在Python中实现多线程运行可以通过使用threading
模块、利用concurrent.futures
模块、避免全局解释器锁(GIL)限制等方式来实现。多线程在Python中虽然因为GIL的存在而有些局限,但在I/O密集型任务中仍然可以显著提高性能。使用threading
模块是最经典的方法之一,它提供了创建和管理线程的简单接口。通过创建一个或多个线程对象,可以使不同的任务并行执行。下面将详细介绍如何使用这些方法实现多线程运行。
一、使用threading
模块
Python的threading
模块提供了创建和管理线程的简单接口。通过这个模块,你可以轻松地在程序中并发地运行多个线程。下面是使用threading
模块进行多线程编程的详细说明。
1.1 创建线程
在threading
模块中,你可以通过创建Thread
类的实例来启动一个新线程。Thread
类的构造函数接受一个目标函数,该函数将在新线程中执行。
import threading
def worker():
"""线程要执行的函数"""
print("Thread is running")
创建一个线程对象
thread = threading.Thread(target=worker)
启动线程
thread.start()
等待线程完成
thread.join()
在这个例子中,我们首先定义了一个名为worker
的函数,它将在新线程中执行。然后,我们创建了一个Thread
对象,并将worker
函数传递给它作为目标函数。接着,通过调用start
方法启动线程。最后,使用join
方法等待线程完成。
1.2 自定义线程类
除了使用目标函数外,你还可以通过继承Thread
类来创建自定义线程类。这种方法允许你更好地组织代码,并在线程中包含更多的逻辑。
import threading
class MyThread(threading.Thread):
def run(self):
"""重写run方法来定义线程的行为"""
print("Custom thread is running")
创建自定义线程对象
thread = MyThread()
启动线程
thread.start()
等待线程完成
thread.join()
在这个例子中,我们定义了一个名为MyThread
的类,继承了Thread
类,并重写了run
方法。run
方法定义了线程的行为。然后,我们创建MyThread
的实例,并调用start
和join
方法。
1.3 线程同步
当多个线程并发访问共享资源时,可能会导致数据竞争和不一致的问题。为了解决这个问题,threading
模块提供了多种同步机制,如锁(Lock)、条件变量(Condition)、事件(Event)等。
使用锁进行同步
锁是最常用的同步机制之一。它允许线程在访问共享资源时获取一个锁,从而确保同一时间只有一个线程能够访问该资源。
import threading
定义一个全局变量
counter = 0
创建一个锁对象
lock = threading.Lock()
def increment():
global counter
for _ in range(1000):
# 获取锁
lock.acquire()
try:
counter += 1
finally:
# 释放锁
lock.release()
创建两个线程
thread1 = threading.Thread(target=increment)
thread2 = threading.Thread(target=increment)
启动线程
thread1.start()
thread2.start()
等待线程完成
thread1.join()
thread2.join()
print(f"Counter: {counter}")
在这个例子中,我们定义了一个全局变量counter
,并使用一个锁对象lock
来确保counter
的操作是线程安全的。在increment
函数中,线程在修改counter
之前获取锁,并在完成后释放锁。这样可以防止多个线程同时修改counter
导致的竞态条件。
1.4 使用条件变量
条件变量允许线程等待某个条件变为真时再继续执行。这在需要线程间协调时非常有用。
import threading
创建一个条件变量
condition = threading.Condition()
def worker():
with condition:
print("Worker is waiting")
condition.wait()
print("Worker is resuming")
def notifier():
with condition:
print("Notifier is notifying")
condition.notify_all()
创建线程
worker_thread = threading.Thread(target=worker)
notifier_thread = threading.Thread(target=notifier)
启动线程
worker_thread.start()
notifier_thread.start()
等待线程完成
worker_thread.join()
notifier_thread.join()
在这个例子中,我们创建了一个条件变量condition
。worker
函数在条件变量上等待,直到被通知。notifier
函数在条件变量上通知所有等待的线程。这种机制允许线程在需要时等待条件的变化。
二、利用concurrent.futures
模块
concurrent.futures
模块是在Python 3.2中引入的一个高级并发模块,它提供了一个更加高级的接口来管理线程和进程池。这个模块使得多线程编程更加简单和直观。下面我们将详细介绍如何使用concurrent.futures
模块来实现多线程。
2.1 使用ThreadPoolExecutor
ThreadPoolExecutor
是concurrent.futures
模块中用于管理线程池的类。它允许你轻松地并发执行多个任务,而不必手动管理每个线程。
from concurrent.futures import ThreadPoolExecutor
def task(n):
print(f"Task {n} is running")
return n
创建一个线程池,最多同时执行3个线程
with ThreadPoolExecutor(max_workers=3) as executor:
# 提交任务给线程池
futures = [executor.submit(task, i) for i in range(5)]
# 获取任务结果
for future in futures:
result = future.result()
print(f"Task {result} is completed")
在这个例子中,我们创建了一个ThreadPoolExecutor
对象,指定最大同时执行的线程数为3。然后,我们使用submit
方法将任务提交给线程池,submit
方法返回一个Future
对象,代表待完成的任务。最后,我们通过调用future.result()
来获取任务的结果。
2.2 使用as_completed
as_completed
方法可以用于迭代已完成的Future
对象,这在需要处理任务结果的顺序时非常有用。
from concurrent.futures import ThreadPoolExecutor, as_completed
def task(n):
print(f"Task {n} is running")
return n
创建一个线程池
with ThreadPoolExecutor(max_workers=3) as executor:
# 提交任务给线程池
futures = [executor.submit(task, i) for i in range(5)]
# 迭代已完成的任务
for future in as_completed(futures):
result = future.result()
print(f"Task {result} is completed")
在这个例子中,我们使用as_completed
方法迭代已完成的Future
对象。这样可以确保任务结果按完成顺序处理,而不是按提交顺序处理。
2.3 使用map
map
方法可以用于将一个函数应用于一个可迭代对象的每个元素,并返回结果。它类似于内置的map
函数,但支持并发执行。
from concurrent.futures import ThreadPoolExecutor
def task(n):
print(f"Task {n} is running")
return n
创建一个线程池
with ThreadPoolExecutor(max_workers=3) as executor:
# 使用map方法执行任务
results = list(executor.map(task, range(5)))
# 处理结果
for result in results:
print(f"Task {result} is completed")
在这个例子中,我们使用map
方法将task
函数应用于range(5)
的每个元素。map
方法返回一个生成器,我们将其转换为列表并迭代处理结果。
三、避免全局解释器锁(GIL)限制
Python的GIL是一个全局锁,用于管理对Python对象的访问。虽然GIL保证了Python解释器的线程安全性,但它也限制了多线程程序的性能。在CPU密集型任务中,GIL可能会成为瓶颈。因此,在这种情况下,通常建议使用多进程而不是多线程。
3.1 使用multiprocessing
模块
multiprocessing
模块提供了一个类似于threading
模块的接口,但使用的是进程而不是线程。由于每个进程都有自己的Python解释器实例,所以它们不受GIL的限制。
from multiprocessing import Process
def task(n):
print(f"Process {n} is running")
创建多个进程
processes = [Process(target=task, args=(i,)) for i in range(5)]
启动进程
for process in processes:
process.start()
等待进程完成
for process in processes:
process.join()
在这个例子中,我们使用multiprocessing
模块创建了多个进程,每个进程都执行task
函数。通过调用start
方法启动进程,使用join
方法等待进程完成。
3.2 使用ProcessPoolExecutor
concurrent.futures
模块还提供了ProcessPoolExecutor
类,用于管理进程池。它的用法与ThreadPoolExecutor
类似,但使用的是进程而不是线程。
from concurrent.futures import ProcessPoolExecutor
def task(n):
print(f"Task {n} is running")
return n
创建一个进程池
with ProcessPoolExecutor(max_workers=3) as executor:
# 提交任务给进程池
futures = [executor.submit(task, i) for i in range(5)]
# 获取任务结果
for future in futures:
result = future.result()
print(f"Task {result} is completed")
在这个例子中,我们使用ProcessPoolExecutor
创建了一个进程池,并提交任务给进程池。这样可以有效地避免GIL的限制,提高CPU密集型任务的性能。
四、线程安全与数据共享
在多线程编程中,线程安全和数据共享是两个需要特别注意的方面。通过适当的同步机制,确保线程安全和数据一致性。
4.1 使用队列进行数据共享
队列(Queue)是线程安全的数据结构,适用于在线程间共享数据。Python的queue
模块提供了多种类型的队列,包括FIFO队列、LIFO队列和优先级队列。
import threading
import queue
创建一个FIFO队列
data_queue = queue.Queue()
def producer():
for i in range(5):
data_queue.put(i)
print(f"Produced: {i}")
def consumer():
while True:
item = data_queue.get()
print(f"Consumed: {item}")
data_queue.task_done()
创建线程
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
启动线程
producer_thread.start()
consumer_thread.start()
等待队列处理完成
data_queue.join()
在这个例子中,我们使用queue.Queue
创建了一个FIFO队列,并在生产者线程中添加数据,在消费者线程中消费数据。队列的线程安全性确保了数据的正确传递。
4.2 使用事件进行线程间通信
事件(Event)是另一种线程间通信机制,允许线程在某个事件发生时被通知。
import threading
创建一个事件对象
event = threading.Event()
def worker():
print("Worker is waiting for the event")
event.wait()
print("Worker is resuming")
def notifier():
print("Notifier is setting the event")
event.set()
创建线程
worker_thread = threading.Thread(target=worker)
notifier_thread = threading.Thread(target=notifier)
启动线程
worker_thread.start()
notifier_thread.start()
等待线程完成
worker_thread.join()
notifier_thread.join()
在这个例子中,我们创建了一个事件对象event
。worker
线程在事件上等待,直到事件被设置。notifier
线程设置事件,通知所有等待的线程继续执行。
五、实际应用场景
多线程编程在许多实际应用场景中都能发挥作用,特别是在I/O密集型任务中,如网络请求、文件读写和数据库访问。
5.1 网络请求
在处理大量网络请求时,多线程可以显著提高效率,因为网络请求通常是I/O密集型任务。
import threading
import requests
def fetch_url(url):
response = requests.get(url)
print(f"Fetched {url} with status code: {response.status_code}")
urls = ["http://example.com"] * 5
创建多个线程
threads = [threading.Thread(target=fetch_url, args=(url,)) for url in urls]
启动线程
for thread in threads:
thread.start()
等待线程完成
for thread in threads:
thread.join()
在这个例子中,我们使用多线程并发地请求多个URL,提高了网络请求的效率。
5.2 文件读写
在处理大文件或多个文件时,多线程可以加速读写操作。
import threading
def write_file(filename, content):
with open(filename, 'w') as f:
f.write(content)
print(f"Written to {filename}")
files = [("file1.txt", "Hello, World!"), ("file2.txt", "Python is fun!")]
创建多个线程
threads = [threading.Thread(target=write_file, args=(filename, content)) for filename, content in files]
启动线程
for thread in threads:
thread.start()
等待线程完成
for thread in threads:
thread.join()
在这个例子中,我们使用多线程并发地写入多个文件,提高了文件写入的效率。
5.3 数据库访问
在处理多个数据库查询时,多线程可以减少等待时间,提高数据库访问的效率。
import threading
import sqlite3
def query_db(query):
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute(query)
result = cursor.fetchall()
print(f"Query result: {result}")
conn.close()
queries = ["SELECT * FROM table1", "SELECT * FROM table2"]
创建多个线程
threads = [threading.Thread(target=query_db, args=(query,)) for query in queries]
启动线程
for thread in threads:
thread.start()
等待线程完成
for thread in threads:
thread.join()
在这个例子中,我们使用多线程并发地执行多个数据库查询,减少了查询的等待时间。
总结
多线程编程在Python中有广泛的应用场景,特别是在I/O密集型任务中。通过使用threading
模块、concurrent.futures
模块和multiprocessing
模块,你可以轻松地实现多线程和多进程编程。然而,需要注意的是,Python的GIL限制了CPU密集型任务的多线程性能。在这种情况下,使用多进程可能是更好的选择。无论是线程还是进程,确保线程安全和数据一致性都是至关重要的。通过适当的同步机制,如锁、条件变量和事件,可以有效地解决这些问题。在实际应用中,结合使用多线程和多进程技术,可以显著提高程序的性能和效率。
相关问答FAQs:
如何使用Python实现多线程?
在Python中,多线程可以通过threading
模块来实现。首先,您需要导入该模块,然后创建一个线程对象,并定义一个函数来执行线程所需的操作。使用start()
方法可以启动线程,使用join()
方法可以等待线程完成。例如,您可以创建一个简单的线程来执行打印操作,或者进行数据处理等任务。
多线程与多进程有什么区别?
多线程和多进程的主要区别在于它们的执行方式和资源使用。多线程在同一进程内运行共享内存,适合I/O密集型任务,比如网络请求或文件操作。而多进程则创建多个独立的进程,适合CPU密集型任务,因为每个进程都有自己的内存空间,互不干扰。在Python中,由于全局解释器锁(GIL)的存在,CPU密集型任务的多线程效率可能不如多进程。
在Python中如何处理线程安全问题?
在多线程编程中,线程安全是一个重要问题。为了确保线程安全,可以使用Lock
对象来控制对共享资源的访问。通过在访问共享资源的代码块前后使用acquire()
和release()
方法,可以避免数据竞争和不一致的情况。此外,Python的queue
模块也提供了线程安全的队列实现,适合在线程之间安全地传递数据。
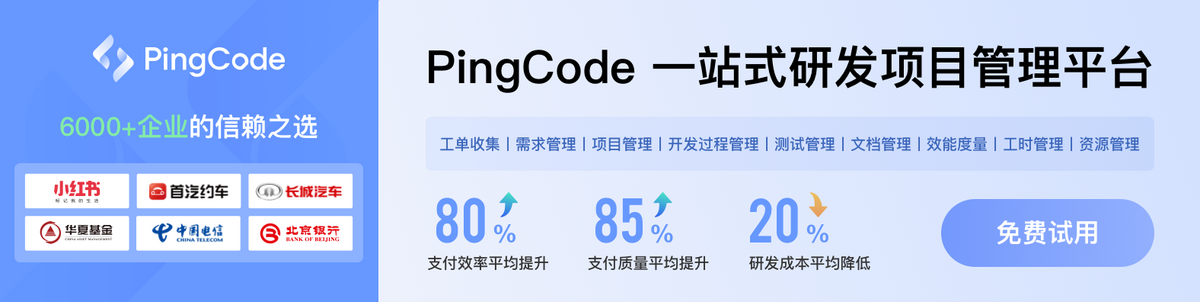