要使用Python从已知的图片地址下载图片,可以使用几个不同的库。最常见的方法是使用 requests
库或 urllib
库,因为它们都非常适合处理HTTP请求。此外,Pillow
库也可以用于图像处理。下面将详细介绍如何使用这些库来下载图片。
一、使用 requests
库
requests
是一个用于发送HTTP请求的库,非常适合用于下载图片。下面是一个简单的示例,展示如何使用 requests
库下载图片。
import requests
def download_image(url, save_path):
# 发送HTTP GET请求
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
with open(save_path, 'wb') as f:
f.write(response.content)
print(f"Image successfully downloaded: {save_path}")
else:
print(f"Failed to retrieve image. HTTP Status code: {response.status_code}")
示例图片URL
image_url = "https://example.com/image.jpg"
保存路径
save_path = "downloaded_image.jpg"
下载图片
download_image(image_url, save_path)
二、使用 urllib
库
urllib
是Python标准库的一部分,也可以用于下载图片。以下是使用 urllib
库下载图片的示例:
import urllib.request
def download_image(url, save_path):
try:
urllib.request.urlretrieve(url, save_path)
print(f"Image successfully downloaded: {save_path}")
except Exception as e:
print(f"Failed to retrieve image. Error: {str(e)}")
示例图片URL
image_url = "https://example.com/image.jpg"
保存路径
save_path = "downloaded_image.jpg"
下载图片
download_image(image_url, save_path)
三、使用 Pillow
库进行图像处理
Pillow
是一个强大的图像处理库,可以与其他库结合使用。虽然它本身不能下载图片,但可以用来处理下载的图片。下面是一个示例,展示如何结合 requests
和 Pillow
来下载并处理图片。
import requests
from PIL import Image
from io import BytesIO
def download_and_process_image(url, save_path):
# 发送HTTP GET请求
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
# 使用Pillow处理图像
image = Image.open(BytesIO(response.content))
image.save(save_path)
print(f"Image successfully downloaded and processed: {save_path}")
else:
print(f"Failed to retrieve image. HTTP Status code: {response.status_code}")
示例图片URL
image_url = "https://example.com/image.jpg"
保存路径
save_path = "processed_image.jpg"
下载并处理图片
download_and_process_image(image_url, save_path)
四、其他方法和建议
1、使用 aiohttp
实现异步下载
对于需要同时下载多个图片的情况,可以使用 aiohttp
库进行异步下载,以提高效率。
import aiohttp
import asyncio
async def download_image(session, url, save_path):
async with session.get(url) as response:
if response.status == 200:
content = await response.read()
with open(save_path, 'wb') as f:
f.write(content)
print(f"Image successfully downloaded: {save_path}")
else:
print(f"Failed to retrieve image. HTTP Status code: {response.status}")
async def main(urls):
async with aiohttp.ClientSession() as session:
tasks = [download_image(session, url, f"image_{i}.jpg") for i, url in enumerate(urls)]
await asyncio.gather(*tasks)
示例图片URL列表
image_urls = ["https://example.com/image1.jpg", "https://example.com/image2.jpg"]
下载图片
asyncio.run(main(image_urls))
2、处理不同类型的图片
在下载图片时,可能会遇到不同类型的图片(如JPG、PNG、GIF等)。确保根据文件类型选择合适的文件扩展名,以避免图像处理错误。
3、错误处理和重试机制
在实际应用中,网络请求可能会失败。可以添加错误处理和重试机制来提高下载的可靠性。
import requests
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.util.retry import Retry
def download_image(url, save_path):
session = requests.Session()
retry = Retry(total=5, backoff_factor=1, status_forcelist=[500, 502, 503, 504])
adapter = HTTPAdapter(max_retries=retry)
session.mount('http://', adapter)
session.mount('https://', adapter)
response = session.get(url)
if response.status_code == 200:
with open(save_path, 'wb') as f:
f.write(response.content)
print(f"Image successfully downloaded: {save_path}")
else:
print(f"Failed to retrieve image. HTTP Status code: {response.status_code}")
示例图片URL
image_url = "https://example.com/image.jpg"
保存路径
save_path = "downloaded_image.jpg"
下载图片
download_image(image_url, save_path)
综上所述,下载图片的方法有很多,具体选择哪一种方法取决于实际需求和场景。使用 requests
库是最简单和常见的方法,但在处理大规模下载或需要异步操作时,aiohttp
库可能更适合。无论选择哪种方法,都应注意错误处理和文件类型的正确处理,以确保下载图片的可靠性和兼容性。
相关问答FAQs:
如何在Python中使用URL下载图片?
要下载图片,首先需要使用Python的请求库(如requests
)获取图片的内容。以下是一个简单的示例代码:
import requests
url = '图片的URL地址'
response = requests.get(url)
if response.status_code == 200:
with open('下载的图片.jpg', 'wb') as file:
file.write(response.content)
else:
print("下载失败,状态码:", response.status_code)
这个代码片段会从指定的URL下载图片并保存为下载的图片.jpg
文件。
下载图片时需要注意哪些问题?
在下载图片时,有几个注意事项。首先,确保你有访问该图片的权限,避免侵犯版权。其次,检查图片的URL是否有效,避免404错误。此外,处理大型文件时,可以考虑分块下载以节省内存。
如何处理下载图片时的异常情况?
在下载图片时,可能会遇到多种异常情况,例如网络问题或URL错误。可以使用try-except
语句来捕获这些异常,确保程序不会因错误而崩溃。例如:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
except requests.exceptions.RequestException as e:
print("下载过程中出现错误:", e)
通过这种方式,可以更好地控制下载过程中的错误处理。
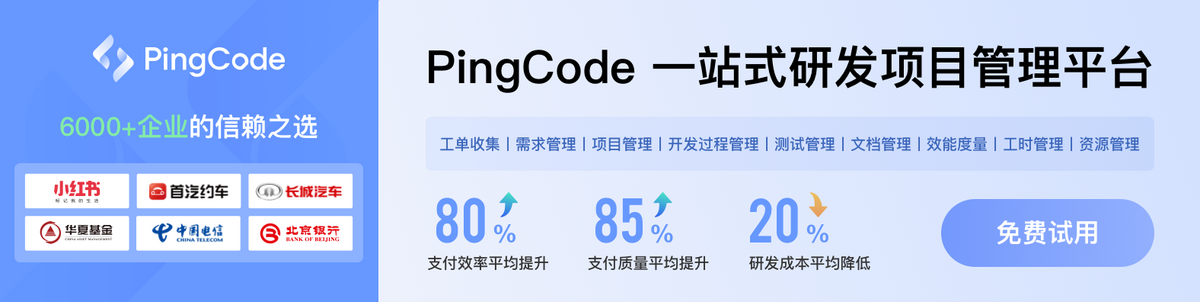