在Python3中打开文件可以使用内置的open()
函数。可以通过使用open()
函数、使用正确的模式、确保文件的正确关闭来完成文件操作。最常用的方法是通过上下文管理器with
语句,这样可以确保文件在使用完毕后自动关闭。使用with
语句可以避免手动关闭文件的麻烦,并且在处理文件异常时更加优雅。
详细描述:使用with
语句来打开文件时,即使在代码块内发生异常,文件也会被自动关闭。with
语句提供了一种更简洁和优雅的方式来处理文件打开和关闭的过程,从而减少了资源泄漏的风险。例如:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
在这个示例中,文件example.txt
以只读模式打开,读取其内容并打印到控制台。一旦with
块内的代码执行完毕,文件会自动关闭。
以下内容将详细介绍Python3中打开和处理文件的多种方式和注意事项。
一、打开文件的基本方法
在Python3中,打开文件的基本方法是使用open()
函数。该函数可以打开现有文件以进行读取、写入或追加操作,甚至可以创建一个新文件。
1、使用open()函数
open()
函数的基本语法如下:
open(file, mode='r', buffering=-1, encoding=None, errors=None, newline=None, closefd=True, opener=None)
- file: 要打开的文件的路径。
- mode: 打开文件的模式。常用的模式有:
'r'
: 只读(默认)。'w'
: 写入(会覆盖文件)。'a'
: 追加(在文件末尾追加内容)。'b'
: 以二进制模式打开文件。'+'
: 更新(读写)。
- buffering: 设置缓冲策略。默认值为-1,表示使用系统默认缓冲策略。
- encoding: 文件的编码方式,如
'utf-8'
。 - errors: 错误处理方式。
- newline: 控制新行字符的处理。
- closefd: 如果传递的file参数是文件描述符而不是文件名时,这个参数控制是否在文件关闭时关闭文件描述符。
- opener: 自定义的文件打开器。
2、常用文件模式
- 只读模式 (
'r'
): 打开文件进行读取。如果文件不存在,会引发FileNotFoundError
。 - 写入模式 (
'w'
): 打开文件进行写入。如果文件存在,会覆盖文件。如果文件不存在,会创建一个新文件。 - 追加模式 (
'a'
): 打开文件进行追加。如果文件存在,写入的数据将被附加到文件末尾。如果文件不存在,会创建一个新文件。 - 二进制模式 (
'b'
): 以二进制模式打开文件,用于处理非文本文件(如图像、音频文件)。 - 读写模式 (
'r+'
): 打开文件进行读写操作。 - 写入和读写模式 (
'w+'
): 打开文件进行读写操作,如果文件存在,会覆盖文件。如果文件不存在,会创建一个新文件。 - 追加和读写模式 (
'a+'
): 打开文件进行读写操作,写入的数据将被附加到文件末尾。如果文件不存在,会创建一个新文件。
二、文件操作的具体方法
1、读取文件内容
读取文件内容有多种方法,包括read()
, readline()
, 和 readlines()
。
1.1、使用read()方法
read()
方法读取整个文件的内容并返回一个字符串。
with open('example.txt', 'r') as file:
content = file.read()
print(content)
1.2、使用readline()方法
readline()
方法逐行读取文件内容,适合处理大文件或需要逐行处理的情况。
with open('example.txt', 'r') as file:
line = file.readline()
while line:
print(line, end='')
line = file.readline()
1.3、使用readlines()方法
readlines()
方法读取文件的所有行并返回一个列表,每个元素是一行内容。
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line, end='')
2、写入文件内容
写入文件可以使用write()
和writelines()
方法。
2.1、使用write()方法
write()
方法将字符串写入文件中。
with open('example.txt', 'w') as file:
file.write("Hello, World!")
2.2、使用writelines()方法
writelines()
方法将一个字符串列表写入文件中。
lines = ["Hello, World!\n", "Welcome to Python programming.\n"]
with open('example.txt', 'w') as file:
file.writelines(lines)
3、追加文件内容
可以使用追加模式('a'
)来追加文件内容。
with open('example.txt', 'a') as file:
file.write("This is an appended line.\n")
三、文件处理的高级技巧
1、处理文件路径
使用os.path
模块提供的函数来处理文件路径,例如os.path.join()
、os.path.exists()
、os.path.abspath()
等。
import os
file_path = os.path.join('folder', 'example.txt')
if os.path.exists(file_path):
print(f"File {file_path} exists.")
else:
print(f"File {file_path} does not exist.")
2、使用不同的文件编码
在处理不同语言或字符集的文件时,可以指定文件的编码方式。
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
3、处理文件异常
使用try-except
块来捕获文件操作中的异常,确保程序不会因为文件问题而崩溃。
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print("File not found.")
except IOError:
print("An error occurred while reading the file.")
4、文件指针的移动
可以使用seek()
方法来移动文件指针,使用tell()
方法来获取当前文件指针的位置。
with open('example.txt', 'r') as file:
file.seek(10) # 移动到第10个字节
content = file.read()
print(content)
position = file.tell() # 获取当前文件指针的位置
print(f"Current file pointer position: {position}")
四、文件操作的实际应用
1、读取CSV文件
使用csv
模块来读取和写入CSV文件。
import csv
with open('data.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
2、处理JSON文件
使用json
模块来读取和写入JSON文件。
import json
with open('data.json', 'r') as jsonfile:
data = json.load(jsonfile)
print(data)
写入JSON文件:
import json
data = {'name': 'John', 'age': 30}
with open('data.json', 'w') as jsonfile:
json.dump(data, jsonfile)
3、压缩和解压文件
使用zipfile
模块来压缩和解压文件。
import zipfile
压缩文件
with zipfile.ZipFile('example.zip', 'w') as zipf:
zipf.write('example.txt')
解压文件
with zipfile.ZipFile('example.zip', 'r') as zipf:
zipf.extractall('extracted_folder')
4、读写二进制文件
处理二进制文件时,需要使用'b'
模式。
# 读取二进制文件
with open('image.jpg', 'rb') as file:
data = file.read()
print(data)
写入二进制文件
with open('new_image.jpg', 'wb') as file:
file.write(data)
五、文件操作的最佳实践
1、使用上下文管理器
使用with
语句来确保文件在使用完毕后自动关闭。
with open('example.txt', 'r') as file:
content = file.read()
print(content)
2、处理文件异常
在文件操作中使用try-except
块来捕获并处理异常。
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print("File not found.")
except IOError:
print("An error occurred while reading the file.")
3、使用合适的文件模式
根据具体需求选择合适的文件模式(如只读、写入、追加、二进制等),避免不必要的文件覆盖或数据丢失。
4、处理文件路径
使用os.path
模块来处理文件路径,确保代码在不同操作系统上运行良好。
import os
file_path = os.path.join('folder', 'example.txt')
if os.path.exists(file_path):
print(f"File {file_path} exists.")
else:
print(f"File {file_path} does not exist.")
5、处理大文件
在处理大文件时,避免一次性读取整个文件内容。可以使用逐行读取或者分块读取的方式来处理大文件,避免内存占用过高。
with open('large_file.txt', 'r') as file:
for line in file:
process(line)
六、文件操作的高级应用
1、文件锁定
在并发环境中,可以使用文件锁定机制来避免多个进程同时修改同一个文件。可以使用fcntl
模块来实现文件锁定。
import fcntl
with open('example.txt', 'a') as file:
fcntl.flock(file, fcntl.LOCK_EX) # 加独占锁
file.write("This is a locked write.\n")
fcntl.flock(file, fcntl.LOCK_UN) # 解锁
2、使用临时文件
使用tempfile
模块来创建临时文件,适用于需要临时存储数据的场景。
import tempfile
with tempfile.TemporaryFile() as temp_file:
temp_file.write(b'This is some temporary data.')
temp_file.seek(0)
print(temp_file.read())
3、处理文件元数据
使用os
模块来获取和修改文件的元数据,如文件大小、创建时间、修改时间等。
import os
file_path = 'example.txt'
file_size = os.path.getsize(file_path)
creation_time = os.path.getctime(file_path)
modification_time = os.path.getmtime(file_path)
print(f"File Size: {file_size} bytes")
print(f"Creation Time: {creation_time}")
print(f"Modification Time: {modification_time}")
4、文件权限管理
使用os
模块来获取和设置文件的权限。
import os
file_path = 'example.txt'
os.chmod(file_path, 0o755) # 设置文件权限为755(rwxr-xr-x)
file_permissions = oct(os.stat(file_path).st_mode)[-3:]
print(f"File Permissions: {file_permissions}")
5、文件监控
使用watchdog
模块来监控文件和目录的变化。
from watchdog.observers import Observer
from watchdog.events import FileSystemEventHandler
class MyHandler(FileSystemEventHandler):
def on_modified(self, event):
print(f"File modified: {event.src_path}")
def on_created(self, event):
print(f"File created: {event.src_path}")
def on_deleted(self, event):
print(f"File deleted: {event.src_path}")
observer = Observer()
observer.schedule(MyHandler(), path='.', recursive=True)
observer.start()
try:
while True:
time.sleep(1)
except KeyboardInterrupt:
observer.stop()
observer.join()
通过上述方法,我们可以在Python3中灵活地进行文件操作,处理各种文件相关的任务。了解并掌握这些方法和技巧,将有助于编写更高效、更可靠的文件处理代码。
相关问答FAQs:
如何在Python3中打开一个文本文件?
在Python3中,可以使用内置的open()
函数来打开文本文件。该函数接受两个主要参数:文件名和模式。常用的模式包括'r'
(读取)、'w'
(写入)和'a'
(追加)。例如,要以读取模式打开名为example.txt
的文件,可以使用以下代码:
file = open('example.txt', 'r')
记得在操作完成后使用file.close()
关闭文件,以释放系统资源。
在Python3中,如何处理文件打开时的异常?
在处理文件时,可能会遇到文件不存在或权限不足等问题。为了确保程序的健壮性,建议使用try-except
块来捕捉异常。例如:
try:
file = open('example.txt', 'r')
except FileNotFoundError:
print("文件未找到,请检查文件名和路径。")
except PermissionError:
print("没有权限访问该文件。")
这样可以有效地处理打开文件时的潜在错误。
Python3中如何使用with
语句打开文件?
使用with
语句可以简化文件操作并确保文件在使用后自动关闭。通过with
语句打开文件时,不需要显式调用close()
方法,代码示例如下:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
这种方式不仅提高了代码的可读性,也降低了因未关闭文件而导致的资源泄露风险。
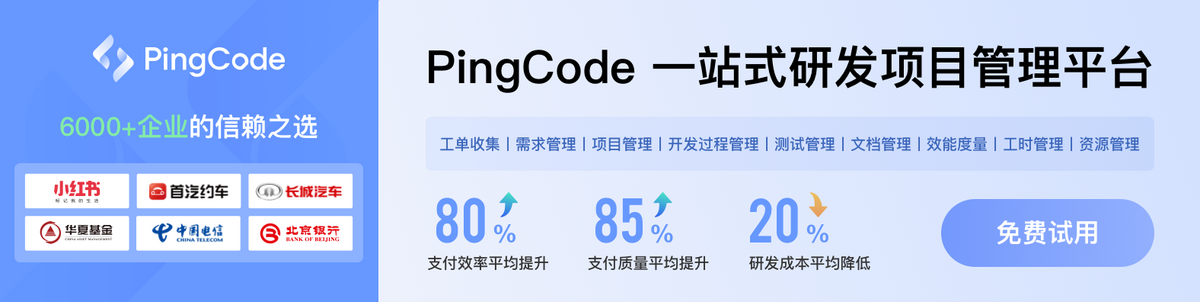