回答标题问题:Python匹配某些字符的方法主要有正则表达式、字符串方法、列表解析等。正则表达式、字符串方法、列表解析是主要方法。正则表达式是最强大和灵活的工具,可以用来处理复杂的匹配和替换任务。下面我们将详细介绍这些方法。
一、正则表达式
正则表达式(Regular Expressions,简称regex)是一个强大而灵活的工具,用于匹配字符串中的模式。Python提供了re
模块来处理正则表达式。以下是一些常见的用法和示例。
1. 导入re
模块
在使用正则表达式之前,首先需要导入Python的re
模块:
import re
2. 基本匹配
正则表达式的基本匹配可以使用re.search()
和re.match()
方法。re.search()
在字符串中搜索整个字符串并返回第一个匹配结果,re.match()
则只在字符串的开头进行匹配。
# 使用re.search()
pattern = r'hello'
text = 'hello world'
match = re.search(pattern, text)
if match:
print("Match found:", match.group())
else:
print("No match found")
使用re.match()
match = re.match(pattern, text)
if match:
print("Match found:", match.group())
else:
print("No match found")
3. 使用正则表达式的特殊字符
正则表达式使用一些特殊字符来表示不同的匹配模式,例如:
.
匹配任意字符(除了换行符)^
匹配字符串的开头$
匹配字符串的结尾*
匹配前一个字符0次或多次+
匹配前一个字符1次或多次?
匹配前一个字符0次或1次{n}
匹配前一个字符恰好n次{n,}
匹配前一个字符至少n次{n,m}
匹配前一个字符至少n次,至多m次[]
匹配括号内的任意一个字符|
表示或()
用于分组
示例:
pattern = r'\d{3}-\d{2}-\d{4}'
text = 'My number is 123-45-6789'
match = re.search(pattern, text)
if match:
print("Match found:", match.group())
else:
print("No match found")
4. 捕获组
捕获组用于提取匹配的子字符串。使用圆括号()
将模式分组,在匹配后可以通过group()
方法获取。
pattern = r'(\d{3})-(\d{2})-(\d{4})'
text = 'My number is 123-45-6789'
match = re.search(pattern, text)
if match:
print("Match found:", match.group())
print("Area code:", match.group(1))
print("Prefix:", match.group(2))
print("Line number:", match.group(3))
5. 替换
可以使用re.sub()
方法来替换匹配的字符串。
pattern = r'\d'
text = 'My number is 123-45-6789'
new_text = re.sub(pattern, '*', text)
print("Replaced text:", new_text)
二、字符串方法
Python的字符串方法也可以用于简单的字符匹配。以下是一些常见的字符串方法。
1. str.find()
str.find(sub)
返回子字符串sub
在字符串中的第一个出现位置,如果没有找到则返回-1。
text = 'hello world'
position = text.find('world')
if position != -1:
print("Found at position:", position)
else:
print("Not found")
2. str.startswith()
和str.endswith()
str.startswith(prefix)
检查字符串是否以指定前缀开头,str.endswith(suffix)
检查字符串是否以指定后缀结尾。
text = 'hello world'
if text.startswith('hello'):
print("Starts with 'hello'")
if text.endswith('world'):
print("Ends with 'world'")
3. str.count()
str.count(sub)
返回子字符串sub
在字符串中出现的次数。
text = 'hello world hello'
count = text.count('hello')
print("'hello' appears", count, "times")
4. str.replace()
str.replace(old, new)
返回一个新字符串,其中所有的old
子字符串被替换为new
。
text = 'hello world'
new_text = text.replace('world', 'Python')
print("Replaced text:", new_text)
三、列表解析
列表解析是一种简洁的列表生成方式,可以用来匹配和提取字符。
1. 基本用法
列表解析的基本语法是[expression for item in iterable if condition]
。
text = 'hello world'
matches = [char for char in text if char in 'aeiou']
print("Vowels:", matches)
2. 使用列表解析和正则表达式
可以将正则表达式与列表解析结合使用以实现更复杂的匹配。
import re
text = 'My numbers are 123-45-6789 and 987-65-4321'
pattern = re.compile(r'\d{3}-\d{2}-\d{4}')
matches = [match.group() for match in pattern.finditer(text)]
print("Matches:", matches)
四、综合实例
以下是一个综合实例,展示如何使用正则表达式、字符串方法和列表解析来匹配和处理字符。
1. 匹配电子邮件地址
使用正则表达式匹配电子邮件地址,并提取域名。
import re
text = 'Please contact us at support@example.com or sales@example.org'
pattern = r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}'
matches = re.findall(pattern, text)
domains = [match.split('@')[1] for match in matches]
print("Email addresses:", matches)
print("Domains:", domains)
2. 匹配电话号码
使用正则表达式匹配电话号码,并格式化输出。
import re
text = 'Contact us at 123-456-7890 or (123) 456-7890 or 123.456.7890'
pattern = r'\(?\d{3}\)?[-.\s]?\d{3}[-.\s]?\d{4}'
matches = re.findall(pattern, text)
formatted_numbers = [re.sub(r'[-.\s]', '-', match) for match in matches]
print("Phone numbers:", formatted_numbers)
3. 提取大写字母
使用列表解析提取字符串中的大写字母。
text = 'Hello World! Welcome to Python Programming.'
uppercase_letters = [char for char in text if char.isupper()]
print("Uppercase letters:", uppercase_letters)
五、总结
Python提供了多种方法来匹配和处理字符,包括正则表达式、字符串方法和列表解析。正则表达式是最强大和灵活的工具,适用于复杂的匹配任务;字符串方法适用于简单的匹配和替换任务;列表解析则提供了一种简洁的方式来处理字符。通过结合这些方法,可以高效地完成各种字符匹配和处理任务。希望本文对您在使用Python匹配字符时有所帮助。
相关问答FAQs:
如何在Python中使用正则表达式进行字符匹配?
在Python中,使用re
模块可以方便地进行字符匹配。通过re.compile()
函数定义正则表达式,然后使用search()
, match()
或findall()
等方法来查找匹配的字符。例如,re.findall(r'\d+', 'abc123def456')
可以找到字符串中的所有数字。
Python中有哪些常用的字符匹配方法?
常用的字符匹配方法包括re.search()
,用于查找字符串中首次出现的匹配;re.match()
,用于检查字符串的开头是否符合正则表达式;以及re.findall()
,用于返回所有匹配的字符串列表。此外,re.sub()
可以用于字符串替换,将匹配的字符替换为其他字符串。
如何提高Python字符匹配的性能?
提高字符匹配性能的一种方法是预编译正则表达式。使用re.compile()
可以将正则表达式编译成一个对象,这样在多次匹配时会更高效。此外,尽量简化正则表达式,避免使用过于复杂的模式,减少不必要的回溯,也有助于提升性能。
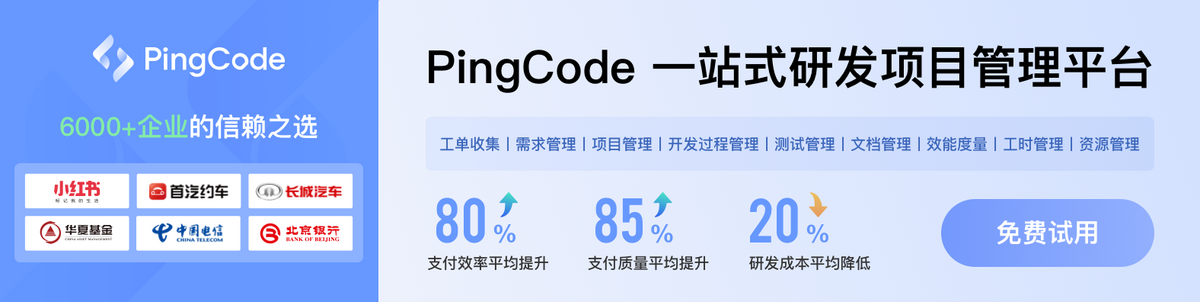