在Python中,根据货币符号自动转换需要使用货币符号识别、汇率获取和转换逻辑。可以使用库如forex-python或currencyconverter来完成此任务。下面将详细介绍如何实现这一过程。
一、识别货币符号
识别货币符号是货币转换的第一步。货币符号通常是由一个或两个字符组成的,如$代表美元,€代表欧元,¥代表日元等。我们可以使用Python中的字典来存储这些货币符号及其对应的货币代码。例如:
currency_symbols = {
'$': 'USD',
'€': 'EUR',
'¥': 'JPY',
'£': 'GBP',
# 其他货币符号
}
二、获取汇率
获取汇率是货币转换的第二步。我们可以使用forex-python或currencyconverter库来获取实时的汇率。例如,使用forex-python库:
from forex_python.converter import CurrencyRates
def get_exchange_rate(from_currency, to_currency):
c = CurrencyRates()
return c.get_rate(from_currency, to_currency)
三、执行转换
执行转换是最后一步。我们可以使用已获取的汇率进行货币转换。例如:
def convert_currency(amount, from_currency, to_currency):
exchange_rate = get_exchange_rate(from_currency, to_currency)
return amount * exchange_rate
四、综合示例
下面是一个综合示例,展示了如何根据货币符号自动进行货币转换:
from forex_python.converter import CurrencyRates
货币符号和货币代码的映射
currency_symbols = {
'$': 'USD',
'€': 'EUR',
'¥': 'JPY',
'£': 'GBP',
# 其他货币符号
}
获取汇率的函数
def get_exchange_rate(from_currency, to_currency):
c = CurrencyRates()
return c.get_rate(from_currency, to_currency)
货币转换的函数
def convert_currency(amount, from_currency, to_currency):
exchange_rate = get_exchange_rate(from_currency, to_currency)
return amount * exchange_rate
根据货币符号自动转换货币的函数
def auto_convert_currency(amount_with_symbol, to_currency):
symbol = amount_with_symbol[0]
amount = float(amount_with_symbol[1:])
from_currency = currency_symbols.get(symbol)
if from_currency:
converted_amount = convert_currency(amount, from_currency, to_currency)
return converted_amount
else:
raise ValueError("无法识别的货币符号")
示例
amount_with_symbol = '$100'
to_currency = 'EUR'
converted_amount = auto_convert_currency(amount_with_symbol, to_currency)
print(f"{amount_with_symbol} 转换为 {to_currency} 后的金额为: {converted_amount:.2f}")
在上述示例中,我们首先定义了一个字典currency_symbols
来存储货币符号和货币代码的映射关系。然后,我们定义了三个函数:get_exchange_rate
用于获取汇率,convert_currency
用于执行货币转换,auto_convert_currency
用于根据货币符号自动进行货币转换。最后,我们展示了一个示例,演示如何使用这些函数进行货币转换。
五、处理更多货币符号
如果需要处理更多的货币符号,可以在currency_symbols
字典中添加更多的符号和代码映射。例如:
currency_symbols = {
'$': 'USD',
'€': 'EUR',
'¥': 'JPY',
'£': 'GBP',
'₹': 'INR',
'₽': 'RUB',
'₩': 'KRW',
'₣': 'CHF',
'C$': 'CAD',
'A$': 'AUD',
'NZ$': 'NZD',
'HK$': 'HKD',
# 其他货币符号
}
六、处理特殊情况
在某些情况下,货币符号可能不唯一,例如,$符号可以代表美元、加拿大元、澳大利亚元等。为了处理这种情况,可以在输入金额时使用两个字符的符号(如C$代表加拿大元,A$代表澳大利亚元),或者通过用户交互来明确货币类型。
def auto_convert_currency(amount_with_symbol, to_currency):
if amount_with_symbol[:2] in currency_symbols:
symbol = amount_with_symbol[:2]
amount = float(amount_with_symbol[2:])
else:
symbol = amount_with_symbol[0]
amount = float(amount_with_symbol[1:])
from_currency = currency_symbols.get(symbol)
if from_currency:
converted_amount = convert_currency(amount, from_currency, to_currency)
return converted_amount
else:
raise ValueError("无法识别的货币符号")
七、优化性能
如果需要频繁进行货币转换,可以优化性能,例如缓存汇率结果,以减少API调用次数:
from functools import lru_cache
@lru_cache(maxsize=128)
def get_cached_exchange_rate(from_currency, to_currency):
return get_exchange_rate(from_currency, to_currency)
八、处理异常
在实际应用中,可能会遇到网络问题或API调用失败的情况。需要在代码中加入异常处理:
def get_exchange_rate(from_currency, to_currency):
try:
c = CurrencyRates()
return c.get_rate(from_currency, to_currency)
except Exception as e:
print(f"获取汇率失败: {e}")
return None
九、用户界面
如果需要提供用户界面,可以使用tkinter
库创建一个简单的GUI应用:
import tkinter as tk
from tkinter import messagebox
def on_convert():
amount_with_symbol = entry_amount.get()
to_currency = entry_to_currency.get()
try:
converted_amount = auto_convert_currency(amount_with_symbol, to_currency)
messagebox.showinfo("转换结果", f"{amount_with_symbol} 转换为 {to_currency} 后的金额为: {converted_amount:.2f}")
except ValueError as e:
messagebox.showerror("错误", str(e))
创建GUI
root = tk.Tk()
root.title("货币转换器")
tk.Label(root, text="输入金额(带符号):").grid(row=0, column=0)
entry_amount = tk.Entry(root)
entry_amount.grid(row=0, column=1)
tk.Label(root, text="目标货币代码:").grid(row=1, column=0)
entry_to_currency = tk.Entry(root)
entry_to_currency.grid(row=1, column=1)
tk.Button(root, text="转换", command=on_convert).grid(row=2, columnspan=2)
root.mainloop()
通过上述示例,我们可以创建一个简单的GUI应用,用户可以输入带货币符号的金额和目标货币代码,并点击按钮进行货币转换。
总结
在Python中,根据货币符号自动转换货币涉及货币符号识别、汇率获取和转换逻辑。通过使用forex-python或currencyconverter库,我们可以轻松实现这一过程。本文详细介绍了如何实现货币转换,并提供了处理更多货币符号、优化性能、处理异常和创建用户界面的方法。希望本文对您有所帮助。
相关问答FAQs:
如何在Python中识别不同的货币符号?
在Python中,可以使用正则表达式和字符串处理功能来识别不同的货币符号。常见的货币符号如美元($)、欧元(€)、英镑(£)等,可以通过编写相应的模式来匹配。使用re
模块,可以轻松地从字符串中提取货币符号,以便后续的转换处理。
Python中是否有库可以帮助进行货币转换?
是的,Python中有多个库可以帮助进行货币转换,比如forex-python
和money
库。这些库提供了简单的API来获取当前的汇率并进行货币转换,用户只需输入金额和源货币符号,库会自动返回相应的目标货币金额。
如何处理货币转换中的小数点和四舍五入问题?
货币转换中通常需要注意小数点的处理和四舍五入。Python中的Decimal
模块可以帮助用户进行高精度的计算,避免浮点数计算带来的误差。使用quantize()
方法可以设置小数点后的位数,从而确保输出的金额符合货币格式要求。
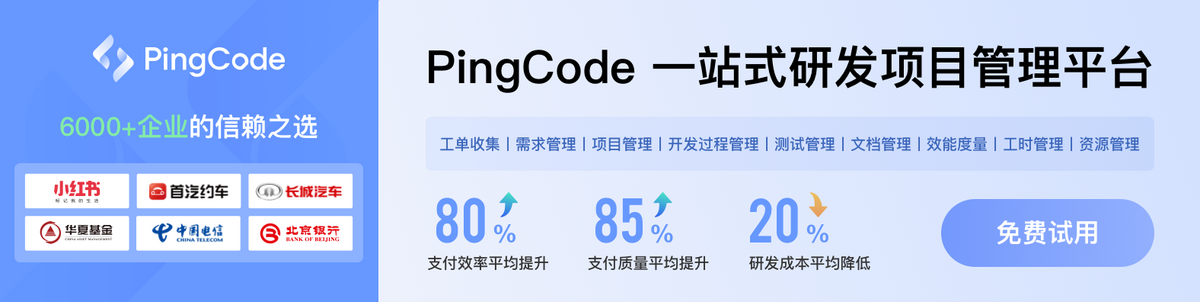