在Python下测试MySQL的方式有多种,包括使用MySQL Connector、SQLAlchemy、PyMySQL等库来连接和操作MySQL数据库、利用unittest或pytest进行单元测试、创建测试数据库以确保数据的独立性和一致性。其中,使用MySQL Connector是较为常见的方法之一,通过它可以方便地连接MySQL数据库,执行SQL语句,并获取结果。下面将详细介绍如何在Python中使用这些方法进行MySQL测试。
一、使用MYSQL CONNECTOR连接和测试
MySQL Connector是官方提供的Python库,用于连接和操作MySQL数据库。它支持Python 3.x,并且可以通过pip轻松安装。
安装MySQL Connector
要使用MySQL Connector,首先需要确保其已安装。可以通过以下命令安装:
pip install mysql-connector-python
连接MySQL数据库
在安装完成后,可以通过以下代码连接MySQL数据库:
import mysql.connector
def connect_to_mysql():
try:
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
if connection.is_connected():
print("Successfully connected to the database")
return connection
except mysql.connector.Error as err:
print(f"Error: {err}")
return None
这里需要提供MySQL服务器的主机名、用户名、密码和数据库名等信息。
执行SQL查询
连接成功后,可以执行SQL查询来测试数据库的功能。例如,查询某个表中的数据:
def fetch_data(connection):
try:
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table")
for row in cursor.fetchall():
print(row)
except mysql.connector.Error as err:
print(f"Error: {err}")
connection = connect_to_mysql()
if connection:
fetch_data(connection)
connection.close()
处理数据库事务
MySQL Connector支持事务管理,可以通过commit()
和rollback()
方法来提交或回滚事务:
def transaction_example(connection):
try:
cursor = connection.cursor()
cursor.execute("INSERT INTO your_table (column1, column2) VALUES (value1, value2)")
connection.commit()
print("Transaction committed.")
except mysql.connector.Error as err:
connection.rollback()
print(f"Transaction failed, rolled back. Error: {err}")
二、利用SQLALCHEMY进行高级数据库操作
SQLAlchemy是一个功能强大的SQL工具包和对象关系映射器(ORM),适用于Python。它可以提供更高层次的数据库操作抽象。
安装SQLAlchemy
可以通过以下命令安装SQLAlchemy:
pip install sqlalchemy
使用SQLAlchemy连接MySQL
利用SQLAlchemy连接MySQL需要设置连接字符串:
from sqlalchemy import create_engine
def create_sqlalchemy_engine():
engine = create_engine('mysql+mysqlconnector://your_username:your_password@localhost/your_database')
return engine
执行数据库查询
使用SQLAlchemy可以用更高级的方式来处理数据库查询:
from sqlalchemy.orm import sessionmaker
def fetch_data_with_sqlalchemy(engine):
Session = sessionmaker(bind=engine)
session = Session()
try:
result = session.execute("SELECT * FROM your_table")
for row in result:
print(row)
except Exception as e:
print(f"Error: {e}")
finally:
session.close()
engine = create_sqlalchemy_engine()
fetch_data_with_sqlalchemy(engine)
使用ORM映射表结构
SQLAlchemy的ORM功能允许将Python类映射到数据库表:
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy import Column, Integer, String
Base = declarative_base()
class YourTable(Base):
__tablename__ = 'your_table'
id = Column(Integer, primary_key=True)
column1 = Column(String)
column2 = Column(String)
def fetch_data_with_orm(session):
try:
results = session.query(YourTable).all()
for instance in results:
print(instance.column1, instance.column2)
except Exception as e:
print(f"Error: {e}")
Session = sessionmaker(bind=engine)
session = Session()
fetch_data_with_orm(session)
session.close()
三、使用PYMYSQL进行轻量级连接
PyMySQL是一个纯Python实现的MySQL客户端,可以用于轻量级的数据库操作。
安装PyMySQL
可以通过以下命令安装PyMySQL:
pip install PyMySQL
使用PyMySQL连接MySQL
连接MySQL的代码如下:
import pymysql
def connect_with_pymysql():
try:
connection = pymysql.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
print("Connected to the database using PyMySQL")
return connection
except pymysql.MySQLError as e:
print(f"Error: {e}")
return None
执行查询和事务
与MySQL Connector类似,可以执行查询和管理事务:
def fetch_data_pymysql(connection):
try:
with connection.cursor() as cursor:
cursor.execute("SELECT * FROM your_table")
result = cursor.fetchall()
for row in result:
print(row)
except pymysql.MySQLError as e:
print(f"Error: {e}")
connection = connect_with_pymysql()
if connection:
fetch_data_pymysql(connection)
connection.close()
处理事务
def transaction_pymysql(connection):
try:
with connection.cursor() as cursor:
cursor.execute("INSERT INTO your_table (column1, column2) VALUES (value1, value2)")
connection.commit()
print("Transaction committed.")
except pymysql.MySQLError as e:
connection.rollback()
print(f"Transaction failed, rolled back. Error: {e}")
四、使用UNITTEST或PYTEST进行单元测试
在开发过程中,单元测试是确保代码质量的重要手段。可以利用unittest或pytest库来对数据库操作进行测试。
使用unittest进行测试
import unittest
from your_module import connect_to_mysql, fetch_data
class TestDatabaseOperations(unittest.TestCase):
def setUp(self):
self.connection = connect_to_mysql()
def tearDown(self):
if self.connection:
self.connection.close()
def test_fetch_data(self):
result = fetch_data(self.connection)
self.assertIsNotNone(result)
if __name__ == '__main__':
unittest.main()
使用pytest进行测试
pytest是一个更为强大的测试框架,支持更简洁的测试编写:
import pytest
from your_module import connect_to_mysql, fetch_data
@pytest.fixture(scope='module')
def db_connection():
connection = connect_to_mysql()
yield connection
if connection:
connection.close()
def test_fetch_data(db_connection):
result = fetch_data(db_connection)
assert result is not None
五、创建测试数据库和数据
为了确保测试的独立性和一致性,可以创建一个单独的测试数据库。可以利用SQL脚本或ORM来创建和填充测试数据。
创建测试数据库
在MySQL中创建测试数据库的SQL脚本示例:
CREATE DATABASE test_database;
USE test_database;
CREATE TABLE test_table (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255),
age INT
);
INSERT INTO test_table (name, age) VALUES ('Alice', 30), ('Bob', 25);
在测试中使用测试数据库
在测试代码中可以指定连接到测试数据库:
def connect_to_test_mysql():
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='test_database'
)
return connection
通过以上步骤,您可以在Python环境下对MySQL进行全面的测试。无论是简单的数据库连接和查询,还是使用ORM进行复杂操作,亦或是通过单元测试来确保代码质量,以上方法都能为您提供有效的解决方案。
相关问答FAQs:
如何在Python中连接MySQL数据库?
要在Python中连接MySQL数据库,您可以使用mysql-connector-python
或PyMySQL
库。首先,您需要安装这些库,通过运行pip install mysql-connector-python
或pip install PyMySQL
。安装完成后,您可以使用如下代码连接数据库:
import mysql.connector
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
cursor = connection.cursor()
# 执行SQL语句
cursor.execute("SELECT * FROM your_table")
results = cursor.fetchall()
for row in results:
print(row)
cursor.close()
connection.close()
如何在Python中执行MySQL查询并获取结果?
在Python中执行MySQL查询非常简单。您可以通过创建一个游标对象来执行SQL语句,并使用fetchone()
或fetchall()
方法获取结果。示例如下:
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table")
results = cursor.fetchall() # 获取所有结果
for row in results:
print(row) # 输出每一行的内容
确保在执行完查询后关闭游标和连接,以释放资源。
如何在Python中处理MySQL异常?
在与MySQL数据库交互时,处理异常是非常重要的。您可以使用try-except
块来捕获和处理潜在的错误。例如:
try:
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if cursor:
cursor.close()
if connection:
connection.close()
这种方法可以确保即使发生错误,程序也能优雅地退出,并且资源能够被正确释放。
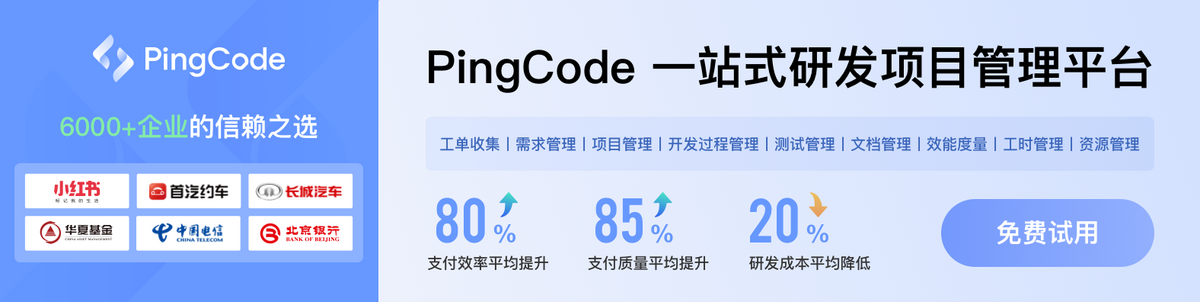