编写Python程序打印价格列表,可以使用Python中的各种数据结构和格式化输出功能。下面是一个基本的例子,通过创建一个包含产品和价格的字典,然后使用循环和格式化字符串打印出价格列表。
一、创建产品和价格的数据结构
首先,我们需要一个数据结构来存储产品和它们的价格。字典是一个很好的选择,因为它允许我们将产品名称(键)与价格(值)配对。
products = {
'Apple': 0.50,
'Banana': 0.30,
'Orange': 0.80,
'Grapes': 2.50,
'Mango': 1.20
}
二、格式化和打印价格列表
Python提供了多种方法来格式化字符串,最常见的包括使用printf
风格的格式化、str.format
方法和f字符串(也称为格式化字符串字面值)。
使用printf
风格的格式化
for product, price in products.items():
print("%-10s : $%.2f" % (product, price))
使用str.format
方法
for product, price in products.items():
print("{:<10} : ${:.2f}".format(product, price))
使用f字符串
for product, price in products.items():
print(f"{product:<10} : ${price:.2f}")
三、添加总计
要添加一个总计,可以在循环结束后计算并打印总计。
total = sum(products.values())
print("\nTotal: ${:.2f}".format(total))
或者使用f字符串:
print(f"\nTotal: ${total:.2f}")
四、示例完整代码
下面是一个完整的示例代码,将上述所有步骤结合在一起:
# 定义产品及其价格的字典
products = {
'Apple': 0.50,
'Banana': 0.30,
'Orange': 0.80,
'Grapes': 2.50,
'Mango': 1.20
}
打印标题
print("{:<10} : {}".format("Product", "Price"))
print("-" * 20)
打印产品和价格
for product, price in products.items():
print(f"{product:<10} : ${price:.2f}")
计算并打印总计
total = sum(products.values())
print("-" * 20)
print(f"Total : ${total:.2f}")
这个程序首先定义一个包含产品和价格的字典,然后使用循环和格式化字符串打印每个产品的价格,最后计算并打印总计。
五、添加更多功能
根据需求,可以进一步扩展这个程序。例如,可以从用户输入读取产品和价格,或将价格列表保存到文件中。以下是一些扩展的示例:
从用户输入读取产品和价格
products = {}
while True:
product = input("Enter product name (or 'done' to finish): ")
if product.lower() == 'done':
break
price = float(input(f"Enter price for {product}: "))
products[product] = price
打印价格列表和总计
print("\nPrice List:")
print("{:<10} : {}".format("Product", "Price"))
print("-" * 20)
for product, price in products.items():
print(f"{product:<10} : ${price:.2f}")
total = sum(products.values())
print("-" * 20)
print(f"Total : ${total:.2f}")
将价格列表保存到文件
# 定义产品及其价格的字典
products = {
'Apple': 0.50,
'Banana': 0.30,
'Orange': 0.80,
'Grapes': 2.50,
'Mango': 1.20
}
保存到文件
with open("price_list.txt", "w") as file:
file.write("{:<10} : {}\n".format("Product", "Price"))
file.write("-" * 20 + "\n")
for product, price in products.items():
file.write(f"{product:<10} : ${price:.2f}\n")
total = sum(products.values())
file.write("-" * 20 + "\n")
file.write(f"Total : ${total:.2f}\n")
通过以上扩展,可以使程序更加灵活和实用。无论是读取用户输入还是将数据保存到文件,这些都是常见的需求,能够进一步提高程序的实用性。希望这些示例对你编写Python程序打印价格列表有所帮助。
相关问答FAQs:
如何在Python中创建一个价格列表并打印出来?
要创建一个价格列表,可以使用Python的列表数据结构来存储价格。示例代码如下:
prices = [19.99, 29.99, 9.99, 5.49] # 定义价格列表
for price in prices: # 遍历价格列表
print(f"${price:.2f}") # 格式化打印价格
这段代码会输出格式化的价格,确保每个价格都有两位小数。
如何对价格列表进行排序并打印排序后的结果?
可以使用Python内置的sorted()
函数对价格列表进行排序。示例如下:
prices = [19.99, 29.99, 9.99, 5.49]
sorted_prices = sorted(prices) # 对价格进行排序
for price in sorted_prices:
print(f"${price:.2f}")
这样,可以得到从低到高的价格列表,便于分析和比较。
如何在打印价格时添加货币符号?
在打印价格时,可以通过字符串格式化轻松添加货币符号。以下是示例代码:
prices = [19.99, 29.99, 9.99, 5.49]
for price in prices:
print(f"Price: ${price:.2f}") # 添加货币符号并格式化
这样的输出会让价格更加清晰易懂,尤其在商业和电商场景中。
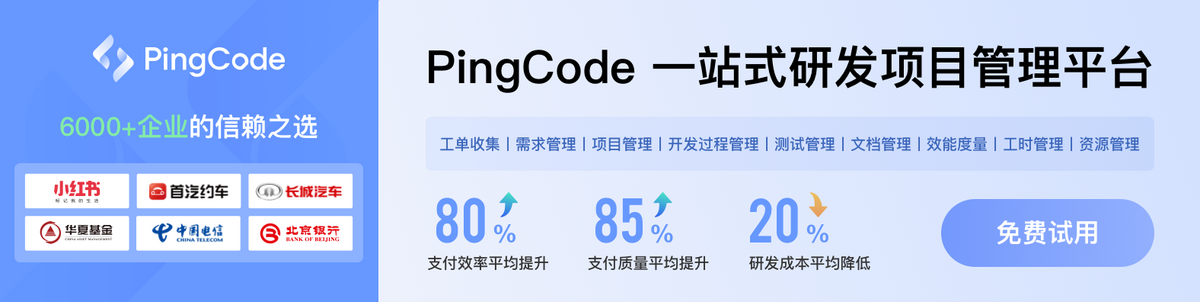