在Python中取出字符串的方法有很多,如使用索引、切片、字符串方法、正则表达式等。在本文中,我将详细介绍这些方法的使用,并提供一些实用的例子。最常用的方法包括:使用索引、切片、字符串方法、正则表达式等。特别是使用索引和切片可以非常方便地提取字符串中的特定部分。
一、使用索引
Python字符串是一个字符序列,每个字符都有一个索引。索引从0开始,负索引从-1开始。通过索引,我们可以获取字符串中的特定字符。
1、正向索引
正向索引从0开始,表示从字符串的开头到结尾。
s = "Hello, World!"
print(s[0]) # 输出: H
print(s[7]) # 输出: W
2、负向索引
负向索引从-1开始,表示从字符串的结尾到开头。
s = "Hello, World!"
print(s[-1]) # 输出: !
print(s[-5]) # 输出: W
二、使用切片
切片允许我们获取字符串的子字符串。切片的语法是 s[start:end:step]
,其中 start
是起始索引,end
是结束索引(不包括),step
是步长。
1、基础切片
s = "Hello, World!"
print(s[0:5]) # 输出: Hello
print(s[7:12]) # 输出: World
2、带步长的切片
s = "Hello, World!"
print(s[::2]) # 输出: Hlo ol!
print(s[1::2]) # 输出: el,Wrd
3、负步长切片(反转字符串)
s = "Hello, World!"
print(s[::-1]) # 输出: !dlroW ,olleH
三、字符串方法
Python提供了许多内置的字符串方法,可以方便地操作和提取字符串。
1、split()
split()
方法根据分隔符将字符串拆分成列表。
s = "Hello, World!"
print(s.split(',')) # 输出: ['Hello', ' World!']
2、join()
join()
方法用于将序列中的元素以指定的字符连接生成一个新的字符串。
words = ['Hello', 'World']
print(' '.join(words)) # 输出: Hello World
3、find()
find()
方法返回子字符串在字符串中首次出现的位置,如果没有找到子字符串则返回-1。
s = "Hello, World!"
print(s.find('World')) # 输出: 7
print(s.find('Python')) # 输出: -1
4、replace()
replace()
方法返回一个新的字符串,其中所有匹配的子字符串都被替换。
s = "Hello, World!"
print(s.replace('World', 'Python')) # 输出: Hello, Python!
四、正则表达式
正则表达式是一种强大的字符串匹配和提取工具。在Python中,可以使用 re
模块来处理正则表达式。
1、re.findall()
re.findall()
方法返回一个列表,包含所有与正则表达式匹配的子字符串。
import re
s = "The rain in Spain falls mainly in the plain."
matches = re.findall(r'\bin\b', s)
print(matches) # 输出: ['in', 'in']
2、re.search()
re.search()
方法返回第一个与正则表达式匹配的对象,如果没有匹配则返回 None
。
import re
s = "The rain in Spain falls mainly in the plain."
match = re.search(r'Spain', s)
if match:
print("Found:", match.group()) # 输出: Found: Spain
3、re.sub()
re.sub()
方法用于替换匹配的子字符串。
import re
s = "The rain in Spain falls mainly in the plain."
new_s = re.sub(r'Spain', 'Italy', s)
print(new_s) # 输出: The rain in Italy falls mainly in the plain.
五、实战案例
1、提取电子邮件地址
假设我们有一段文本,其中包含多个电子邮件地址,我们需要提取所有的电子邮件地址。
import re
text = """
Please contact us at support@example.com for further information.
You can also reach out to sales@example.com or feedback@example.org.
"""
emails = re.findall(r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b', text)
print(emails) # 输出: ['support@example.com', 'sales@example.com', 'feedback@example.org']
2、提取URL
假设我们有一段HTML代码,需要提取其中所有的URL。
import re
html = """
<a href="http://example.com">Example</a>
<a href="https://www.example.org">Example Org</a>
<a href="ftp://files.example.net">Example FTP</a>
"""
urls = re.findall(r'href="(.*?)"', html)
print(urls) # 输出: ['http://example.com', 'https://www.example.org', 'ftp://files.example.net']
3、提取日期
假设我们有一段文本,其中包含多个日期,我们需要提取所有的日期。
import re
text = """
The event will be held on 2023-05-15. Another event is scheduled for 2023-06-20.
Please mark your calendar for 2023-07-25.
"""
dates = re.findall(r'\b\d{4}-\d{2}-\d{2}\b', text)
print(dates) # 输出: ['2023-05-15', '2023-06-20', '2023-07-25']
通过上述方法,我们可以灵活地在Python中提取字符串。这些方法各有优缺点,选择哪种方法取决于具体的应用场景。在实际开发中,合理组合使用这些方法,可以大大提高字符串处理的效率。希望这篇文章能帮助你更好地理解和掌握Python中提取字符串的方法。
相关问答FAQs:
如何在Python中提取字符串的特定部分?
在Python中,可以使用切片操作来提取字符串的特定部分。通过指定起始和结束索引,可以轻松获取所需的子字符串。例如,my_string[1:5]
将提取从索引1到索引4的部分。使用负索引也可以从字符串的末尾开始提取。
Python中有哪些方法可以查找字符串?
Python提供多种方法来查找字符串中的特定内容。常用的方法包括find()
、index()
和count()
。find()
返回子字符串首次出现的索引,如果未找到,则返回-1;而index()
在未找到时会引发异常。count()
可以用于统计子字符串在原字符串中出现的次数。
如何使用正则表达式提取字符串中的特定模式?
使用Python的re
模块,可以通过正则表达式提取字符串中符合特定模式的内容。例如,使用re.findall()
可以找到所有匹配的子串,并将其以列表形式返回。正则表达式提供了强大的模式匹配能力,能够处理复杂的字符串提取需求。
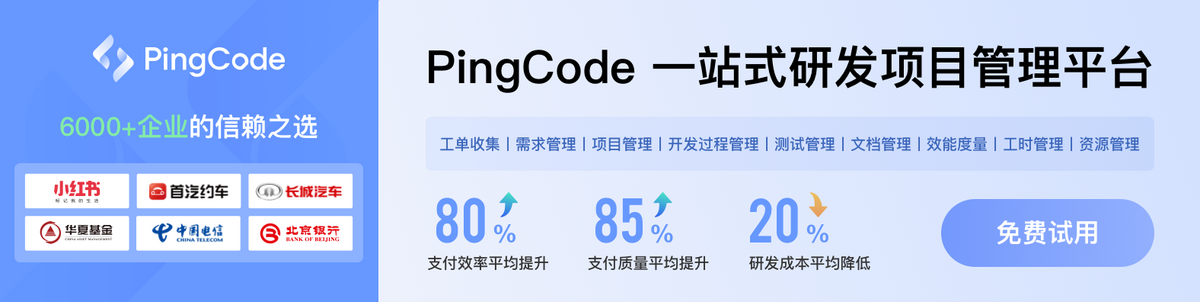