) 是最推荐的方法,因为它简洁、灵活且高效。
相关问答FAQs:
在Python中,如何在字符串的特定位置插入字符?
在Python中,字符串是不可变的,因此无法直接在现有字符串中插入字符。不过,可以通过切片的方式来实现这一点。比如,如果您想在字符串的第n个位置插入一个字符,可以这样做:使用字符串的切片功能,将字符串分为两部分,然后在中间添加您想插入的字符,最后将这三部分连接起来。示例代码如下:
original_string = "Hello World"
position = 5
char_to_add = "!"
new_string = original_string[:position] + char_to_add + original_string[position:]
print(new_string) # 输出: Hello! World
在Python中如何使用字符串方法来添加字符?
Python提供了多种字符串方法,可以帮助您轻松添加字符。例如,您可以使用join()
方法来将多个字符串连接起来,这样也可以在字符串之间添加字符。示例代码如下:
strings = ["Hello", "World"]
separator = " "
new_string = separator.join(strings)
print(new_string) # 输出: Hello World
此外,您也可以使用format()
或f-string(Python 3.6及以上版本)来插入字符,示例代码如下:
name = "World"
new_string = f"Hello, {name}!"
print(new_string) # 输出: Hello, World!
在Python中是否可以使用列表来构建字符串并添加字符?
是的,您可以将字符串转换为列表,添加字符后再将其转换回字符串。这样做的好处是可以进行更复杂的字符串操作。示例代码如下:
original_string = "Hello World"
char_to_add = "!"
string_list = list(original_string)
string_list.insert(5, char_to_add) # 在第5个位置插入字符
new_string = ''.join(string_list)
print(new_string) # 输出: Hello! World
这种方法特别适合需要进行多次插入或删除操作的场景。
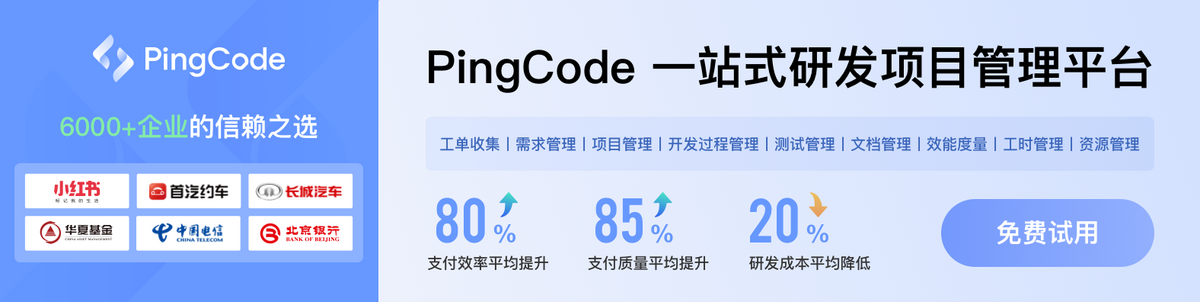