在Python中编制五星红旗,可以通过以下几个步骤:使用matplotlib绘图库绘制、定义五角星的绘制函数、设置坐标和颜色、组合图形。 其中,最重要的是如何使用matplotlib进行图形绘制,并且准确地定义五角星的形状和位置。
一、使用Matplotlib绘图库绘制
Matplotlib是Python中常用的绘图库,能够绘制各种图形和图表。首先,我们需要安装matplotlib库,然后导入相关的模块。
import matplotlib.pyplot as plt
import numpy as np
二、定义五角星的绘制函数
为了绘制五角星,我们需要定义一个函数来生成五角星的顶点坐标。五角星有五个顶点,每个顶点之间的角度为72度。
def draw_star(ax, center, radius, color):
x, y = center
angles = np.linspace(0, 2 * np.pi, 6)[:-1] # 0 to 2pi, but only 5 points
points = np.array([(x + np.cos(angle) * radius, y + np.sin(angle) * radius) for angle in angles])
star = plt.Polygon(points, closed=True, color=color)
ax.add_patch(star)
三、设置坐标和颜色
五星红旗的颜色主要是红色和黄色。我们需要设置红色背景,并在合适的位置绘制黄色的五角星。
def draw_flag():
fig, ax = plt.subplots()
ax.set_xlim(0, 30)
ax.set_ylim(0, 20)
ax.set_aspect('equal')
# 绘制红色背景
ax.add_patch(plt.Rectangle((0, 0), 30, 20, color='red'))
# 绘制大五角星
draw_star(ax, (5, 15), 3, 'yellow')
# 绘制小五角星
small_star_centers = [(10, 18), (12, 16), (12, 13), (10, 11)]
for center in small_star_centers:
draw_star(ax, center, 1, 'yellow')
plt.axis('off')
plt.show()
draw_flag()
四、组合图形
通过以上步骤,我们已经可以绘制出五星红旗的基本形状和颜色。接下来,我们需要将这些图形组合起来,确保每个元素的位置和大小都符合规范。
def rotate_point(origin, point, angle):
ox, oy = origin
px, py = point
qx = ox + np.cos(angle) * (px - ox) - np.sin(angle) * (py - oy)
qy = oy + np.sin(angle) * (px - ox) + np.cos(angle) * (py - oy)
return qx, qy
def draw_star(ax, center, radius, color, angle=0):
x, y = center
angles = np.linspace(0, 2 * np.pi, 6)[:-1] # 0 to 2pi, but only 5 points
points = np.array([(x + np.cos(angle) * radius, y + np.sin(angle) * radius) for angle in angles])
points = [rotate_point(center, point, angle) for point in points]
star = plt.Polygon(points, closed=True, color=color)
ax.add_patch(star)
def draw_flag():
fig, ax = plt.subplots()
ax.set_xlim(0, 30)
ax.set_ylim(0, 20)
ax.set_aspect('equal')
# 绘制红色背景
ax.add_patch(plt.Rectangle((0, 0), 30, 20, color='red'))
# 绘制大五角星
draw_star(ax, (5, 15), 3, 'yellow')
# 绘制小五角星
small_star_centers = [(10, 18), (12, 16), (12, 13), (10, 11)]
for center in small_star_centers:
draw_star(ax, center, 1, 'yellow', angle=np.pi/5)
plt.axis('off')
plt.show()
draw_flag()
五、总结
通过以上步骤,我们可以使用Python的matplotlib库绘制出标准的五星红旗。在绘制过程中,我们需要注意以下几点:
- 使用matplotlib库进行图形绘制,包括设置背景颜色和绘制多边形;
- 定义五角星的顶点坐标,并使用多边形函数绘制五角星;
- 设置五角星的颜色和大小,并在合适的位置绘制;
- 组合图形,确保每个元素的位置和大小都符合规范。
通过这些步骤,我们可以在Python中轻松地绘制出五星红旗,为图形绘制提供了一个实际的应用案例。
相关问答FAQs:
如何使用Python绘制五星红旗?
可以使用Python的图形库,如Matplotlib或Turtle,来绘制五星红旗。通过设置背景颜色为红色,并在适当的位置绘制五颗黄色星星,就能完成这一任务。具体步骤包括创建画布、填充颜色以及添加星星的绘制函数。
绘制五星红旗需要哪些Python库?
通常推荐使用Matplotlib或Turtle库。Matplotlib适合于生成高质量的图形和图像,而Turtle库则更适合初学者,因为其图形化操作更直观,容易上手。可以根据自己的需求选择合适的库进行绘制。
在编写代码时有哪些注意事项?
确保选择合适的坐标系和比例,以准确地呈现五星红旗的各个元素。此外,注意颜色的准确性,红色背景和黄色星星应该符合实际国旗的标准。代码的可读性也很重要,适当添加注释能帮助他人理解你的代码逻辑。
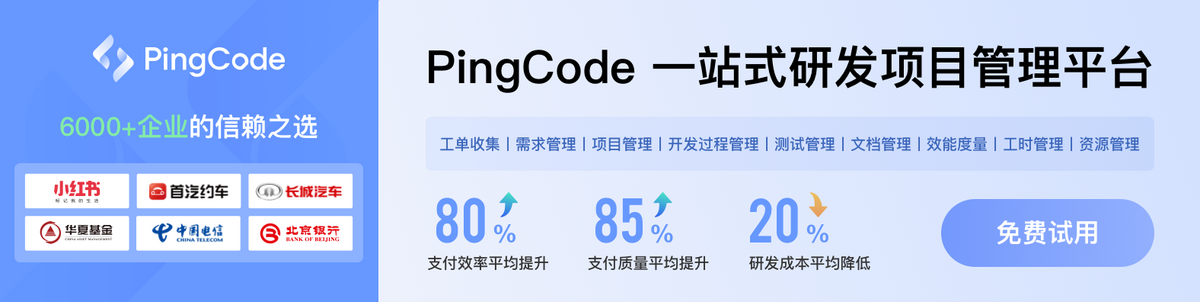