Python获取资源的方法多种多样,通常可以通过网络请求、文件操作、数据库连接、API调用、网络爬虫等手段获取。其中,API调用和网络爬虫是比较常用的方式。API调用通常用于获取结构化数据,例如使用RESTful API访问网络服务;而网络爬虫则用于从网页中提取信息。下面将详细介绍Python中获取资源的多种方法,并结合实例说明如何使用这些技术手段。
一、通过API调用获取资源
API(应用程序接口)提供了一种访问网络服务的标准方式。许多服务提供商,如Twitter、Facebook和Google,都提供API以便开发者可以轻松访问其服务中的数据。
1.1 使用requests库调用API
Python的requests
库是一个简单而强大的HTTP库,非常适合用于API调用。通过它,我们可以发送HTTP请求并处理响应。
import requests
def fetch_data_from_api(url, params=None):
response = requests.get(url, params=params)
if response.status_code == 200:
return response.json()
else:
response.raise_for_status()
示例:调用GitHub API获取用户信息
url = "https://api.github.com/users/octocat"
user_info = fetch_data_from_api(url)
print(user_info)
在这个示例中,我们使用requests.get
方法发送GET请求,并使用response.json()
方法将响应转换为Python字典。
1.2 处理API响应数据
API响应的数据通常是JSON格式。Python内置了json
库,可以很方便地解析和处理JSON数据。
import json
def process_json_data(json_data):
# 解析JSON数据
data_dict = json.loads(json_data)
# 处理数据
for key, value in data_dict.items():
print(f"{key}: {value}")
示例:处理API响应数据
json_data = '{"name": "octocat", "blog": "https://github.blog"}'
process_json_data(json_data)
通过json.loads
方法,我们可以将JSON字符串解析为Python字典,然后可以对其进行遍历和操作。
二、通过网络爬虫获取资源
网络爬虫是一种自动访问互联网并提取信息的技术。Python中有许多库可以帮助我们实现网络爬虫,如BeautifulSoup
、Scrapy
和Selenium
。
2.1 使用BeautifulSoup解析网页
BeautifulSoup
是一个用于解析HTML和XML文档的Python库。它提供了一种方便的方式来遍历文档树,并提取需要的信息。
from bs4 import BeautifulSoup
import requests
def fetch_webpage_content(url):
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
response.raise_for_status()
def parse_html_content(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
# 提取标题
title = soup.title.string
print(f"Page Title: {title}")
# 提取所有链接
for link in soup.find_all('a'):
print(link.get('href'))
示例:解析网页内容
url = "https://www.python.org/"
html_content = fetch_webpage_content(url)
parse_html_content(html_content)
在这个示例中,我们首先获取网页的HTML内容,然后使用BeautifulSoup
解析HTML文档。通过soup.title.string
可以获取网页的标题,通过soup.find_all('a')
可以获取所有链接。
2.2 使用Scrapy进行高级爬虫
Scrapy
是一个强大的爬虫框架,适合于构建复杂的爬虫和数据提取项目。它提供了许多功能,如请求调度、数据提取和存储等。
import scrapy
class QuotesSpider(scrapy.Spider):
name = "quotes"
start_urls = ['http://quotes.toscrape.com/']
def parse(self, response):
for quote in response.css('div.quote'):
yield {
'text': quote.css('span.text::text').get(),
'author': quote.css('small.author::text').get(),
'tags': quote.css('div.tags a.tag::text').getall(),
}
示例:运行Scrapy爬虫
需要在命令行中运行:scrapy runspider quotes_spider.py
在这个示例中,我们定义了一个名为QuotesSpider
的爬虫类。通过定义start_urls
和parse
方法,我们可以指定爬虫的起始网址和数据提取逻辑。
三、通过文件操作获取资源
Python提供了强大的文件操作功能,可以读取和写入本地文件。这种方式常用于处理本地数据文件,如文本文件、CSV文件和JSON文件。
3.1 读取和写入文本文件
Python内置的open
函数可以用于打开文件,并提供文件读取和写入的功能。
def read_text_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
def write_text_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
示例:读取和写入文本文件
file_path = 'example.txt'
write_text_file(file_path, 'Hello, World!')
content = read_text_file(file_path)
print(content)
在这个示例中,我们使用open
函数以读模式和写模式打开文件,并使用with
语句确保文件自动关闭。
3.2 处理CSV文件
CSV(逗号分隔值)文件是一种常见的数据交换格式。Python的csv
库提供了读取和写入CSV文件的功能。
import csv
def read_csv_file(file_path):
with open(file_path, newline='') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(', '.join(row))
def write_csv_file(file_path, data):
with open(file_path, 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerows(data)
示例:读取和写入CSV文件
file_path = 'example.csv'
data = [['Name', 'Age'], ['Alice', '30'], ['Bob', '25']]
write_csv_file(file_path, data)
read_csv_file(file_path)
在这个示例中,我们使用csv.reader
读取CSV文件,并使用csv.writer
写入CSV文件。
四、通过数据库连接获取资源
数据库是存储和管理数据的常用方式。Python支持与多种数据库的连接,包括SQLite、MySQL、PostgreSQL等。
4.1 使用SQLite数据库
SQLite是一个轻量级的嵌入式数据库,Python标准库中自带了sqlite3
模块。
import sqlite3
def create_database(db_path):
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT, age INTEGER)''')
conn.commit()
conn.close()
def insert_user(db_path, name, age):
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute('INSERT INTO users (name, age) VALUES (?, ?)', (name, age))
conn.commit()
conn.close()
def fetch_users(db_path):
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute('SELECT * FROM users')
users = cursor.fetchall()
conn.close()
return users
示例:操作SQLite数据库
db_path = 'example.db'
create_database(db_path)
insert_user(db_path, 'Alice', 30)
insert_user(db_path, 'Bob', 25)
users = fetch_users(db_path)
print(users)
在这个示例中,我们使用sqlite3.connect
连接到数据库,并使用SQL语句创建表、插入数据和查询数据。
4.2 使用MySQL数据库
MySQL是一个流行的关系型数据库管理系统。Python中可以使用pymysql
库连接到MySQL数据库。
import pymysql
def connect_to_mysql(host, user, password, db):
connection = pymysql.connect(host=host, user=user, password=password, database=db)
return connection
def fetch_data_from_mysql(connection):
with connection.cursor() as cursor:
cursor.execute("SELECT * FROM users")
result = cursor.fetchall()
return result
示例:连接到MySQL数据库并获取数据
host = 'localhost'
user = 'root'
password = 'password'
db = 'test_db'
connection = connect_to_mysql(host, user, password, db)
users = fetch_data_from_mysql(connection)
print(users)
connection.close()
在这个示例中,我们使用pymysql.connect
连接到MySQL数据库,并使用SQL语句查询数据。
五、通过云服务获取资源
云服务提供了强大的计算和存储能力,Python可以通过各种SDK(软件开发工具包)与云服务进行交互,如AWS SDK、Google Cloud SDK和Azure SDK。
5.1 使用AWS SDK(Boto3)
Boto3是AWS SDK for Python,它可以用于与AWS服务进行交互。
import boto3
def list_s3_buckets():
s3 = boto3.client('s3')
response = s3.list_buckets()
return [bucket['Name'] for bucket in response['Buckets']]
示例:列出S3桶
buckets = list_s3_buckets()
print(buckets)
在这个示例中,我们使用Boto3创建一个S3客户端,并调用list_buckets
方法列出所有S3桶。
5.2 使用Google Cloud SDK
Google Cloud SDK提供了与Google Cloud服务交互的功能。Python可以通过google-cloud-storage
库访问Google Cloud Storage。
from google.cloud import storage
def list_gcs_buckets():
client = storage.Client()
buckets = client.list_buckets()
return [bucket.name for bucket in buckets]
示例:列出GCS桶
buckets = list_gcs_buckets()
print(buckets)
在这个示例中,我们创建了一个Google Cloud Storage客户端,并调用list_buckets
方法列出所有GCS桶。
六、通过第三方库获取资源
Python有丰富的第三方库,可以用于获取各种类型的资源,如图像、视频、音频等。
6.1 使用Pillow处理图像
Pillow是一个强大的图像处理库,可以用于打开、操作和保存图像文件。
from PIL import Image
def open_image(file_path):
with Image.open(file_path) as img:
img.show()
def save_image(img, file_path):
img.save(file_path)
示例:打开和保存图像
file_path = 'example.jpg'
img = Image.open(file_path)
save_image(img, 'output.jpg')
在这个示例中,我们使用Pillow打开图像文件,并将其显示和保存。
6.2 使用OpenCV处理视频
OpenCV是一个流行的计算机视觉库,可以用于处理视频和图像。
import cv2
def play_video(file_path):
cap = cv2.VideoCapture(file_path)
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
cv2.imshow('Video', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
示例:播放视频
file_path = 'example.mp4'
play_video(file_path)
在这个示例中,我们使用OpenCV打开和播放视频文件。
七、通过调试和优化获取资源
在获取资源的过程中,调试和优化是不可或缺的步骤。Python提供了许多工具和技巧,可以帮助我们提高代码的性能和可靠性。
7.1 使用logging调试代码
logging
模块提供了灵活的日志记录功能,可以帮助我们调试和跟踪程序的执行。
import logging
logging.basicConfig(level=logging.INFO)
def fetch_data():
logging.info('Fetching data...')
# 模拟获取数据过程
data = {'key': 'value'}
logging.info('Data fetched: %s', data)
return data
示例:使用logging调试代码
data = fetch_data()
在这个示例中,我们使用logging.info
方法记录信息,这些日志可以帮助我们了解程序的执行流程。
7.2 使用profiling优化性能
cProfile
模块可以用于分析Python程序的性能,帮助我们找出程序中的瓶颈。
import cProfile
def compute():
total = 0
for i in range(10000):
total += i
return total
示例:使用cProfile分析性能
cProfile.run('compute()')
在这个示例中,我们使用cProfile.run
方法分析compute
函数的性能,输出结果中包含了函数调用的次数和时间。
总结
通过以上方法,Python可以高效地获取各种类型的资源。无论是通过API调用、网络爬虫、文件操作、数据库连接,还是通过云服务和第三方库,Python都提供了丰富的工具和库来满足不同的需求。同时,调试和优化也是获取资源过程中不可或缺的部分,可以帮助我们提高代码的性能和可靠性。在实际应用中,根据具体的需求和场景选择合适的方法和工具,是成功获取资源的关键。
相关问答FAQs:
如何使用Python从网络获取数据?
Python提供了多种库来帮助用户从网络获取数据,例如Requests和BeautifulSoup。通过Requests库,您可以发送HTTP请求并获取网页内容,而BeautifulSoup则可以解析HTML文档,提取您所需的特定信息。这种组合非常适合进行网页抓取和数据分析。
Python中有哪些库可以帮助获取API数据?
在Python中,使用Requests库是获取API数据的常用方法。通过发送GET或POST请求,您可以轻松获取API提供的JSON或XML格式的数据。此外,像Pandas库也可以帮助您处理和分析API返回的数据,使数据分析变得更加高效。
如何在Python中处理和存储获取的资源?
获取的资源可以使用Python的多种数据结构进行存储,例如列表、字典或Pandas DataFrame。如果您需要将数据持久化,您可以使用SQLite、CSV或JSON格式进行存储。这样,您可以在后续分析或处理过程中方便地访问和使用这些数据。
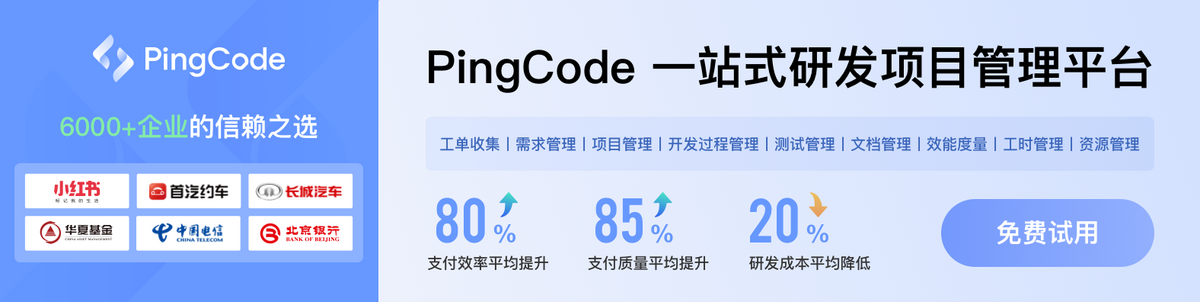