要用Python打开WordPress,有几种方法:使用WordPress REST API、通过WordPress XML-RPC API、直接解析WordPress数据库。其中,使用WordPress REST API是最为现代和推荐的方法,因为它提供了简化的接口和更好的安全性。REST API允许你通过HTTP请求与WordPress进行交互,获取或修改数据。首先,你需要确保WordPress站点启用了REST API,然后通过Python的requests
库发送请求,获取数据或执行操作。
一、使用WORDPRESS REST API
WordPress REST API是一个强大的工具,允许开发者通过HTTP请求与WordPress进行交互。通过REST API,Python开发者可以轻松获取WordPress站点的数据,或对其进行操作。
1. 安装和配置
在使用WordPress REST API之前,确保你的WordPress站点启用了REST API。默认情况下,从WordPress 4.7版本开始,REST API已经内置并自动启用。为了确保API正常工作,检查网站的permalink设置,并设置为非默认结构。
安装Python的requests
库以便于发送HTTP请求:
pip install requests
2. 基础请求操作
使用requests
库,可以获取WordPress站点的信息。以下是一个简单的例子,演示如何获取最新的文章:
import requests
url = "https://example.com/wp-json/wp/v2/posts"
response = requests.get(url)
if response.status_code == 200:
posts = response.json()
for post in posts:
print(f"Title: {post['title']['rendered']}")
print(f"Content: {post['content']['rendered']}")
else:
print("Failed to retrieve posts")
在这个例子中,我们发送了一个GET请求到WordPress REST API的/wp/v2/posts
端点,并解析了返回的JSON数据。
3. 认证与授权
对于需要认证的操作,如创建、更新或删除文章,需要使用OAuth、Application Passwords或JWT进行认证。以下是使用Application Passwords进行认证的例子:
from requests.auth import HTTPBasicAuth
url = "https://example.com/wp-json/wp/v2/posts"
username = "your_username"
password = "your_application_password"
response = requests.get(url, auth=HTTPBasicAuth(username, password))
if response.status_code == 200:
posts = response.json()
# 处理获取的文章
else:
print("Failed to retrieve posts")
4. 创建和更新文章
创建新文章需要发送POST请求,并提供必要的数据。以下是一个创建文章的示例:
post_data = {
"title": "New Post Title",
"content": "This is the content of the new post.",
"status": "publish"
}
response = requests.post(url, json=post_data, auth=HTTPBasicAuth(username, password))
if response.status_code == 201:
print("Post created successfully")
else:
print("Failed to create post")
更新文章类似于创建文章,只需发送PUT请求到特定文章的端点。
二、通过WORDPRESS XML-RPC API
XML-RPC API是WordPress较早提供的接口方法。虽然REST API是现代化的选择,但XML-RPC仍然被很多工具和插件使用。
1. 启用XML-RPC
默认情况下,WordPress的XML-RPC接口是启用的。可以通过访问https://example.com/xmlrpc.php
来确认。如果返回的是"XML-RPC server accepts POST requests only.",则表示接口正常启用。
2. 使用Python进行请求
Python有一个xmlrpc.client
库,可以方便地与XML-RPC接口进行交互。
import xmlrpc.client
url = "https://example.com/xmlrpc.php"
username = "your_username"
password = "your_password"
server = xmlrpc.client.ServerProxy(url)
try:
# 获取最近10篇文章
posts = server.wp.getPosts(0, username, password, {'number': 10})
for post in posts:
print(f"Title: {post['post_title']}")
except xmlrpc.client.Error as err:
print(f"An error occurred: {err}")
三、直接解析WORDPRESS数据库
直接解析WordPress数据库是一种较为复杂的方法,通常不推荐,因为需要直接访问数据库,涉及安全性和数据完整性问题。
1. 数据库连接
使用Python连接到WordPress的MySQL数据库需要安装mysql-connector-python
库:
pip install mysql-connector-python
然后,编写代码连接到数据库:
import mysql.connector
config = {
'user': 'your_db_user',
'password': 'your_db_password',
'host': 'your_db_host',
'database': 'your_db_name'
}
conn = mysql.connector.connect(config)
cursor = conn.cursor()
查询文章
query = "SELECT post_title, post_content FROM wp_posts WHERE post_status='publish' AND post_type='post'"
cursor.execute(query)
for (title, content) in cursor:
print(f"Title: {title}")
print(f"Content: {content}")
cursor.close()
conn.close()
2. 数据库查询和操作
查询WordPress数据库需要了解其数据结构。WordPress的主要数据存储在wp_posts
、wp_postmeta
、wp_users
等表中。要进行更多复杂的查询,可能需要连接多个表并处理数据关系。
四、总结与注意事项
选择合适的方法来与WordPress进行交互取决于具体需求。使用WordPress REST API是最推荐的方式,因为它安全、现代化且易于使用。XML-RPC是较旧的方法,但仍然适用于某些特定场景。直接访问数据库则需要注意安全性和数据完整性问题。
在任何情况下,确保对WordPress站点的操作是经过授权的,并妥善管理认证信息。对于生产环境,避免直接使用明文密码,建议使用环境变量或安全存储工具来管理敏感信息。
相关问答FAQs:
如何使用Python连接到WordPress网站?
要使用Python连接到WordPress网站,可以使用WordPress的REST API。首先,确保你的WordPress网站启用了REST API。然后,可以使用requests库发送HTTP请求进行身份验证和获取数据。示例代码如下:
import requests
url = "https://yourwordpresssite.com/wp-json/wp/v2/posts"
response = requests.get(url)
print(response.json())
这将返回网站上所有文章的JSON数据。
Python可以用来进行哪些WordPress操作?
Python可以用来执行多种WordPress操作,包括但不限于创建、更新和删除文章,管理用户,上传媒体文件等。通过REST API,Python脚本可以与WordPress进行交互,从而实现自动化任务。例如,可以编写脚本定期发布新内容或分析现有文章的表现。
如何处理WordPress的身份验证问题?
进行身份验证时,通常需要使用OAuth或基本认证。对于简单的操作,基本认证是一个不错的选择。可以通过添加Authorization头来实现。使用requests库时,代码示例如下:
from requests.auth import HTTPBasicAuth
response = requests.get(url, auth=HTTPBasicAuth('username', 'password'))
确保将username
和password
替换为你的WordPress凭证。对于更复杂的应用,建议使用OAuth 2.0进行安全认证。
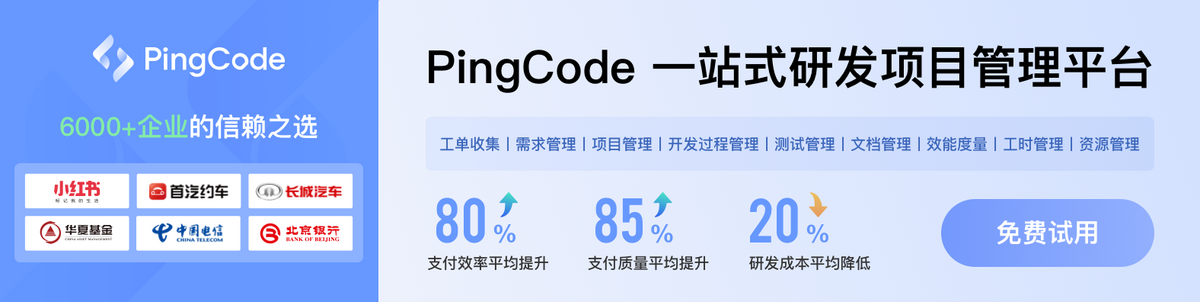