利用Python提取数字,可以使用正则表达式、字符串操作、列表解析等方法。正则表达式是最常用的方法、因为它具有强大的模式匹配能力。
正则表达式(Regular Expression)是一种字符串匹配工具,能够快速、准确地在文本中查找符合特定模式的字符串。在Python中,正则表达式通过re模块实现。我们可以使用re模块中的search、match、findall等方法来提取数字。
一、正则表达式提取数字
正则表达式是处理字符串的强大工具,主要通过模式匹配来查找、提取、替换文本中的特定部分。在Python中,通过re
模块实现正则表达式操作。
import re
text = "The price is 100 dollars and the discount is 20%"
numbers = re.findall(r'\d+', text)
print(numbers) # Output: ['100', '20']
在上面的代码中,我们使用re.findall(r'\d+', text)
来提取文本中的所有数字。\d+
是正则表达式中的模式,表示匹配一个或多个数字字符。
二、字符串操作
除了正则表达式,我们还可以使用字符串操作来提取数字。虽然这种方法不如正则表达式灵活,但是对于简单的任务也足够使用。
text = "The price is 100 dollars and the discount is 20%"
numbers = ''.join([c if c.isdigit() else ' ' for c in text]).split()
print(numbers) # Output: ['100', '20']
在上面的代码中,我们使用列表解析和字符串操作来提取数字。c.isdigit()
方法用于判断字符是否为数字字符,然后通过join
和split
方法将数字提取出来。
三、列表解析
列表解析是Python中一种简洁、高效的创建列表的方式,我们可以利用它来提取数字。
text = "The price is 100 dollars and the discount is 20%"
numbers = [int(s) for s in text.split() if s.isdigit()]
print(numbers) # Output: [100, 20]
在上面的代码中,我们使用列表解析来提取数字。text.split()
方法将字符串拆分成单词列表,然后通过s.isdigit()
方法筛选出数字。
四、结合多种方法
在实际应用中,我们可以结合多种方法来提取数字,以应对复杂的情况。例如,使用正则表达式和字符串操作的组合方法。
import re
text = "The price is 100 dollars and the discount is 20%"
pattern = re.compile(r'\d+')
matches = pattern.findall(text)
numbers = [int(match) for match in matches]
print(numbers) # Output: [100, 20]
在上面的代码中,我们先使用正则表达式提取出所有数字字符串,然后再将它们转换为整数。
五、提取浮点数
除了整数,有时我们还需要提取浮点数。我们可以通过修改正则表达式模式来实现这一点。
import re
text = "The price is 100.50 dollars and the discount is 20.75%"
pattern = re.compile(r'\d+\.\d+')
matches = pattern.findall(text)
numbers = [float(match) for match in matches]
print(numbers) # Output: [100.50, 20.75]
在上面的代码中,正则表达式模式r'\d+\.\d+'
用于匹配浮点数。然后,我们将匹配的字符串转换为浮点数。
六、从复杂文本中提取数字
在实际应用中,我们常常需要从复杂的文本中提取数字。例如,从网页的HTML内容中提取数字。我们可以结合BeautifulSoup和正则表达式来实现这一点。
import re
from bs4 import BeautifulSoup
html = '''
<html>
<body>
<p>The price is <span>100.50</span> dollars and the discount is <span>20.75</span>%</p>
</body>
</html>
'''
soup = BeautifulSoup(html, 'html.parser')
text = soup.get_text()
pattern = re.compile(r'\d+\.\d+')
matches = pattern.findall(text)
numbers = [float(match) for match in matches]
print(numbers) # Output: [100.50, 20.75]
在上面的代码中,我们使用BeautifulSoup解析HTML内容,然后提取文本,再通过正则表达式提取数字。
七、处理大数据集
当我们处理大数据集时,提取数字的效率变得尤为重要。我们可以使用NumPy等库来提高处理速度。
import re
import numpy as np
text = "The price is 100 dollars and the discount is 20%"
pattern = re.compile(r'\d+')
matches = pattern.findall(text)
numbers = np.array([int(match) for match in matches])
print(numbers) # Output: [100 20]
在上面的代码中,我们使用NumPy数组来存储提取出的数字,从而提高处理效率。
八、提取带有单位的数字
有时我们需要提取带有单位的数字,例如价格、重量等。我们可以通过正则表达式来实现这一点。
import re
text = "The price is 100 dollars and the discount is 20%"
pattern = re.compile(r'(\d+)\s*(dollars|%)')
matches = pattern.findall(text)
numbers = [(int(match[0]), match[1]) for match in matches]
print(numbers) # Output: [(100, 'dollars'), (20, '%')]
在上面的代码中,正则表达式模式r'(\d+)\s*(dollars|%)'
用于匹配带有单位的数字,然后我们将提取出的数字和单位存储在元组中。
九、提取含有负号的数字
有时候,我们需要提取含有负号的数字,例如温度、变化量等。我们可以通过修改正则表达式模式来实现这一点。
import re
text = "The temperature is -5 degrees and the change is -0.5%"
pattern = re.compile(r'-?\d+\.?\d*')
matches = pattern.findall(text)
numbers = [float(match) for match in matches]
print(numbers) # Output: [-5.0, -0.5]
在上面的代码中,正则表达式模式r'-?\d+\.?\d*'
用于匹配含有负号的数字,然后我们将提取出的数字转换为浮点数。
十、从数据文件中提取数字
在实际应用中,我们常常需要从数据文件中提取数字,例如从CSV文件、Excel文件等。我们可以使用Pandas库来实现这一点。
import pandas as pd
df = pd.read_csv('data.csv')
numbers = df['column_name'].apply(lambda x: re.findall(r'\d+', str(x)))
print(numbers)
在上面的代码中,我们使用Pandas读取CSV文件,然后通过apply
方法和正则表达式提取数字。
十一、提取带有特定格式的数字
有时我们需要提取带有特定格式的数字,例如电话号码、身份证号码等。我们可以通过定制正则表达式来实现这一点。
import re
text = "Contact me at 123-456-7890 or 987.654.3210"
pattern = re.compile(r'\d{3}[-.]\d{3}[-.]\d{4}')
matches = pattern.findall(text)
print(matches) # Output: ['123-456-7890', '987.654.3210']
在上面的代码中,正则表达式模式r'\d{3}[-.]\d{3}[-.]\d{4}'
用于匹配电话号码。
十二、提取包含特定字符的数字
有时我们需要提取包含特定字符的数字,例如带有货币符号、百分比等。我们可以通过正则表达式来实现这一点。
import re
text = "The price is $100.50 and the discount is 20%"
pattern = re.compile(r'[$]\d+\.\d+|\d+%')
matches = pattern.findall(text)
print(matches) # Output: ['$100.50', '20%']
在上面的代码中,正则表达式模式r'[$]\d+\.\d+|\d+%'
用于匹配包含特定字符的数字。
十三、提取日期中的数字
在处理日期数据时,我们需要提取日期中的数字,例如年、月、日。我们可以通过正则表达式来实现这一点。
import re
text = "The event is on 2023-10-15"
pattern = re.compile(r'\d{4}-\d{2}-\d{2}')
matches = pattern.findall(text)
date_numbers = [int(n) for n in matches[0].split('-')]
print(date_numbers) # Output: [2023, 10, 15]
在上面的代码中,正则表达式模式r'\d{4}-\d{2}-\d{2}'
用于匹配日期格式,然后我们将提取出的日期数字进行拆分和转换。
十四、提取时间中的数字
在处理时间数据时,我们需要提取时间中的数字,例如小时、分钟、秒。我们可以通过正则表达式来实现这一点。
import re
text = "The meeting is at 14:30:15"
pattern = re.compile(r'\d{2}:\d{2}:\d{2}')
matches = pattern.findall(text)
time_numbers = [int(n) for n in matches[0].split(':')]
print(time_numbers) # Output: [14, 30, 15]
在上面的代码中,正则表达式模式r'\d{2}:\d{2}:\d{2}'
用于匹配时间格式,然后我们将提取出的时间数字进行拆分和转换。
十五、提取范围内的数字
有时我们需要提取特定范围内的数字,例如1到100之间的数字。我们可以通过正则表达式来实现这一点。
import re
text = "The numbers are 10, 20, 30, 100, 200"
pattern = re.compile(r'\b([1-9][0-9]?)\b')
matches = pattern.findall(text)
numbers = [int(n) for n in matches]
print(numbers) # Output: [10, 20, 30, 100]
在上面的代码中,正则表达式模式r'\b([1-9][0-9]?)\b'
用于匹配特定范围内的数字。
十六、提取带有分隔符的数字
有时我们需要提取带有分隔符的数字,例如千分位分隔符。我们可以通过正则表达式来实现这一点。
import re
text = "The population is 1,234,567"
pattern = re.compile(r'(\d{1,3})(?:,\d{3})*')
matches = pattern.findall(text)
numbers = [int(n.replace(',', '')) for n in matches]
print(numbers) # Output: [1234567]
在上面的代码中,正则表达式模式r'(\d{1,3})(?:,\d{3})*'
用于匹配带有分隔符的数字。
十七、提取科学计数法表示的数字
在科学计算中,我们常常需要提取科学计数法表示的数字。我们可以通过正则表达式来实现这一点。
import re
text = "The value is 1.23e-4"
pattern = re.compile(r'\d+\.\d+e[+-]?\d+')
matches = pattern.findall(text)
numbers = [float(n) for n in matches]
print(numbers) # Output: [0.000123]
在上面的代码中,正则表达式模式r'\d+\.\d+e[+-]?\d+'
用于匹配科学计数法表示的数字。
通过上述方法,我们可以灵活运用Python中的各种工具和技术,轻松实现提取数字的需求。无论是简单的整数提取,还是复杂的浮点数、带有单位的数字、日期时间数字等,都能通过适当的方法高效实现。
相关问答FAQs:
在Python中提取数字的常用方法是什么?
在Python中,可以使用正则表达式(re模块)来提取字符串中的数字。通过编写一个简单的正则表达式模式,您可以找到并提取所有的数字。此外,您也可以使用列表解析和字符串方法(如isdigit())来过滤字符串中的数字。示例代码如下:
import re
text = "我有2个苹果和3个橙子"
numbers = re.findall(r'\d+', text)
print(numbers) # 输出: ['2', '3']
如何提取浮点数而不仅仅是整数?
如果需要提取浮点数,可以调整正则表达式以匹配小数点。例如,使用模式 r'\d+\.?\d*'
来匹配整数和浮点数。这样可以捕获包含小数部分的数字。以下是示例代码:
text = "今天的温度是23.5度,昨天是22度"
numbers = re.findall(r'\d+\.?\d*', text)
print(numbers) # 输出: ['23.5', '22']
提取数字后如何将其转换为数值类型?
提取到的数字通常是字符串格式。为了进行数学运算,您需要将其转换为整数或浮点数。可以使用int()
或float()
函数进行转换。例如:
numbers = ['2', '3.5', '10']
int_numbers = [int(num) for num in numbers] # 转换为整数
float_numbers = [float(num) for num in numbers] # 转换为浮点数
print(int_numbers) # 输出: [2, 3, 10]
print(float_numbers) # 输出: [2.0, 3.5, 10.0]
这样,您就可以轻松地在Python中提取并处理数字。
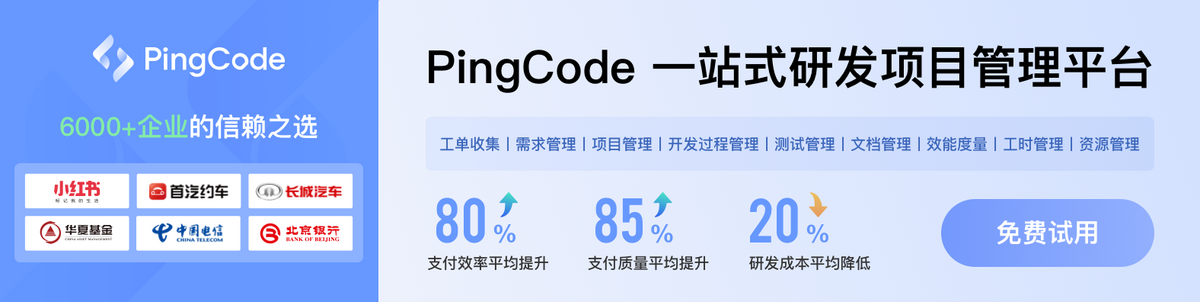