VBA可以通过调用外部程序来执行Python脚本,常见的方法有Shell函数、CreateObject函数、使用批处理文件等。其中,Shell函数是最常用的方法之一,因为它简单易用且适用于大部分需求。下面将详细介绍如何通过VBA调用Python程序,并举例说明每种方法的具体实现步骤。
一、Shell函数调用Python程序
使用Shell函数是最简单的方法之一。Shell函数用于在Microsoft Windows中启动其他程序。通过Shell函数,我们可以直接调用Python解释器来执行Python脚本。
Sub RunPythonScript()
Dim pythonPath As String
Dim scriptPath As String
Dim shellCommand As String
' 设置Python解释器的路径
pythonPath = "C:\Python39\python.exe"
' 设置要执行的Python脚本的路径
scriptPath = "C:\path\to\your\script.py"
' 构建Shell命令
shellCommand = pythonPath & " " & scriptPath
' 使用Shell函数运行Python脚本
Shell shellCommand, vbNormalFocus
End Sub
在上面的例子中,我们首先设置了Python解释器和Python脚本的路径,然后构建Shell命令并使用Shell函数运行Python脚本。注意,路径中包含空格时需要使用双引号括起来,以避免路径解析错误。
二、CreateObject函数调用Python程序
CreateObject函数用于创建和操作Automation对象。通过CreateObject函数,我们可以调用Windows脚本宿主(WSH)来运行Python脚本。
Sub RunPythonScriptWithWSH()
Dim shell As Object
Dim pythonPath As String
Dim scriptPath As String
Dim shellCommand As String
' 创建Windows脚本宿主对象
Set shell = CreateObject("WScript.Shell")
' 设置Python解释器的路径
pythonPath = "C:\Python39\python.exe"
' 设置要执行的Python脚本的路径
scriptPath = "C:\path\to\your\script.py"
' 构建Shell命令
shellCommand = pythonPath & " " & scriptPath
' 使用Windows脚本宿主运行Python脚本
shell.Run shellCommand, 1, True
' 释放对象
Set shell = Nothing
End Sub
在这个例子中,我们通过CreateObject函数创建了一个Windows脚本宿主对象,然后使用shell.Run方法来运行Python脚本。shell.Run方法的第三个参数为True时表示等待Python脚本执行完成后再继续执行VBA代码。
三、使用批处理文件调用Python程序
有时候为了简化调用过程,可以先创建一个批处理文件来运行Python脚本,然后在VBA中调用这个批处理文件。
- 创建一个批处理文件(例如run_python.bat),内容如下:
@echo off
C:\Python39\python.exe C:\path\to\your\script.py
- 在VBA中调用这个批处理文件:
Sub RunPythonScriptWithBatch()
Dim batchFilePath As String
' 设置批处理文件的路径
batchFilePath = "C:\path\to\your\run_python.bat"
' 使用Shell函数运行批处理文件
Shell batchFilePath, vbNormalFocus
End Sub
通过这种方法,我们可以将所有Python脚本相关的路径和参数配置都放在批处理文件中,简化了VBA代码中的配置。
四、Python脚本与VBA交互
在实际应用中,可能需要将Python脚本的执行结果返回给VBA程序。常见的方法是通过文件、剪贴板或标准输出进行数据交换。
- 使用文件进行数据交换
在Python脚本中将结果写入一个文件,然后在VBA中读取该文件。
Python脚本(script.py):
# script.py
result = "Hello from Python!"
with open("C:\\path\\to\\result.txt", "w") as file:
file.write(result)
VBA代码:
Sub RunPythonScriptAndReadResult()
Dim pythonPath As String
Dim scriptPath As String
Dim shellCommand As String
Dim resultFilePath As String
Dim fileContent As String
Dim fileNumber As Integer
' 设置Python解释器的路径
pythonPath = "C:\Python39\python.exe"
' 设置要执行的Python脚本的路径
scriptPath = "C:\path\to\your\script.py"
' 构建Shell命令
shellCommand = pythonPath & " " & scriptPath
' 使用Shell函数运行Python脚本
Shell shellCommand, vbNormalFocus
' 设置结果文件的路径
resultFilePath = "C:\path\to\result.txt"
' 等待Python脚本执行完成并生成结果文件
Application.Wait (Now + TimeValue("0:00:05"))
' 读取结果文件内容
fileNumber = FreeFile
Open resultFilePath For Input As #fileNumber
Line Input #fileNumber, fileContent
Close #fileNumber
' 显示结果
MsgBox fileContent
End Sub
- 使用剪贴板进行数据交换
在Python脚本中将结果复制到剪贴板,然后在VBA中读取剪贴板内容。需要安装pyperclip库来操作剪贴板。
Python脚本(script.py):
# script.py
import pyperclip
result = "Hello from Python!"
pyperclip.copy(result)
VBA代码:
Sub RunPythonScriptAndReadClipboard()
Dim pythonPath As String
Dim scriptPath As String
Dim shellCommand As String
Dim clipboardContent As String
' 设置Python解释器的路径
pythonPath = "C:\Python39\python.exe"
' 设置要执行的Python脚本的路径
scriptPath = "C:\path\to\your\script.py"
' 构建Shell命令
shellCommand = pythonPath & " " & scriptPath
' 使用Shell函数运行Python脚本
Shell shellCommand, vbNormalFocus
' 等待Python脚本执行完成并复制结果到剪贴板
Application.Wait (Now + TimeValue("0:00:05"))
' 读取剪贴板内容
clipboardContent = GetClipboardText()
' 显示结果
MsgBox clipboardContent
End Sub
Function GetClipboardText() As String
Dim DataObj As MSForms.DataObject
Set DataObj = New MSForms.DataObject
On Error GoTo ErrHandler
DataObj.GetFromClipboard
GetClipboardText = DataObj.GetText(1)
Exit Function
ErrHandler:
GetClipboardText = ""
End Function
- 使用标准输出进行数据交换
在Python脚本中将结果打印到标准输出,然后在VBA中通过Shell函数的重定向读取标准输出内容。实现这一点需要使用Windows命令行的输出重定向功能。
Python脚本(script.py):
# script.py
result = "Hello from Python!"
print(result)
VBA代码:
Sub RunPythonScriptAndReadStdout()
Dim pythonPath As String
Dim scriptPath As String
Dim shellCommand As String
Dim resultFilePath As String
Dim fileContent As String
Dim fileNumber As Integer
' 设置Python解释器的路径
pythonPath = "C:\Python39\python.exe"
' 设置要执行的Python脚本的路径
scriptPath = "C:\path\to\your\script.py"
' 设置结果文件的路径
resultFilePath = "C:\path\to\result.txt"
' 构建Shell命令,并将输出重定向到结果文件
shellCommand = pythonPath & " " & scriptPath & " > " & resultFilePath
' 使用Shell函数运行Python脚本
Shell shellCommand, vbNormalFocus
' 等待Python脚本执行完成并生成结果文件
Application.Wait (Now + TimeValue("0:00:05"))
' 读取结果文件内容
fileNumber = FreeFile
Open resultFilePath For Input As #fileNumber
Line Input #fileNumber, fileContent
Close #fileNumber
' 显示结果
MsgBox fileContent
End Sub
通过上述方法,我们可以实现VBA与Python脚本之间的数据交换。根据具体需求选择合适的方法,可以实现不同的交互效果。
总之,通过以上介绍的几种方法,VBA可以方便地调用Python程序并实现与Python脚本的交互。根据实际需求选择合适的方法,可以提高工作效率和程序的灵活性。无论是简单的Shell函数调用,还是通过文件、剪贴板或标准输出进行数据交换,都可以满足不同场景下的需求。
相关问答FAQs:
如何在VBA中调用Python程序?
在VBA中调用Python程序的常用方法是通过Shell命令。可以通过创建一个VBA宏,使用Shell函数来执行Python脚本。例如,您可以编写如下代码:
Sub RunPythonScript()
Dim Ret_Val
Ret_Val = Shell("C:\Path\To\Python.exe C:\Path\To\YourScript.py", vbNormalFocus)
End Sub
确保将路径替换为您的Python解释器和脚本的实际路径。
在VBA中如何处理Python程序的输出结果?
如果您希望获取Python程序的输出结果,可以考虑将输出写入一个文本文件或Excel单元格。在Python脚本中,您可以使用文件写入功能来将结果保存。然后在VBA中读取这个文件或直接从Excel中获取数据。
是否需要安装特定的库才能在VBA中调用Python?
在VBA中调用Python程序本身不需要额外的库,但为了让Python能够执行特定的任务,您可能需要安装相关的Python库。例如,如果您使用Pandas进行数据处理,确保在Python环境中安装了Pandas库。此外,确保您的Python环境已正确配置并添加到系统的环境变量中,以便在Shell命令中调用。
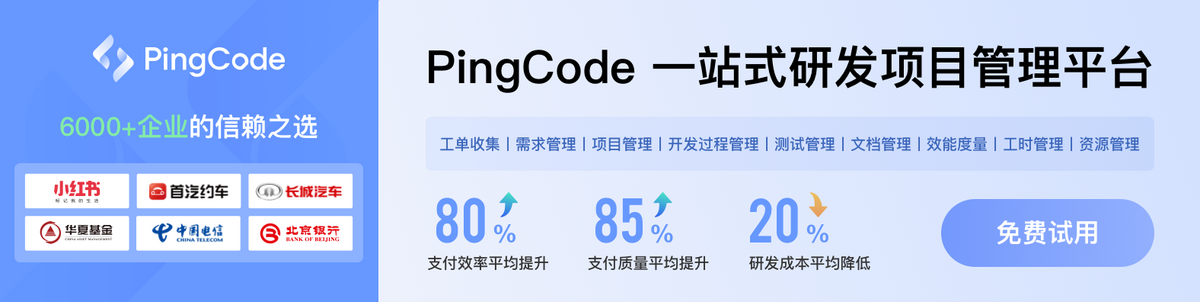