利用Python快速生成大文件的方法包括:使用循环写入、利用生成器生成数据、使用随机数据生成库、并行处理。 其中,使用循环写入是一种直观且常用的方法,通过不断地写入数据来生成大文件。下面将详细介绍这种方法。
使用循环写入是一种常见的方法,通过在循环中不断地写入数据,可以快速生成大文件。以下是一个简单的示例代码:
with open("large_file.txt", "w") as file:
for _ in range(1000000):
file.write("This is a line in a large file.\n")
该代码将生成一个包含1000000行文本的大文件。我们接下来将详细介绍如何利用Python生成大文件的几种方法。
一、使用循环写入
循环写入是最简单直观的方法。通过在循环中不断写入数据,可以快速生成大文件。以下是几种具体实现方式。
1. 使用字符串数据
可以通过在循环中写入字符串数据来生成大文件。这种方法简单直接,非常适合生成文本文件。
with open("large_file.txt", "w") as file:
for _ in range(1000000):
file.write("This is a line in a large file.\n")
2. 使用数字数据
生成包含数字数据的文件也是一种常见需求。例如,可以生成一个包含大量随机数的文件。
import random
with open("large_file.txt", "w") as file:
for _ in range(1000000):
file.write(f"{random.randint(0, 1000000)}\n")
3. 使用二进制数据
生成大文件不仅限于文本文件,有时也需要生成包含二进制数据的文件。以下示例展示如何生成一个包含随机字节的大文件。
import os
with open("large_file.bin", "wb") as file:
for _ in range(1000): # Adjust the range for desired file size
file.write(os.urandom(1024)) # Write 1024 random bytes
二、使用生成器生成数据
生成器是一种强大的工具,可以在生成数据的同时节省内存。通过使用生成器,可以逐步生成数据并写入文件。
1. 生成文本数据
以下示例展示如何使用生成器生成文本数据并写入文件。
def text_generator(num_lines):
for _ in range(num_lines):
yield "This is a line in a large file.\n"
with open("large_file.txt", "w") as file:
for line in text_generator(1000000):
file.write(line)
2. 生成随机数数据
可以使用生成器生成随机数数据,并将其写入文件。
import random
def random_number_generator(num_numbers):
for _ in range(num_numbers):
yield f"{random.randint(0, 1000000)}\n"
with open("large_file.txt", "w") as file:
for number in random_number_generator(1000000):
file.write(number)
3. 生成二进制数据
生成二进制数据时,生成器也可以发挥作用。
import os
def binary_data_generator(num_chunks, chunk_size):
for _ in range(num_chunks):
yield os.urandom(chunk_size)
with open("large_file.bin", "wb") as file:
for chunk in binary_data_generator(1000, 1024): # Adjust the parameters for desired file size
file.write(chunk)
三、使用随机数据生成库
Python的许多库可以帮助生成随机数据。例如,Faker库可以生成随机的伪数据,非常适合生成测试数据。
1. 安装Faker库
首先,安装Faker库:
pip install faker
2. 使用Faker生成文本数据
Faker库可以生成各种类型的伪数据,如姓名、地址、电子邮件等。
from faker import Faker
fake = Faker()
with open("large_file.txt", "w") as file:
for _ in range(1000000):
file.write(f"{fake.name()}, {fake.address()}\n")
3. 使用Faker生成结构化数据
可以使用Faker生成结构化数据,例如CSV格式的数据。
import csv
from faker import Faker
fake = Faker()
with open("large_file.csv", "w", newline='') as file:
writer = csv.writer(file)
writer.writerow(["Name", "Address", "Email"])
for _ in range(1000000):
writer.writerow([fake.name(), fake.address(), fake.email()])
四、并行处理
对于特别大的文件,单线程写入可能效率不高。可以考虑使用并行处理来加速大文件的生成。Python的multiprocessing
库可以帮助实现并行处理。
1. 使用多进程生成数据
以下示例展示如何使用多进程生成大文件。
from multiprocessing import Process
import os
def write_large_file(filename, num_chunks, chunk_size):
with open(filename, "wb") as file:
for _ in range(num_chunks):
file.write(os.urandom(chunk_size))
num_processes = 4
chunk_size = 1024 * 1024 # 1 MB
num_chunks_per_process = 250 # Adjust the parameters for desired file size
processes = []
for i in range(num_processes):
p = Process(target=write_large_file, args=(f"large_file_{i}.bin", num_chunks_per_process, chunk_size))
processes.append(p)
p.start()
for p in processes:
p.join()
2. 合并文件
如果使用多进程生成了多个文件,可以在最后将这些文件合并成一个大文件。
filenames = [f"large_file_{i}.bin" for i in range(num_processes)]
with open("final_large_file.bin", "wb") as final_file:
for filename in filenames:
with open(filename, "rb") as file:
final_file.write(file.read())
os.remove(filename) # Optional: remove the temporary files
以上介绍了几种利用Python快速生成大文件的方法,包括使用循环写入、生成器生成数据、随机数据生成库以及并行处理。根据具体需求,选择适合的方法可以高效地生成大文件。
相关问答FAQs:
如何使用Python生成大文件的主要方法有哪些?
在Python中,生成大文件的常用方法包括使用标准库中的文件操作函数,比如open()
和write()
。你可以通过循环结构将大量数据写入文件,或者利用random
模块生成随机内容。此外,使用pandas
库可以方便地处理大规模数据集,生成CSV或Excel文件。根据需求,选择合适的方法可以提高文件生成的效率。
生成大文件时,如何控制文件大小和内容格式?
控制文件大小可以通过在循环中设置具体的数据量来实现,例如在写入时指定每次写入的字节数。对于内容格式,用户可以根据需求选择文本格式(如CSV、JSON、TXT等)或二进制格式。在创建CSV文件时,可以使用pandas
库来定义每列的数据类型和格式,确保生成的文件符合特定要求。
在生成大文件时,有哪些性能优化的建议?
为了提高生成大文件的性能,可以考虑使用缓冲写入的方法,减少频繁的磁盘I/O操作。此外,使用with open()
语句可以确保文件在写入后正确关闭,避免资源浪费。使用多线程或异步IO也能加快文件生成的速度,尤其是在处理复杂数据时。此外,尽量减少数据处理的复杂度,确保数据生成过程高效流畅。
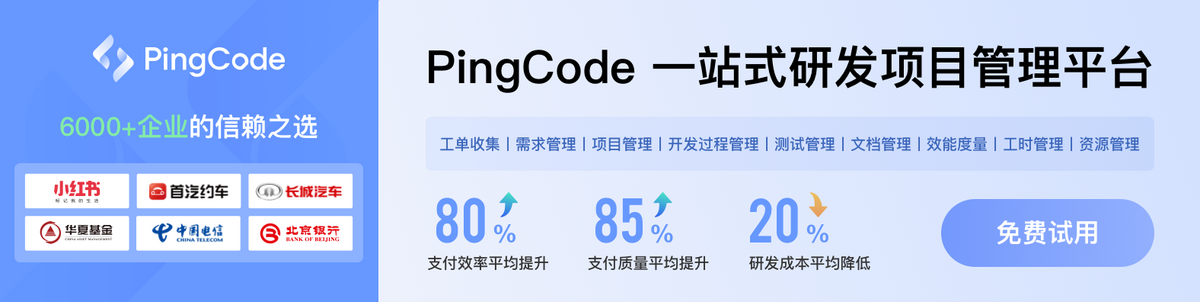