使用Python运行Fortran代码可以通过多种方式实现,主要方法包括使用f2py工具、Cython、以及直接调用Fortran编译生成的共享库。f2py工具是最方便的方法,因为它是NumPy的一部分,可以将Fortran代码直接编译并生成Python模块。
以下是详细介绍这些方法的内容:
一、F2PY工具
f2py是NumPy的一个子模块,专门用于连接Fortran和Python。它可以将Fortran代码直接编译成Python可调用的模块。
1. 使用f2py编译Fortran代码
首先,确保你已经安装了NumPy,因为f2py是其一部分。可以通过以下命令安装NumPy:
pip install numpy
假设你有一个Fortran文件 example.f90
:
subroutine add_arrays(a, b, c, n)
integer, intent(in) :: n
real(8), intent(in) :: a(n), b(n)
real(8), intent(out) :: c(n)
integer :: i
do i = 1, n
c(i) = a(i) + b(i)
end do
end subroutine add_arrays
使用以下命令编译Fortran代码:
f2py -c -m example_module example.f90
这将生成一个名为 example_module
的Python模块。
2. 在Python中调用Fortran代码
编译完成后,可以在Python中使用生成的模块:
import example_module
import numpy as np
a = np.array([1.0, 2.0, 3.0], dtype=np.float64)
b = np.array([4.0, 5.0, 6.0], dtype=np.float64)
c = np.zeros(3, dtype=np.float64)
example_module.add_arrays(a, b, c, len(a))
print(c)
通过这种方式,Python可以直接调用Fortran编写的子程序,将复杂的数值计算交给Fortran处理。
二、CYTHON
Cython是一种将Python代码转换为C/C++代码的工具,它也可以用来连接Python和Fortran代码。
1. 使用Cython编写接口
首先,需要创建一个Cython文件,比如 example.pyx
,用于定义Fortran函数接口:
cdef extern from "example.h":
void add_arrays(double* a, double* b, double* c, int* n)
def py_add_arrays(double[:] a, double[:] b):
cdef int n = a.shape[0]
cdef double[::1] c = np.zeros(n, dtype=np.float64)
add_arrays(&a[0], &b[0], &c[0], &n)
return np.array(c)
2. 编写setup.py进行编译
然后,编写一个 setup.py
文件来编译Cython代码:
from distutils.core import setup
from Cython.Build import cythonize
setup(
ext_modules = cythonize("example.pyx")
)
通过以下命令编译:
python setup.py build_ext --inplace
3. 在Python中调用
编译完成后,可以在Python中调用:
import example
import numpy as np
a = np.array([1.0, 2.0, 3.0], dtype=np.float64)
b = np.array([4.0, 5.0, 6.0], dtype=np.float64)
c = example.py_add_arrays(a, b)
print(c)
Cython提供了一种灵活的方法来连接Fortran和Python,但需要编写Cython接口代码。
三、直接调用共享库
如果你已经有一个编译好的Fortran共享库,可以直接使用Python的 ctypes
或 cffi
模块来调用。
1. 使用ctypes
首先,确保你的Fortran代码已经编译成共享库(例如 example.so
)。
在Python中,可以使用 ctypes
调用:
import ctypes
import numpy as np
加载共享库
lib = ctypes.CDLL('./example.so')
定义函数接口
add_arrays = lib.add_arrays
add_arrays.argtypes = [
np.ctypeslib.ndpointer(dtype=np.float64, ndim=1, flags='C_CONTIGUOUS'),
np.ctypeslib.ndpointer(dtype=np.float64, ndim=1, flags='C_CONTIGUOUS'),
np.ctypeslib.ndpointer(dtype=np.float64, ndim=1, flags='C_CONTIGUOUS'),
ctypes.POINTER(ctypes.c_int)
]
add_arrays.restype = None
使用函数
a = np.array([1.0, 2.0, 3.0], dtype=np.float64)
b = np.array([4.0, 5.0, 6.0], dtype=np.float64)
c = np.zeros(3, dtype=np.float64)
n = ctypes.c_int(len(a))
add_arrays(a, b, c, ctypes.byref(n))
print(c)
这种方法适合于已经有共享库的情况,方便直接调用。
四、总结
使用Python运行Fortran代码有多种方法选择,f2py 是最简单直接的方式,适合快速集成和调用Fortran代码。Cython 适合需要更多定制化接口或者需要同时结合C/C++代码的情况。而 ctypes 则适合于已编译的共享库,可以直接调用库中的函数。根据实际需求和开发环境,可以选择最适合的方法来整合Python和Fortran代码。
相关问答FAQs:
如何将Fortran代码与Python结合使用?
将Fortran代码与Python结合使用,可以通过多种方式实现。最常见的方法是使用f2py
,这是NumPy的一部分,能够将Fortran代码编译并作为Python模块导入。首先,确保你已经安装了NumPy和Fortran编译器。通过f2py
命令将Fortran源代码编译成共享库,然后在Python中导入这个模块。这样,你就可以在Python脚本中调用Fortran函数,从而充分利用Fortran在数值计算方面的高效性。
在Python中调用Fortran程序需要哪些工具或库?
要在Python中调用Fortran程序,通常需要安装f2py
,它是NumPy的一部分。此外,确保你的系统上安装了适合的Fortran编译器(如gfortran)。如果需要更复杂的接口,可以考虑使用Cython
或SWIG
,这些工具可以帮助你将Fortran代码封装成Python可调用的模块。通过这些工具,可以实现更灵活和高效的集成。
使用Python调用Fortran代码的性能如何?
Python与Fortran结合使用时,性能通常会显著提升,特别是在涉及大量数值计算的任务中。Fortran在处理数组和矩阵运算时表现出色,因此如果你的项目中包含复杂的数值计算,使用Fortran进行计算,Python则用于数据处理和可视化,将会是一个高效的方案。不过,性能的提升也取决于如何设计接口和数据传递的方式,因此在实现时需要注意数据格式的兼容性。
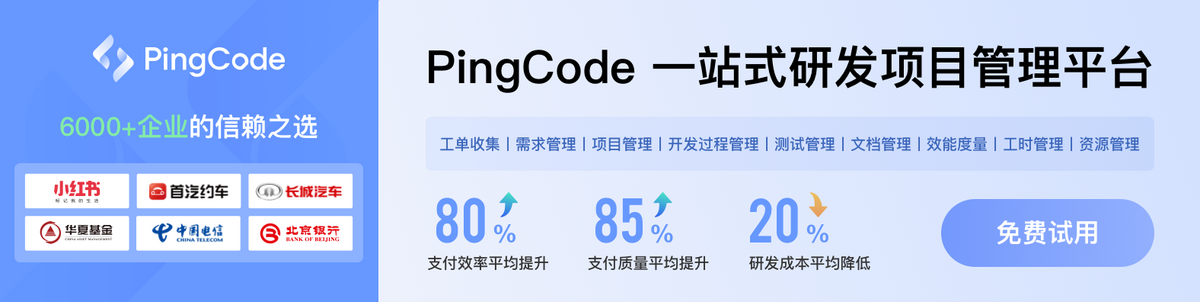