如何使用Python写一个小软件下载
使用Python写一个小软件下载的关键点包括:选择适当的库、实现文件下载、处理并发下载、实现进度显示。这些步骤可以帮助我们在Python中实现一个高效、可靠的小软件下载工具。本文将详细介绍每个步骤,并提供完整的代码示例。
一、选择适当的库
在Python中,有许多库可以用来实现文件下载功能。常用的库包括requests
、urllib
、和aiohttp
。其中,requests
库以其简单易用的API和强大的功能成为最受欢迎的选择。
1、使用requests库
requests
库是一个简洁且强大的HTTP库,适用于各种网络请求。它的语法简单直观,非常适合初学者和专业开发者。
import requests
url = 'https://example.com/file.zip'
response = requests.get(url)
with open('file.zip', 'wb') as file:
file.write(response.content)
2、使用urllib库
urllib
是Python标准库的一部分,无需额外安装。虽然功能强大,但它的API相对复杂。
import urllib.request
url = 'https://example.com/file.zip'
urllib.request.urlretrieve(url, 'file.zip')
3、使用aiohttp库
aiohttp
是一个异步HTTP库,适用于需要处理并发请求的场景。
import aiohttp
import asyncio
async def download_file(url, filename):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
with open(filename, 'wb') as file:
file.write(await response.read())
url = 'https://example.com/file.zip'
filename = 'file.zip'
asyncio.run(download_file(url, filename))
二、实现文件下载
在选择合适的库后,我们需要实现文件下载功能。以下是使用requests
库实现文件下载的完整代码示例。
import requests
def download_file(url, filename):
response = requests.get(url, stream=True)
total_size = int(response.headers.get('content-length', 0))
block_size = 1024
with open(filename, 'wb') as file:
for data in response.iter_content(block_size):
file.write(data)
print(f'{filename} downloaded successfully.')
url = 'https://example.com/file.zip'
filename = 'file.zip'
download_file(url, filename)
三、处理并发下载
在处理多个文件下载时,并发下载可以显著提高效率。我们可以使用concurrent.futures
库来实现并发下载。
import concurrent.futures
import requests
def download_file(url, filename):
response = requests.get(url, stream=True)
total_size = int(response.headers.get('content-length', 0))
block_size = 1024
with open(filename, 'wb') as file:
for data in response.iter_content(block_size):
file.write(data)
print(f'{filename} downloaded successfully.')
urls = [
('https://example.com/file1.zip', 'file1.zip'),
('https://example.com/file2.zip', 'file2.zip'),
('https://example.com/file3.zip', 'file3.zip')
]
with concurrent.futures.ThreadPoolExecutor() as executor:
futures = [executor.submit(download_file, url, filename) for url, filename in urls]
for future in concurrent.futures.as_completed(futures):
future.result()
四、实现进度显示
在下载过程中显示进度条可以提升用户体验。我们可以使用tqdm
库来实现进度显示。
import requests
from tqdm import tqdm
def download_file(url, filename):
response = requests.get(url, stream=True)
total_size = int(response.headers.get('content-length', 0))
block_size = 1024
progress_bar = tqdm(total=total_size, unit='iB', unit_scale=True)
with open(filename, 'wb') as file:
for data in response.iter_content(block_size):
progress_bar.update(len(data))
file.write(data)
progress_bar.close()
print(f'{filename} downloaded successfully.')
url = 'https://example.com/file.zip'
filename = 'file.zip'
download_file(url, filename)
五、处理错误和异常
在实际应用中,网络请求可能会出现各种错误。我们需要处理这些错误,以确保程序的稳定性。
import requests
from requests.exceptions import HTTPError, Timeout, RequestException
from tqdm import tqdm
def download_file(url, filename):
try:
response = requests.get(url, stream=True, timeout=10)
response.raise_for_status()
total_size = int(response.headers.get('content-length', 0))
block_size = 1024
progress_bar = tqdm(total=total_size, unit='iB', unit_scale=True)
with open(filename, 'wb') as file:
for data in response.iter_content(block_size):
progress_bar.update(len(data))
file.write(data)
progress_bar.close()
print(f'{filename} downloaded successfully.')
except HTTPError as http_err:
print(f'HTTP error occurred: {http_err}')
except Timeout as timeout_err:
print(f'Timeout error occurred: {timeout_err}')
except RequestException as req_err:
print(f'Error occurred: {req_err}')
url = 'https://example.com/file.zip'
filename = 'file.zip'
download_file(url, filename)
六、优化和扩展
1、支持断点续传
断点续传可以在下载中断时继续下载未完成的部分。我们可以通过HTTP头中的Range
字段实现这一功能。
import os
import requests
from tqdm import tqdm
def download_file(url, filename):
headers = {}
if os.path.exists(filename):
headers['Range'] = f'bytes={os.path.getsize(filename)}-'
response = requests.get(url, headers=headers, stream=True)
total_size = int(response.headers.get('content-length', 0)) + os.path.getsize(filename)
block_size = 1024
progress_bar = tqdm(total=total_size, unit='iB', unit_scale=True, initial=os.path.getsize(filename))
with open(filename, 'ab') as file:
for data in response.iter_content(block_size):
progress_bar.update(len(data))
file.write(data)
progress_bar.close()
print(f'{filename} downloaded successfully.')
url = 'https://example.com/file.zip'
filename = 'file.zip'
download_file(url, filename)
2、支持多线程下载
多线程下载可以进一步提升下载速度。我们可以将文件分块,使用多个线程同时下载不同的块,然后合并这些块。
import os
import requests
from concurrent.futures import ThreadPoolExecutor
from tqdm import tqdm
def download_chunk(url, start, end, filename, chunk_num):
headers = {'Range': f'bytes={start}-{end}'}
response = requests.get(url, headers=headers, stream=True)
with open(f'{filename}.part{chunk_num}', 'wb') as file:
file.write(response.content)
def download_file(url, filename, num_threads=4):
response = requests.head(url)
total_size = int(response.headers.get('content-length', 0))
chunk_size = total_size // num_threads
with ThreadPoolExecutor(max_workers=num_threads) as executor:
futures = []
for i in range(num_threads):
start = i * chunk_size
end = start + chunk_size - 1 if i != num_threads - 1 else total_size - 1
futures.append(executor.submit(download_chunk, url, start, end, filename, i))
for future in tqdm(futures, total=len(futures)):
future.result()
with open(filename, 'wb') as output_file:
for i in range(num_threads):
with open(f'{filename}.part{i}', 'rb') as part_file:
output_file.write(part_file.read())
os.remove(f'{filename}.part{i}')
print(f'{filename} downloaded successfully.')
url = 'https://example.com/file.zip'
filename = 'file.zip'
download_file(url, filename)
通过以上步骤,我们可以使用Python实现一个功能强大、高效的小软件下载工具。无论是单线程下载、并发下载、还是多线程下载,Python都提供了丰富的库和方法来满足不同的需求。希望本文对您有所帮助。
相关问答FAQs:
如何选择适合的小程序框架来开发Python软件下载?
在开发Python软件下载时,选择合适的框架至关重要。常见的框架有Tkinter、PyQt和Kivy等。Tkinter适合简单的桌面应用,易于上手;PyQt则提供了丰富的组件和更复杂的界面设计,适合需要高度自定义的应用;Kivy则适合跨平台开发,能在Windows、Mac、Linux及移动设备上运行。根据项目需求和目标用户选择合适的框架,可以提高开发效率和用户体验。
Python软件下载后如何进行安装和配置?
用户下载Python软件后,通常需要进行安装和配置。一般情况下,软件会提供安装向导,用户只需按照提示进行操作。对于一些需要依赖库的程序,确保在安装前已经安装好Python环境,并使用pip安装所需的第三方库。在配置过程中,用户可能需要设置环境变量,以便在命令行中方便地调用软件。
如何确保Python下载软件的安全性和稳定性?
确保下载软件的安全性和稳定性非常重要。首先,用户应从官方网站或可信赖的源下载软件,以避免恶意软件。其次,定期更新软件版本,确保使用最新的安全补丁和功能。此外,用户可以查看其他用户的评价和反馈,了解软件的使用情况和稳定性,帮助做出明智的选择。
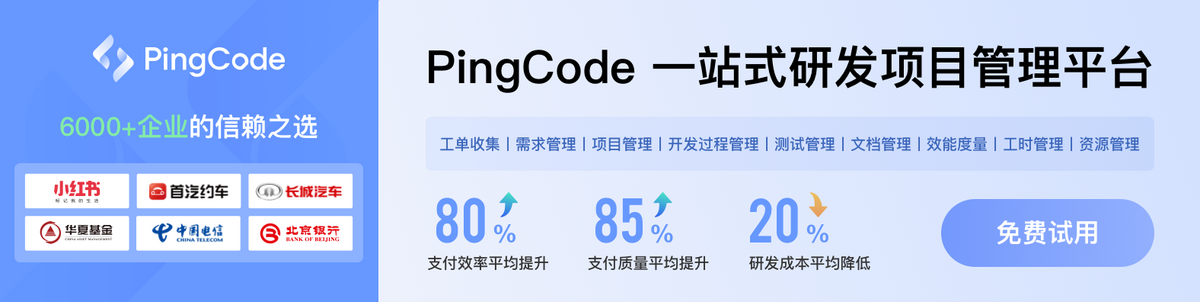