编写Python窗口程序,常用的库包括Tkinter、PyQt5、wxPython。推荐使用Tkinter、PyQt5。
其中,Tkinter是Python的标准GUI库,简单、轻量,适合新手学习;PyQt5功能强大,适合复杂应用。本文将详细介绍如何使用Tkinter和PyQt5编写窗口程序。
一、使用Tkinter编写窗口程序
1、安装Tkinter
Tkinter是Python的标准库,通常已经随Python一起安装。你可以通过以下命令检查是否已安装:
import tkinter
如果没有报错,说明已经安装;否则,可以通过Python的包管理工具pip进行安装:
pip install tk
2、创建基本窗口
首先,我们来创建一个基本的窗口:
import tkinter as tk
创建主窗口
root = tk.Tk()
root.title("Tkinter Window")
root.geometry("400x300")
进入主循环
root.mainloop()
这段代码创建了一个标题为“Tkinter Window”、大小为400×300的窗口。
3、添加组件
我们可以在窗口中添加各种组件,如标签、按钮、输入框等。
import tkinter as tk
root = tk.Tk()
root.title("Tkinter Window")
root.geometry("400x300")
标签
label = tk.Label(root, text="Hello, Tkinter!")
label.pack()
按钮
button = tk.Button(root, text="Click Me", command=lambda: print("Button clicked"))
button.pack()
输入框
entry = tk.Entry(root)
entry.pack()
root.mainloop()
4、布局管理
Tkinter提供了三种布局管理器:pack、grid和place。
- pack:按顺序排列组件。
- grid:类似于表格,按行和列排列组件。
- place:通过绝对坐标来定位组件。
import tkinter as tk
root = tk.Tk()
root.title("Tkinter Window")
root.geometry("400x300")
使用grid布局
label1 = tk.Label(root, text="Label 1")
label1.grid(row=0, column=0)
label2 = tk.Label(root, text="Label 2")
label2.grid(row=1, column=1)
root.mainloop()
5、事件处理
事件处理是指响应用户的操作,如点击按钮、输入文字等。
import tkinter as tk
def on_button_click():
print("Button clicked")
root = tk.Tk()
root.title("Tkinter Window")
root.geometry("400x300")
button = tk.Button(root, text="Click Me", command=on_button_click)
button.pack()
root.mainloop()
二、使用PyQt5编写窗口程序
1、安装PyQt5
可以通过pip安装PyQt5:
pip install PyQt5
2、创建基本窗口
首先,我们来创建一个基本的窗口:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt5 Window")
self.setGeometry(100, 100, 400, 300)
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
这段代码创建了一个标题为“PyQt5 Window”、大小为400×300的窗口。
3、添加组件
我们可以在窗口中添加各种组件,如标签、按钮、输入框等。
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton, QLineEdit
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt5 Window")
self.setGeometry(100, 100, 400, 300)
# 标签
self.label = QLabel("Hello, PyQt5!", self)
self.label.move(50, 50)
# 按钮
self.button = QPushButton("Click Me", self)
self.button.move(50, 100)
self.button.clicked.connect(self.on_button_click)
# 输入框
self.entry = QLineEdit(self)
self.entry.move(50, 150)
def on_button_click(self):
print("Button clicked")
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
4、布局管理
PyQt5提供了多种布局管理器:QVBoxLayout、QHBoxLayout、QGridLayout等。
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QVBoxLayout, QWidget
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt5 Window")
self.setGeometry(100, 100, 400, 300)
layout = QVBoxLayout()
label1 = QLabel("Label 1")
layout.addWidget(label1)
label2 = QLabel("Label 2")
layout.addWidget(label2)
container = QWidget()
container.setLayout(layout)
self.setCentralWidget(container)
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
5、事件处理
事件处理是指响应用户的操作,如点击按钮、输入文字等。
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt5 Window")
self.setGeometry(100, 100, 400, 300)
self.button = QPushButton("Click Me", self)
self.button.move(50, 50)
self.button.clicked.connect(self.on_button_click)
def on_button_click(self):
print("Button clicked")
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
三、总结
通过以上介绍,我们了解了如何使用Tkinter和PyQt5编写窗口程序。Tkinter适合简单的应用,学习成本低,PyQt5适合复杂的应用,功能强大。根据需求选择合适的库,能够帮助我们更好地完成窗口程序的开发。
相关问答FAQs:
如何开始学习Python窗口程序的开发?
学习Python窗口程序的开发可以从几个方面入手。首先,熟悉Python语言的基础语法是必要的。接着,选择一个适合的图形用户界面(GUI)库,例如Tkinter、PyQt或wxPython。Tkinter是Python自带的库,适合初学者使用,而PyQt则功能更为强大,适合开发复杂的应用。可以通过在线教程、书籍或视频课程来深入学习这些库的用法。
在Python中创建窗口程序需要哪些基本组件?
创建一个基本的窗口程序通常需要几个关键组件,包括主窗口、按钮、文本框和标签等。主窗口是应用程序的基础界面,按钮用于触发事件,文本框用于用户输入,标签用于显示信息。了解这些组件的用法和属性,可以帮助你更好地设计和实现自己的窗口应用程序。
如何处理Python窗口程序中的事件和交互?
在Python窗口程序中,事件处理是实现用户交互的核心。每个GUI库都有其特定的事件处理机制,例如Tkinter使用绑定事件的方式来响应用户的操作。可以通过定义回调函数来处理按钮点击、键盘输入等事件。掌握这些事件的处理方法,可以大大提升程序的用户体验,使应用更加灵活和互动。
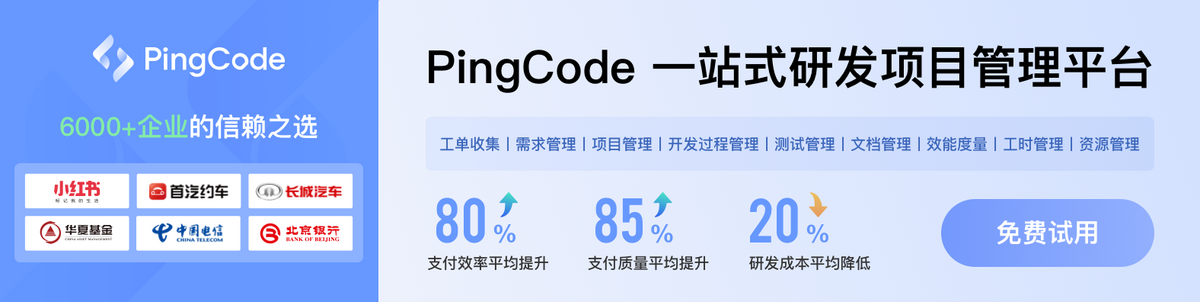