Python实现界面跳转的方法包括:使用Tkinter库创建多个窗口、使用框架如Flask或Django处理网页跳转、使用Kivy库创建复杂的应用界面。下面将详细介绍通过使用Tkinter库实现桌面应用程序中的界面跳转的方法。
一、Tkinter库的介绍
Tkinter是Python的标准GUI库,它是Python包装的Tcl/Tk GUI工具包,提供了构建图形用户界面的基本功能。通过Tkinter,我们可以轻松地创建窗口、按钮、标签等控件,并实现界面跳转等功能。
二、创建基本窗口与控件
在开始实现界面跳转之前,我们需要了解如何使用Tkinter创建基本窗口和控件。以下是一个简单的示例代码,展示了如何创建一个窗口,并在其中添加一个按钮和标签:
import tkinter as tk
def on_button_click():
label.config(text="Hello, Tkinter!")
root = tk.Tk()
root.title("Simple Tkinter Example")
label = tk.Label(root, text="Welcome!")
label.pack()
button = tk.Button(root, text="Click Me", command=on_button_click)
button.pack()
root.mainloop()
上述代码创建了一个简单的窗口,包含一个标签和一个按钮。当按钮被点击时,标签的文本将会更改。
三、实现界面跳转
为了实现界面跳转,我们需要创建多个窗口或帧,并在用户操作时显示相应的窗口或帧。以下是一个示例代码,展示了如何在Tkinter中实现界面跳转:
import tkinter as tk
class Application(tk.Tk):
def __init__(self):
super().__init__()
self.title("Tkinter Page Navigation")
self.container = tk.Frame(self)
self.container.pack(fill="both", expand=True)
self.frames = {}
for F in (StartPage, PageOne, PageTwo):
page_name = F.__name__
frame = F(parent=self.container, controller=self)
self.frames[page_name] = frame
frame.grid(row=0, column=0, sticky="nsew")
self.show_frame("StartPage")
def show_frame(self, page_name):
frame = self.frames[page_name]
frame.tkraise()
class StartPage(tk.Frame):
def __init__(self, parent, controller):
super().__init__(parent)
self.controller = controller
label = tk.Label(self, text="This is the start page")
label.pack(side="top", fill="x", pady=10)
button1 = tk.Button(self, text="Go to Page One",
command=lambda: controller.show_frame("PageOne"))
button1.pack()
button2 = tk.Button(self, text="Go to Page Two",
command=lambda: controller.show_frame("PageTwo"))
button2.pack()
class PageOne(tk.Frame):
def __init__(self, parent, controller):
super().__init__(parent)
self.controller = controller
label = tk.Label(self, text="This is page one")
label.pack(side="top", fill="x", pady=10)
button = tk.Button(self, text="Back to Start Page",
command=lambda: controller.show_frame("StartPage"))
button.pack()
class PageTwo(tk.Frame):
def __init__(self, parent, controller):
super().__init__(parent)
self.controller = controller
label = tk.Label(self, text="This is page two")
label.pack(side="top", fill="x", pady=10)
button = tk.Button(self, text="Back to Start Page",
command=lambda: controller.show_frame("StartPage"))
button.pack()
if __name__ == "__main__":
app = Application()
app.mainloop()
四、详细解释代码实现
-
创建主窗口类
Application
:我们使用继承tk.Tk
创建一个应用程序的主窗口。该类包含一个容器container
,用于放置所有页面帧。 -
创建页面帧
frames
字典:在初始化过程中,我们创建了三个页面帧StartPage
、PageOne
和PageTwo
,并将它们存储在frames
字典中。每个帧都通过grid
方法被放置在容器中。 -
显示页面方法
show_frame
:该方法接收一个页面名称作为参数,并将对应的页面帧置于顶层显示。 -
定义各个页面帧类:我们分别定义了
StartPage
、PageOne
和PageTwo
三个页面帧类。在每个页面中,我们创建了标签和按钮,按钮的命令通过lambda
表达式调用controller.show_frame
方法,切换到指定的页面。
五、实现更复杂的界面跳转
在实际应用中,我们可能需要实现更复杂的界面跳转逻辑,例如根据用户输入或条件判断跳转到不同的页面。以下是一个示例代码,展示了如何根据用户输入实现条件跳转:
import tkinter as tk
from tkinter import messagebox
class Application(tk.Tk):
def __init__(self):
super().__init__()
self.title("Tkinter Conditional Navigation")
self.container = tk.Frame(self)
self.container.pack(fill="both", expand=True)
self.frames = {}
for F in (StartPage, LoginPage, SuccessPage, FailurePage):
page_name = F.__name__
frame = F(parent=self.container, controller=self)
self.frames[page_name] = frame
frame.grid(row=0, column=0, sticky="nsew")
self.show_frame("StartPage")
def show_frame(self, page_name):
frame = self.frames[page_name]
frame.tkraise()
class StartPage(tk.Frame):
def __init__(self, parent, controller):
super().__init__(parent)
self.controller = controller
label = tk.Label(self, text="This is the start page")
label.pack(side="top", fill="x", pady=10)
button = tk.Button(self, text="Go to Login Page",
command=lambda: controller.show_frame("LoginPage"))
button.pack()
class LoginPage(tk.Frame):
def __init__(self, parent, controller):
super().__init__(parent)
self.controller = controller
label = tk.Label(self, text="Login Page")
label.pack(side="top", fill="x", pady=10)
self.username_entry = tk.Entry(self)
self.username_entry.pack()
self.password_entry = tk.Entry(self, show="*")
self.password_entry.pack()
button = tk.Button(self, text="Login", command=self.check_login)
button.pack()
def check_login(self):
username = self.username_entry.get()
password = self.password_entry.get()
if username == "admin" and password == "password":
self.controller.show_frame("SuccessPage")
else:
messagebox.showerror("Error", "Invalid username or password")
self.controller.show_frame("FailurePage")
class SuccessPage(tk.Frame):
def __init__(self, parent, controller):
super().__init__(parent)
self.controller = controller
label = tk.Label(self, text="Login Successful!")
label.pack(side="top", fill="x", pady=10)
button = tk.Button(self, text="Back to Start Page",
command=lambda: controller.show_frame("StartPage"))
button.pack()
class FailurePage(tk.Frame):
def __init__(self, parent, controller):
super().__init__(parent)
self.controller = controller
label = tk.Label(self, text="Login Failed!")
label.pack(side="top", fill="x", pady=10)
button = tk.Button(self, text="Back to Login Page",
command=lambda: controller.show_frame("LoginPage"))
button.pack()
if __name__ == "__main__":
app = Application()
app.mainloop()
六、总结
以上代码展示了如何使用Tkinter库实现界面跳转,包括创建多个页面帧、在不同页面之间进行跳转,以及根据用户输入实现条件跳转。通过这种方法,我们可以轻松地构建具有多页面导航功能的桌面应用程序。
在实际开发中,根据具体需求,还可以进一步扩展和优化代码,例如添加更多的控件、实现复杂的业务逻辑、增强界面美观度等。无论是简单的单页面应用,还是复杂的多页面应用,Tkinter都能为我们提供强大的支持。
相关问答FAQs:
1. 如何在Python中使用tkinter实现界面跳转?
在Python中,tkinter是一个广泛使用的GUI库。要实现界面跳转,可以通过创建多个窗口(或帧)并使用按钮或其他控件来控制显示的界面。可以将不同的界面封装为类,使用pack()
和grid()
方法来管理布局,通过调用destroy()
方法关闭当前界面,再用deiconify()
方法显示目标界面。
2. 使用PyQt实现界面跳转的步骤有哪些?
在使用PyQt时,可以通过创建不同的QWidget或QDialog来实现界面跳转。首先,定义不同的界面类,每个类代表一个窗口。在主窗口中,可以通过信号与槽机制连接按钮点击事件到相应的界面切换逻辑。使用show()
方法显示新窗口,使用close()
方法隐藏当前窗口。
3. Python中的Flask框架如何实现页面跳转?
在Flask框架中,可以通过路由和重定向来实现页面跳转。使用@app.route()
装饰器定义不同的视图函数,并在需要跳转的地方使用redirect()
和url_for()
函数,来实现从一个页面到另一个页面的跳转。这种方式适用于Web应用程序,使得用户在浏览器中能够轻松地导航不同的页面。
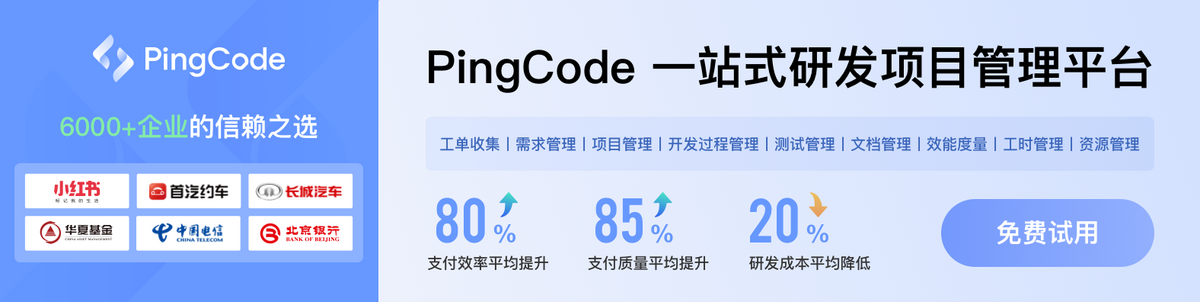