在Python中定义函数及使用的步骤如下:使用def关键字定义函数、在函数体内编写逻辑代码、使用函数名加括号调用函数。我们可以通过一个简单的示例来详细讲解如何定义和使用函数。在Python中定义函数,首先使用def
关键字,然后是函数名和一对括号,括号中可以包含参数列表。接着在函数体内编写逻辑代码,最后通过函数名加括号来调用函数。
一、定义函数
在Python中,定义函数的基本语法结构如下:
def function_name(parameters):
"""
Docstring explaining the function.
"""
# Function body
statement(s)
return value
1、使用def
关键字
定义函数需要使用def
关键字,它用来声明一个新的函数。函数名应该是描述性强且遵循Python命名规范的标识符。
2、参数列表
参数列表包含在括号内,可以为空或包含多个参数。参数在函数调用时传递值给函数。
3、函数体
函数体是缩进代码块,包含函数的逻辑操作。通常使用return
语句返回值。
4、Docstring
函数定义内可以包含一个文档字符串(docstring),用于描述函数的用途和使用方法。docstring通常使用三重引号。
def greet(name):
"""
This function greets the person passed in as a parameter.
"""
print(f"Hello, {name}!")
二、调用函数
调用函数时使用函数名加一对括号,括号内传入实际参数。
1、无参函数
如果函数不需要参数,只需调用函数名加一对空括号。
def say_hello():
"""
This function prints a hello message.
"""
print("Hello, World!")
Call the function
say_hello()
2、带参函数
如果函数需要参数,调用时传入实际参数。
def greet(name):
"""
This function greets the person passed in as a parameter.
"""
print(f"Hello, {name}!")
Call the function with an argument
greet("Alice")
3、返回值函数
函数可以返回值,通过return
语句实现。
def add(a, b):
"""
This function returns the sum of two numbers.
"""
return a + b
Call the function and store the return value
result = add(3, 5)
print(result) # Output: 8
三、函数参数类型
Python函数可以接受不同类型的参数,如位置参数、关键字参数、默认参数和可变参数。
1、位置参数
位置参数是最常见的参数类型,按顺序传递给函数。
def multiply(a, b):
"""
This function multiplies two numbers.
"""
return a * b
Call the function with positional arguments
result = multiply(4, 5)
print(result) # Output: 20
2、关键字参数
关键字参数在调用时使用参数名指定传递值。
def divide(a, b):
"""
This function divides the first number by the second number.
"""
return a / b
Call the function with keyword arguments
result = divide(a=10, b=2)
print(result) # Output: 5.0
3、默认参数
默认参数在函数定义时指定默认值,调用时可省略传递值。
def greet(name, message="Hello"):
"""
This function greets a person with a given message.
"""
print(f"{message}, {name}!")
Call the function with default parameter
greet("Alice") # Output: Hello, Alice!
Call the function with custom message
greet("Alice", "Good morning") # Output: Good morning, Alice!
4、可变参数
可变参数允许函数接受任意数量的参数,使用*args
和kwargs
。
*args
*args
用于传递可变数量的位置参数。
def sum_all(*args):
"""
This function returns the sum of all arguments.
"""
return sum(args)
Call the function with variable number of arguments
result = sum_all(1, 2, 3, 4)
print(result) # Output: 10
kwargs
kwargs
用于传递可变数量的关键字参数。
def print_info(kwargs):
"""
This function prints key-value pairs of all arguments.
"""
for key, value in kwargs.items():
print(f"{key}: {value}")
Call the function with variable number of keyword arguments
print_info(name="Alice", age=30, city="New York")
四、函数嵌套与作用域
1、嵌套函数
函数可以嵌套定义,即在一个函数内定义另一个函数。
def outer_function():
"""
This is the outer function.
"""
def inner_function():
"""
This is the inner function.
"""
print("Inner function")
print("Outer function")
inner_function()
Call the outer function
outer_function()
2、作用域
Python的变量作用域分为局部作用域和全局作用域。局部变量在函数内定义,全局变量在函数外定义。
global_var = "I am global"
def my_function():
local_var = "I am local"
print(global_var) # Access global variable
print(local_var) # Access local variable
Call the function
my_function()
Access global variable outside function
print(global_var)
Access local variable outside function (This will raise an error)
print(local_var)
五、匿名函数(Lambda)
Python提供了lambda关键字用于创建匿名函数(即没有名字的函数),常用于简单的函数定义。
# Define a lambda function
add = lambda x, y: x + y
Call the lambda function
result = add(3, 5)
print(result) # Output: 8
六、函数的高级用法
1、函数作为参数
函数可以作为参数传递给另一个函数。
def apply_function(func, value):
"""
This function applies the passed function to the value.
"""
return func(value)
def square(x):
"""
This function returns the square of a number.
"""
return x * x
Pass the square function as an argument
result = apply_function(square, 4)
print(result) # Output: 16
2、函数作为返回值
函数可以作为另一个函数的返回值。
def outer_function():
"""
This function returns an inner function.
"""
def inner_function():
print("Hello from inner function")
return inner_function
Get the inner function
inner_func = outer_function()
Call the inner function
inner_func()
3、装饰器
装饰器是用于修改函数或方法行为的高级函数,使用@decorator_name
语法。
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
Call the decorated function
say_hello()
4、递归函数
递归函数是调用自身的函数,常用于解决分治问题。
def factorial(n):
"""
This function returns the factorial of a number using recursion.
"""
if n == 1:
return 1
else:
return n * factorial(n - 1)
Call the recursive function
result = factorial(5)
print(result) # Output: 120
通过以上内容,你可以掌握在Python中定义和使用函数的各种方法和技巧。无论是简单的函数定义,还是高级用法如装饰器和递归函数,这些知识将帮助你在Python编程中灵活运用函数,提高代码的可读性和复用性。
相关问答FAQs:
在Python中定义函数时需要遵循哪些基本规则?
在Python中定义函数时,需要使用def
关键字,后面跟上函数名和括号。函数名应遵循命名规范,通常使用小写字母和下划线分隔。括号内可以定义参数,参数之间用逗号分隔。函数体需要缩进,通常使用四个空格。一个简单的函数示例是:
def my_function(param1, param2):
return param1 + param2
如何在Python中调用一个已定义的函数?
调用一个已定义的函数非常简单,只需使用函数名并传入相应的参数。例如,若有一个名为my_function
的函数,可以这样调用它:
result = my_function(5, 10)
print(result) # 输出结果为 15
确保传递给函数的参数数量与定义时一致,这样才能正确执行。
在Python函数中如何处理默认参数?
在Python中,可以为函数参数设置默认值,这样调用函数时可以选择性地传入参数。如果未提供参数,默认值将被使用。定义默认参数的方式如下:
def greet(name="Guest"):
return f"Hello, {name}!"
调用时,若不传入任何值,函数将返回 "Hello, Guest!";若传入值如greet("Alice")
,则返回 "Hello, Alice!"。这种方式使得函数更加灵活和易于使用。
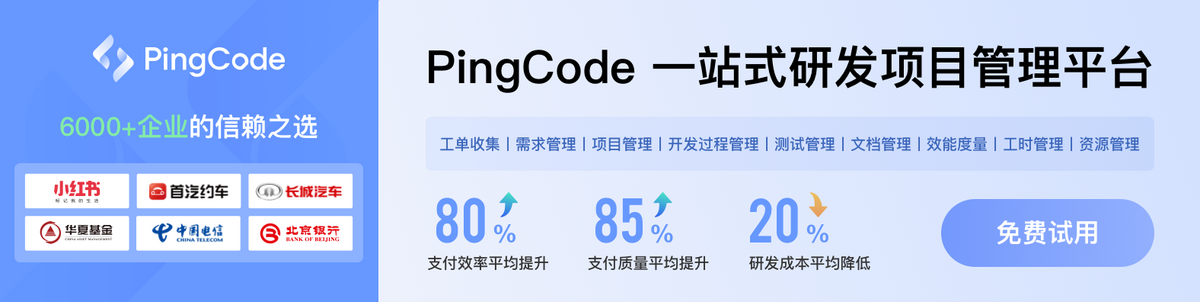