在Windows中运行Python线程的方法包括:使用threading
模块、定义线程函数或继承Thread
类、启动线程、使用join
方法等待线程完成。 在Windows操作系统中,Python提供了多种方式来实现多线程,这主要得益于其强大的threading
模块。threading
模块允许开发者创建和管理线程,从而有效地利用多核CPU的优势。下面将详细介绍如何在Windows中运行Python线程。
一、THREADING
模块介绍
threading
模块是Python标准库中用于多线程的模块。它提供了一些简单易用的接口来创建和管理线程。Python中的线程是操作系统原生线程的轻量级包装,因此能够充分利用多核CPU的能力。
-
创建线程
在使用
threading
模块时,创建线程的方式主要有两种:一种是直接使用Thread
类,另一种是通过继承Thread
类。- 直接使用
Thread
类:可以通过创建Thread
类的实例来定义一个新线程。需要传递目标函数和其参数。
import threading
def my_function(arg1, arg2):
print(f"Thread running with arguments: {arg1}, {arg2}")
创建一个线程
thread = threading.Thread(target=my_function, args=(10, 20))
- 继承
Thread
类:通过继承Thread
类并重写其run
方法,可以定义一个线程类。
import threading
class MyThread(threading.Thread):
def __init__(self, arg1, arg2):
super().__init__()
self.arg1 = arg1
self.arg2 = arg2
def run(self):
print(f"Thread running with arguments: {self.arg1}, {self.arg2}")
创建一个线程
thread = MyThread(10, 20)
- 直接使用
-
启动线程
一旦线程被创建,可以通过调用
start()
方法来启动线程。此方法会调用线程对象的run()
方法。thread.start()
-
等待线程结束
如果需要等待线程完成,可以使用
join()
方法。该方法会阻塞调用线程,直到被调用的线程完成。thread.join()
二、线程同步
在多线程编程中,线程同步是一个重要的概念。Python的threading
模块提供了一些同步机制,如锁(Lock)、条件变量(Condition)等。
-
使用锁
锁是一种同步原语,它可以确保某段代码在任何时刻只有一个线程能够访问。使用锁的典型场景是对共享资源的访问。
import threading
lock = threading.Lock()
shared_resource = 0
def thread_task():
global shared_resource
with lock:
# 对共享资源的访问
shared_resource += 1
print(shared_resource)
threads = [threading.Thread(target=thread_task) for _ in range(10)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
-
使用条件变量
条件变量用于线程之间的通信和协调。它允许一个线程等待一个条件的发生,并在条件满足时被其他线程唤醒。
import threading
condition = threading.Condition()
data_ready = False
def producer():
global data_ready
with condition:
data_ready = True
condition.notify_all()
def consumer():
with condition:
condition.wait_for(lambda: data_ready)
print("Data is ready")
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
consumer_thread.start()
producer_thread.start()
consumer_thread.join()
producer_thread.join()
三、线程安全
线程安全是指多线程环境下程序能够正确地执行。为了确保线程安全,通常需要在访问共享资源时使用同步机制。
-
原子操作
在Python中,某些操作是原子的,即它们在执行过程中不会被中断。这些操作通常是基本的数据类型操作,如整数加减、布尔运算等。
-
避免共享可变数据
在多线程编程中,共享可变数据是导致线程不安全的常见原因。避免共享可变数据是实现线程安全的一个简单有效的策略。
-
使用线程安全的数据结构
Python的
queue
模块提供了线程安全的队列,可以用于在线程之间传递数据。import queue
import threading
q = queue.Queue()
def producer():
for i in range(5):
q.put(i)
print(f"Produced {i}")
def consumer():
while True:
item = q.get()
if item is None:
break
print(f"Consumed {item}")
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
consumer_thread.start()
producer_thread.join()
q.put(None) # Signal the consumer to exit
consumer_thread.join()
四、线程池
为了管理大量线程,Python的concurrent.futures
模块提供了线程池接口。线程池可以简化线程管理,提高程序效率。
-
创建线程池
可以使用
ThreadPoolExecutor
类创建线程池。通过submit
方法提交任务,线程池会自动管理线程的创建和销毁。from concurrent.futures import ThreadPoolExecutor
def task(n):
return n * n
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(task, i) for i in range(10)]
results = [future.result() for future in futures]
print(results)
-
使用
map
方法ThreadPoolExecutor
还提供了map
方法,可以一次性提交多个任务,并返回结果迭代器。with ThreadPoolExecutor(max_workers=5) as executor:
results = executor.map(task, range(10))
print(list(results))
五、性能优化
在使用线程时,性能优化是一个重要的考虑因素。虽然多线程可以提高程序的并发性,但不当的使用可能导致性能下降。
-
避免线程过多
线程过多会导致系统资源的浪费,并可能导致线程间的频繁上下文切换。通常,线程的数量应与CPU核心数相匹配。
-
使用线程池
线程池可以有效地管理线程的创建和销毁,减少线程管理的开销。
-
减少锁的使用
锁的使用会导致线程阻塞,从而降低程序的并发性。应尽量减少锁的使用,或使用更细粒度的锁。
通过合理地使用Python的threading
模块和相关同步机制,可以在Windows系统中高效地运行多线程程序。希望本文的介绍能为您在Python多线程编程中提供帮助。
相关问答FAQs:
如何在Windows环境下使用Python创建线程?
在Windows上使用Python创建线程非常简单,您可以使用内置的threading
模块。首先,您需要导入该模块,然后定义一个函数作为线程的目标。接着,通过threading.Thread
类实例化一个线程对象,最后调用start()
方法来启动线程。以下是一个简单的示例:
import threading
def thread_function(name):
print(f'Thread {name} is running')
thread = threading.Thread(target=thread_function, args=(1,))
thread.start()
多线程在Python中有什么优势?
使用多线程可以让您的程序同时执行多个任务,这在处理I/O密集型操作时尤其有效。例如,如果您在下载文件或进行网络请求,使用线程可以使这些操作并行执行,从而提高程序的效率。此外,线程可以共享内存空间,方便数据的共享和通信。
Python中的线程与进程有什么区别?
Python中的线程是轻量级的,适合用于I/O密集型的任务。而进程则是更重的资源占用,适合CPU密集型的计算任务。由于Python的全局解释器锁(GIL),多线程在执行CPU密集型任务时可能不会带来性能提升,而多进程可以利用多核CPU的优势。因此,在选择使用线程或进程时,要考虑任务的类型和需求。
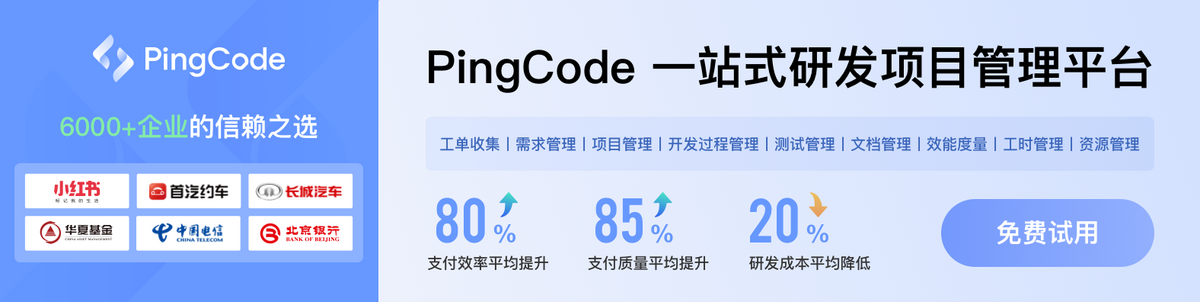