Python可以实现办公自动化的方式包括:自动化数据处理、自动化文件管理、自动化邮件处理、自动化网络爬虫等。 其中,自动化数据处理是最常见和最有用的一种方式。例如,通过Python编写脚本,可以自动从电子表格中提取数据,进行数据清洗和分析,然后将结果生成新的报告。这不仅提高了工作效率,还减少了人为错误。
一、自动化数据处理
自动化数据处理是指利用Python脚本自动完成数据的收集、清洗、分析和可视化等一系列工作。Python的pandas、numpy等库使得数据处理变得非常简单和高效。例如,我们可以使用pandas从Excel文件中读取数据,进行数据清洗和转换,然后使用matplotlib或seaborn库生成图表。
import pandas as pd
import matplotlib.pyplot as plt
读取Excel文件
df = pd.read_excel('data.xlsx')
数据清洗
df.dropna(inplace=True)
数据分析
summary = df.describe()
数据可视化
plt.figure(figsize=(10, 6))
plt.plot(df['Date'], df['Sales'])
plt.title('Sales Over Time')
plt.xlabel('Date')
plt.ylabel('Sales')
plt.show()
二、自动化文件管理
自动化文件管理是指使用Python脚本自动完成文件的创建、删除、重命名、移动等操作。os和shutil库是实现这一功能的主要工具。例如,可以编写脚本自动整理下载文件夹中的文件,将不同类型的文件移动到对应的文件夹中。
import os
import shutil
定义文件夹路径
download_folder = 'C:/Users/YourName/Downloads'
image_folder = 'C:/Users/YourName/Pictures'
doc_folder = 'C:/Users/YourName/Documents'
获取文件列表
files = os.listdir(download_folder)
移动文件
for file in files:
if file.endswith('.jpg') or file.endswith('.png'):
shutil.move(os.path.join(download_folder, file), image_folder)
elif file.endswith('.docx') or file.endswith('.pdf'):
shutil.move(os.path.join(download_folder, file), doc_folder)
三、自动化邮件处理
自动化邮件处理是指使用Python脚本自动发送、接收和处理电子邮件。smtplib和imaplib是实现这一功能的主要库。例如,可以编写脚本定期检查邮箱中的新邮件,并根据邮件内容执行相应的操作,如自动回复或将邮件内容保存到数据库中。
import smtplib
import imaplib
import email
发送邮件
def send_email(subject, body, to_email):
from_email = 'your_email@example.com'
password = 'your_password'
msg = email.message.EmailMessage()
msg.set_content(body)
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
with smtplib.SMTP_SSL('smtp.example.com', 465) as server:
server.login(from_email, password)
server.send_message(msg)
接收邮件
def check_inbox():
email_user = 'your_email@example.com'
email_pass = 'your_password'
mail = imaplib.IMAP4_SSL('imap.example.com')
mail.login(email_user, email_pass)
mail.select('inbox')
status, data = mail.search(None, 'ALL')
mail_ids = data[0].split()
for mail_id in mail_ids:
status, msg_data = mail.fetch(mail_id, '(RFC822)')
msg = email.message_from_bytes(msg_data[0][1])
print('From:', msg['From'])
print('Subject:', msg['Subject'])
print('Body:', msg.get_payload(decode=True).decode())
四、自动化网络爬虫
自动化网络爬虫是指使用Python脚本自动抓取网页数据。BeautifulSoup和Scrapy是实现这一功能的主要库。例如,可以编写脚本定期抓取某个网站的新闻文章,并将文章内容保存到本地文件或数据库中。
import requests
from bs4 import BeautifulSoup
定义目标网站
url = 'https://example.com/news'
发送请求
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
解析网页内容
articles = soup.find_all('article')
for article in articles:
title = article.find('h2').get_text()
content = article.find('p').get_text()
# 保存文章内容
with open(f'{title}.txt', 'w', encoding='utf-8') as file:
file.write(content)
五、自动化报告生成
自动化报告生成是指使用Python脚本自动生成PDF或Word格式的报告。reportlab和python-docx是实现这一功能的主要库。例如,可以编写脚本从数据库中提取数据,进行分析和可视化,然后将结果生成PDF报告。
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
创建PDF文档
pdf_file = 'report.pdf'
pdf = canvas.Canvas(pdf_file, pagesize=letter)
pdf.setTitle('Monthly Report')
添加内容
pdf.drawString(100, 750, 'Monthly Sales Report')
pdf.drawString(100, 730, 'Total Sales: $10000')
保存PDF文档
pdf.save()
六、自动化办公流程
自动化办公流程是指使用Python脚本自动化完成一系列办公任务。例如,可以编写脚本自动从ERP系统中提取数据,生成报告,并通过邮件发送给相关人员。这可以大大提高办公效率,减少人为错误。
import pandas as pd
import smtplib
import email
从ERP系统中提取数据(假设数据已保存为CSV文件)
df = pd.read_csv('erp_data.csv')
生成报告
report = df.describe().to_string()
发送报告邮件
def send_email(subject, body, to_email):
from_email = 'your_email@example.com'
password = 'your_password'
msg = email.message.EmailMessage()
msg.set_content(body)
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
with smtplib.SMTP_SSL('smtp.example.com', 465) as server:
server.login(from_email, password)
server.send_message(msg)
send_email('Monthly Report', report, 'manager@example.com')
七、自动化日程管理
自动化日程管理是指使用Python脚本自动管理日程安排。例如,可以编写脚本自动从Google Calendar中获取日程信息,并根据日程自动发送提醒邮件或生成提醒通知。
from googleapiclient.discovery import build
from google.oauth2.credentials import Credentials
获取Google Calendar日程信息
def get_calendar_events():
creds = Credentials.from_authorized_user_file('credentials.json')
service = build('calendar', 'v3', credentials=creds)
events_result = service.events().list(calendarId='primary', maxResults=10, singleEvents=True, orderBy='startTime').execute()
events = events_result.get('items', [])
return events
发送提醒邮件
def send_reminder_email(event):
from_email = 'your_email@example.com'
password = 'your_password'
subject = f'Reminder: {event["summary"]}'
body = f'Don\'t forget your event: {event["summary"]} at {event["start"]["dateTime"]}'
to_email = 'your_email@example.com'
msg = email.message.EmailMessage()
msg.set_content(body)
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
with smtplib.SMTP_SSL('smtp.example.com', 465) as server:
server.login(from_email, password)
server.send_message(msg)
events = get_calendar_events()
for event in events:
send_reminder_email(event)
总之,Python可以通过编写脚本实现各种办公自动化任务,从而大大提高办公效率,减少人为错误。这不仅可以节省时间和精力,还可以使办公流程更加规范和高效。
相关问答FAQs:
如何使用Python进行办公自动化?
Python是一个非常灵活且强大的编程语言,它提供了多种库和工具来实现办公自动化。通过使用如Pandas、OpenPyXL和Selenium等库,用户可以轻松处理数据、自动化生成报告、与电子表格交互以及进行网页抓取等任务。这些工具能够帮助企业提高工作效率,减少人为错误,从而优化办公流程。
Python办公自动化的常见应用场景有哪些?
在实际应用中,Python可以用于自动化生成和发送电子邮件、定期更新数据库、批量处理文件、分析数据并生成可视化报告等。通过这些自动化任务,员工可以将更多时间集中在核心业务上,而不是重复的手动操作。
我需要什么技能才能开始使用Python进行办公自动化?
要有效地使用Python进行办公自动化,用户应具备基本的编程知识,了解数据结构和常用算法。此外,熟悉相关库的使用和API的调用将有助于实现更复杂的自动化任务。通过在线课程和实践项目,用户可以逐步提升自己的技能水平,掌握办公自动化的要领。
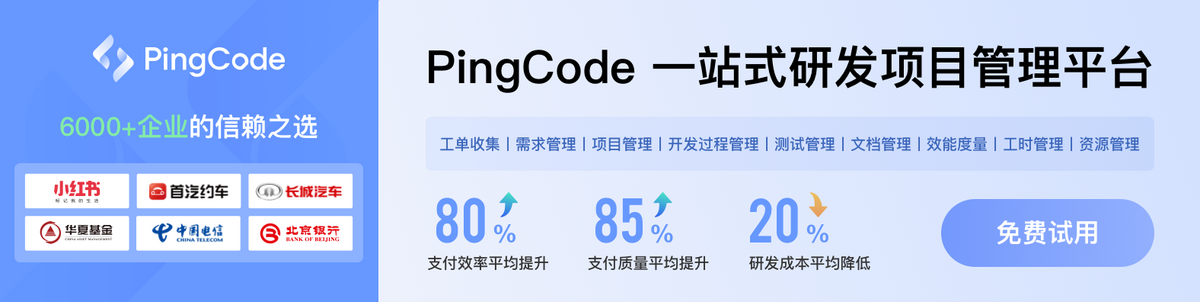