在Python中,打印画笔坐标可以通过使用turtle模块来实现、使用event bindings来追踪鼠标事件、使用定时器函数更新并打印坐标。
turtle模块提供了一个简单的方式去绘制图形,同时也能获取画笔的当前位置坐标。
在Python中,打印画笔坐标可以通过多种方式实现,以下是详细介绍:
一、使用TURTLE模块
Turtle是Python自带的一个模块,适合初学者学习编程和理解图形学的基本概念。它提供了一个简单易用的接口来绘制图形,同时也能追踪画笔的位置。
- 初始化Turtle环境
首先,确保你的Python环境已经安装了Turtle模块(通常Python自带)。我们需要导入这个模块并设置一个基本的绘图环境。
import turtle
创建一个Turtle对象
pen = turtle.Turtle()
设置窗口大小和背景色
screen = turtle.Screen()
screen.setup(width=600, height=600)
screen.bgcolor("white")
- 获取画笔坐标
Turtle模块提供了一个方法pen.position()
,可以返回当前画笔的坐标。我们可以利用这个方法在绘图过程中实时获取画笔的位置。
# 移动画笔到某个位置
pen.goto(100, 100)
获取当前画笔坐标
x, y = pen.position()
print(f"Current pen position: x={x}, y={y}")
- 动态显示坐标
可以通过事件绑定或定时器函数实时显示坐标。下面的例子展示了如何通过鼠标事件来更新和打印坐标。
def print_position(x, y):
print(f"Mouse clicked at position: x={x}, y={y}")
绑定鼠标点击事件
screen.onscreenclick(print_position)
保持窗口开启
turtle.done()
二、使用PYGAME模块
Pygame是一个流行的Python游戏开发模块,它提供了更强大的图形和事件处理功能。
- 初始化Pygame环境
首先,确保你的Python环境安装了Pygame模块。可以通过pip install pygame
来安装。
import pygame
from pygame.locals import *
初始化Pygame
pygame.init()
设置窗口大小
screen = pygame.display.set_mode((600, 600))
pygame.display.set_caption('Pygame Coordinate Tracker')
- 获取鼠标坐标
Pygame提供了pygame.mouse.get_pos()
方法,可以用来获取鼠标的当前位置。
running = True
while running:
for event in pygame.event.get():
if event.type == QUIT:
running = False
# 获取鼠标坐标
x, y = pygame.mouse.get_pos()
print(f"Mouse position: x={x}, y={y}")
# 填充背景色
screen.fill((255, 255, 255))
# 更新屏幕
pygame.display.flip()
pygame.quit()
三、使用TKINTER模块
Tkinter是Python的标准GUI库,适合快速创建图形用户界面应用。
- 初始化Tkinter环境
Tkinter是Python自带的GUI库,无需额外安装。
import tkinter as tk
创建主窗口
root = tk.Tk()
root.title("Tkinter Coordinate Tracker")
root.geometry("600x600")
- 获取鼠标坐标
我们可以通过绑定鼠标事件来获取并打印鼠标的坐标。
def print_mouse_position(event):
x, y = event.x, event.y
print(f"Mouse position: x={x}, y={y}")
绑定鼠标移动事件
root.bind('<Motion>', print_mouse_position)
运行主循环
root.mainloop()
总结:
- Turtle模块适合简单的图形绘制和教育目的。
- Pygame模块提供了更强大的图形和事件处理功能,适合开发游戏和复杂的图形应用。
- Tkinter模块适合快速创建图形用户界面应用。
通过以上模块,你可以轻松实现鼠标或画笔坐标的获取和打印,并应用于不同的Python应用程序中。
相关问答FAQs:
如何在Python中获取并打印画笔的坐标?
在Python中,您可以使用图形库(如Tkinter、Pygame或Matplotlib)来创建画笔功能并获取其坐标。以Tkinter为例,您可以通过绑定鼠标事件来获取坐标并在画布上显示。以下是一个简单的示例代码:
import tkinter as tk
def get_coordinates(event):
print(f"当前坐标: ({event.x}, {event.y})")
root = tk.Tk()
canvas = tk.Canvas(root, width=400, height=400)
canvas.pack()
canvas.bind("<Button-1>", get_coordinates) # 绑定左键点击事件
root.mainloop()
在Python中如何绘制图形并显示坐标信息?
要在Python中绘制图形并显示坐标,您可以使用Matplotlib库,结合鼠标事件来获取坐标。例如,您可以在绘图时使用ginput()
函数来获取用户点击的点并打印坐标:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6]) # 绘制简单图形
plt.title('点击获取坐标')
points = plt.ginput(n=-1) # n=-1表示无限次点击
for point in points:
print(f"点击坐标: {point}")
plt.show()
使用Pygame时,如何捕获并打印画笔的移动坐标?
在Pygame中,您可以通过捕获鼠标移动事件来实现。这可以帮助您实时获取画笔的坐标并在窗口中显示。以下是一个基本示例:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400, 400))
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if event.type == pygame.MOUSEMOTION:
print(f"画笔坐标: {event.pos}")
screen.fill((255, 255, 255)) # 清空屏幕
pygame.display.flip()
通过这些方法,您可以在Python中获取和打印画笔的坐标,满足您的绘图需求。
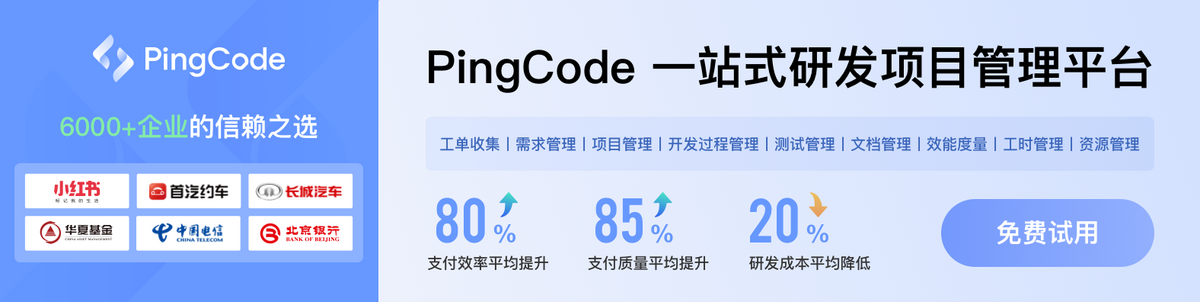