在Python中替换敏感字符的方法有很多种,常用的方法包括使用字符串的replace()方法、使用正则表达式的sub()方法、利用字典进行批量替换。其中,使用正则表达式的sub()方法最为灵活和强大。
正则表达式的sub()方法是Python中一个强大的字符串处理工具,可以用来查找和替换敏感字符。下面我将详细描述如何使用正则表达式来替换敏感字符。
一、使用字符串的replace()方法
字符串的replace()方法是最简单的替换方法,它适用于替换单个或少量的敏感字符。这个方法不会使用正则表达式,但它的语法非常简单直观。我们可以通过以下示例来了解如何使用它:
s = "Hello, this is a sensitive string."
s = s.replace("sensitive", "<strong></strong>*")
print(s) # 输出: Hello, this is a <strong></strong>* string.
二、使用正则表达式的sub()方法
正则表达式的sub()方法是一个强大的工具,它允许我们使用正则表达式模式来查找和替换字符串中的敏感字符。我们可以通过以下示例来了解如何使用它:
import re
s = "Hello, this is a sensitive string with bad words."
pattern = re.compile(r'sensitive|bad words')
s = pattern.sub("<strong></strong>*", s)
print(s) # 输出: Hello, this is a <strong></strong>* string with <strong></strong>*.
在上面的示例中,我们首先导入了re模块,然后定义了一个正则表达式模式,用来匹配敏感字符。接着,我们使用sub()方法将匹配到的敏感字符替换为“*”。
三、利用字典进行批量替换
当我们需要替换多个敏感字符时,使用字典可以使代码更简洁和易于维护。我们可以通过以下示例来了解如何使用字典进行批量替换:
import re
s = "Hello, this is a sensitive string with bad words and inappropriate content."
sensitive_words = {
"sensitive": "<strong></strong>*",
"bad words": "<strong></strong>*",
"inappropriate": "<strong></strong>*"
}
def replace_sensitive_words(text, words_dict):
pattern = re.compile("|".join(re.escape(key) for key in words_dict.keys()))
return pattern.sub(lambda x: words_dict[x.group()], text)
s = replace_sensitive_words(s, sensitive_words)
print(s) # 输出: Hello, this is a <strong></strong>* string with <strong></strong>* and <strong></strong>* content.
在上面的示例中,我们首先定义了一个字典来存储敏感字符及其替换值。接着,我们使用正则表达式来匹配字典中的敏感字符,并使用sub()方法进行替换。
四、处理复杂的敏感字符替换
在实际应用中,敏感字符替换可能会涉及到更复杂的情形,比如处理大小写不敏感的匹配、处理包含特殊字符的敏感词等。我们可以通过以下示例来了解如何处理这些复杂情形:
import re
s = "Hello, this is a Sensitive string with BAD words and inappropriate content."
sensitive_words = {
"sensitive": "<strong></strong>*",
"bad words": "<strong></strong>*",
"inappropriate": "<strong></strong>*"
}
def replace_sensitive_words(text, words_dict):
pattern = re.compile("|".join(re.escape(key) for key in words_dict.keys()), re.IGNORECASE)
return pattern.sub(lambda x: words_dict[x.group().lower()], text)
s = replace_sensitive_words(s, sensitive_words)
print(s) # 输出: Hello, this is a <strong></strong>* string with <strong></strong>* and <strong></strong>* content.
在上面的示例中,我们使用了re.IGNORECASE标志来使匹配过程不区分大小写,并在替换函数中将匹配到的敏感字符转换为小写,以便从字典中查找其替换值。
五、优化性能
在处理大规模文本数据时,性能是一个需要考虑的重要因素。以下是一些优化性能的方法:
- 使用预编译正则表达式:预编译正则表达式可以提高匹配效率,尤其是在需要多次使用同一个正则表达式时。
- 减少字典查找次数:在替换过程中,尽量减少对字典的重复查找,可以使用缓存技术来优化。
- 批量处理:对于大规模文本数据,可以将文本分块处理,以减少内存使用和提高处理速度。
import re
s = "Hello, this is a sensitive string with bad words and inappropriate content." * 10000
sensitive_words = {
"sensitive": "<strong></strong>*",
"bad words": "<strong></strong>*",
"inappropriate": "<strong></strong>*"
}
def replace_sensitive_words(text, words_dict):
pattern = re.compile("|".join(re.escape(key) for key in words_dict.keys()), re.IGNORECASE)
return pattern.sub(lambda x: words_dict[x.group().lower()], text)
预编译正则表达式
compiled_pattern = re.compile("|".join(re.escape(key) for key in sensitive_words.keys()), re.IGNORECASE)
使用缓存技术优化字典查找
cache = {}
def replace_with_cache(match):
word = match.group().lower()
if word not in cache:
cache[word] = sensitive_words[word]
return cache[word]
s = compiled_pattern.sub(replace_with_cache, s)
print(s[:1000]) # 输出前1000个字符
通过以上优化方法,我们可以显著提高大规模文本数据处理的性能。
六、总结
在本文中,我们介绍了在Python中替换敏感字符的几种常用方法,包括使用字符串的replace()方法、使用正则表达式的sub()方法、利用字典进行批量替换,以及处理复杂情形和优化性能的方法。希望这些方法能够帮助你更好地处理敏感字符替换问题。
相关问答FAQs:
如何在Python中识别敏感字符?
在Python中,可以通过使用正则表达式库re
来识别敏感字符。首先,定义一个字符串模式,包含所有需要替换的敏感字符。使用re.findall()
方法可以找到所有符合条件的字符,之后再进行替换。
有哪些常用的方法可以替换敏感字符?
Python提供了多种替换敏感字符的方法。常见的有使用str.replace()
方法直接替换特定字符,或使用re.sub()
方法来替换符合特定模式的字符。对于大量文本处理,re.sub()
往往更为灵活和高效。
替换敏感字符后,如何确保文本的完整性?
在替换敏感字符后,可以通过多种方式确保文本的完整性。使用str.isalnum()
方法可以检查文本中是否仅包含字母和数字,利用re.match()
来验证文本格式是否符合预期。此外,进行单元测试也是确保文本完整性的重要步骤,可以通过对比替换前后的内容来确认替换过程没有引入错误。
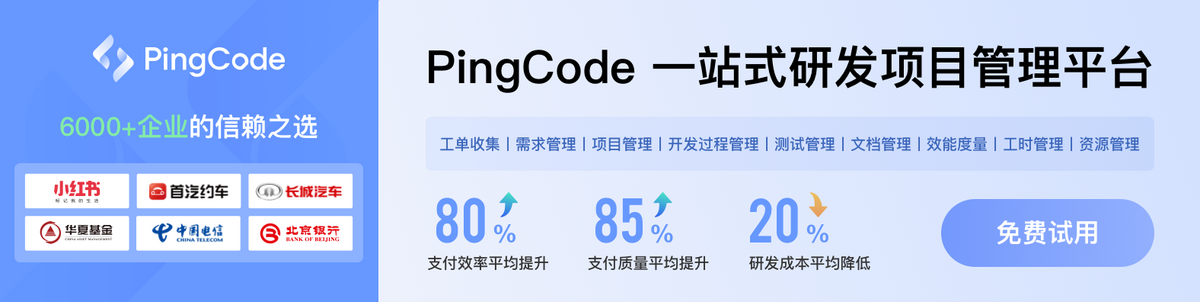