在Python中写和读文件路径的方式有很多种,主要包括:使用原生文件处理函数、使用os模块、使用pathlib模块等。这些方法各有优劣,可以根据具体情况选择使用。以下将详细介绍几种常用方法,并给出示例代码。
一、使用原生文件处理函数
在Python中,最简单的方式是使用内置的open()函数来读取文件。对于文件路径的处理,可以直接在路径前加上引号,注意路径中的反斜杠需要双写。
# 写文件
with open('path/to/your/file.txt', 'w') as file:
file.write('This is a test.')
读文件
with open('path/to/your/file.txt', 'r') as file:
content = file.read()
print(content)
二、使用os模块
os模块提供了丰富的文件和目录操作功能,其中os.path模块专门处理路径问题,可以更加灵活和安全地操作文件路径。
import os
写文件
file_path = os.path.join('path', 'to', 'your', 'file.txt')
with open(file_path, 'w') as file:
file.write('This is a test.')
读文件
with open(file_path, 'r') as file:
content = file.read()
print(content)
使用os.path.join()可以避免手动拼接路径时容易出错的问题,比如忘记加斜杠或者斜杠方向错误。
三、使用pathlib模块
pathlib模块是Python 3.4引入的新模块,用于处理文件路径,提供了面向对象的路径操作方式,更加直观和便捷。
from pathlib import Path
写文件
file_path = Path('path/to/your/file.txt')
file_path.write_text('This is a test.')
读文件
content = file_path.read_text()
print(content)
pathlib模块不仅提供了更加简洁的语法,还支持跨平台路径处理,非常适合处理复杂的文件路径操作。
四、处理相对路径和绝对路径
在文件操作中,经常需要处理相对路径和绝对路径,可以使用os.path.abspath()函数将相对路径转换为绝对路径,确保文件操作的准确性。
import os
相对路径
relative_path = 'path/to/your/file.txt'
转换为绝对路径
absolute_path = os.path.abspath(relative_path)
print(f'Absolute path: {absolute_path}')
写文件
with open(absolute_path, 'w') as file:
file.write('This is a test.')
读文件
with open(absolute_path, 'r') as file:
content = file.read()
print(content)
五、处理文件路径中的特殊字符
在文件路径中,可能会遇到一些特殊字符,如空格、中文字符等,需要进行适当的编码处理。可以使用urllib.parse模块对路径进行编码和解码。
from urllib.parse import quote, unquote
原始路径
file_path = 'path/to/your/file with spaces.txt'
编码路径
encoded_path = quote(file_path)
print(f'Encoded path: {encoded_path}')
解码路径
decoded_path = unquote(encoded_path)
print(f'Decoded path: {decoded_path}')
写文件
with open(decoded_path, 'w') as file:
file.write('This is a test.')
读文件
with open(decoded_path, 'r') as file:
content = file.read()
print(content)
通过编码处理,可以确保路径中的特殊字符不会导致文件操作出错。
六、处理文件路径中的环境变量
在某些情况下,文件路径中可能包含环境变量,需要使用os模块对环境变量进行替换。
import os
原始路径
file_path = '$HOME/path/to/your/file.txt'
替换环境变量
expanded_path = os.path.expandvars(file_path)
print(f'Expanded path: {expanded_path}')
写文件
with open(expanded_path, 'w') as file:
file.write('This is a test.')
读文件
with open(expanded_path, 'r') as file:
content = file.read()
print(content)
通过环境变量替换,可以确保文件路径的灵活性和可移植性。
七、处理文件路径中的用户目录
在文件路径中,可能需要处理用户目录(如home目录),可以使用os.path.expanduser()函数将波浪号(~)转换为实际的用户目录。
import os
原始路径
file_path = '~/path/to/your/file.txt'
替换用户目录
expanded_path = os.path.expanduser(file_path)
print(f'Expanded path: {expanded_path}')
写文件
with open(expanded_path, 'w') as file:
file.write('This is a test.')
读文件
with open(expanded_path, 'r') as file:
content = file.read()
print(content)
通过用户目录替换,可以确保文件操作的灵活性和用户体验。
八、处理文件路径中的父目录和当前目录
在文件路径中,可能需要处理父目录(..)和当前目录(.),可以使用os.path.abspath()函数将相对路径转换为绝对路径,确保文件操作的准确性。
import os
原始路径
file_path = '../path/to/your/file.txt'
转换为绝对路径
absolute_path = os.path.abspath(file_path)
print(f'Absolute path: {absolute_path}')
写文件
with open(absolute_path, 'w') as file:
file.write('This is a test.')
读文件
with open(absolute_path, 'r') as file:
content = file.read()
print(content)
九、处理文件路径中的文件名和扩展名
在文件操作中,可能需要处理文件名和扩展名,可以使用os.path.splitext()函数将文件名和扩展名分开,方便后续操作。
import os
原始路径
file_path = 'path/to/your/file.txt'
分离文件名和扩展名
file_name, file_ext = os.path.splitext(file_path)
print(f'File name: {file_name}')
print(f'File extension: {file_ext}')
修改文件名
new_file_path = file_name + '_new' + file_ext
print(f'New file path: {new_file_path}')
写文件
with open(new_file_path, 'w') as file:
file.write('This is a test.')
读文件
with open(new_file_path, 'r') as file:
content = file.read()
print(content)
通过分离文件名和扩展名,可以方便地对文件名进行修改,满足不同的需求。
十、处理文件路径中的目录
在文件操作中,可能需要处理目录,可以使用os.makedirs()函数创建目录,确保文件路径的存在性。
import os
原始路径
file_path = 'path/to/your/file.txt'
提取目录路径
dir_path = os.path.dirname(file_path)
print(f'Directory path: {dir_path}')
创建目录
os.makedirs(dir_path, exist_ok=True)
写文件
with open(file_path, 'w') as file:
file.write('This is a test.')
读文件
with open(file_path, 'r') as file:
content = file.read()
print(content)
通过创建目录,可以确保文件路径的存在性,避免文件操作出错。
总结
在Python中写和读文件路径的方法多种多样,可以根据具体情况选择合适的方法。使用原生文件处理函数、os模块和pathlib模块是最常用的方法,可以满足大多数文件操作需求。在处理文件路径时,还需要注意相对路径和绝对路径、特殊字符、环境变量、用户目录、父目录和当前目录、文件名和扩展名、目录等问题,确保文件操作的准确性和灵活性。
相关问答FAQs:
在Python中,如何正确使用相对路径和绝对路径读取文件?
在Python中,可以使用相对路径和绝对路径来读取文件。相对路径是相对于当前工作目录的路径,而绝对路径是文件在文件系统中的完整路径。使用os
模块可以方便地获取当前工作目录。示例代码如下:
import os
# 获取当前工作目录
current_directory = os.getcwd()
# 使用相对路径
with open('data/file.txt', 'r') as file:
content = file.read()
# 使用绝对路径
absolute_path = os.path.join(current_directory, 'data', 'file.txt')
with open(absolute_path, 'r') as file:
content = file.read()
这样可以确保无论在哪个目录下运行代码,都能正确读取文件。
在Python中读取文件时,如何处理文件不存在的情况?
当尝试读取一个不存在的文件时,Python会抛出FileNotFoundError
异常。为了优雅地处理这种情况,可以使用try-except
块。示例代码如下:
try:
with open('data/file.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("文件未找到,请检查路径是否正确。")
这种方式可以避免程序崩溃,并提供友好的错误提示。
Python中如何以不同模式读取文件,例如只读、写入和追加?
在Python中,打开文件时可以使用不同的模式来控制文件的读取和写入方式。常用的模式包括:
'r'
:只读模式,文件必须存在。'w'
:写入模式,如果文件存在将被覆盖。'a'
:追加模式,在文件末尾添加内容。
示例代码如下:
# 只读
with open('data/file.txt', 'r') as file:
content = file.read()
# 写入
with open('data/file.txt', 'w') as file:
file.write("写入新的内容。")
# 追加
with open('data/file.txt', 'a') as file:
file.write("追加的内容。")
选择合适的模式可以确保文件操作的正确性与效率。
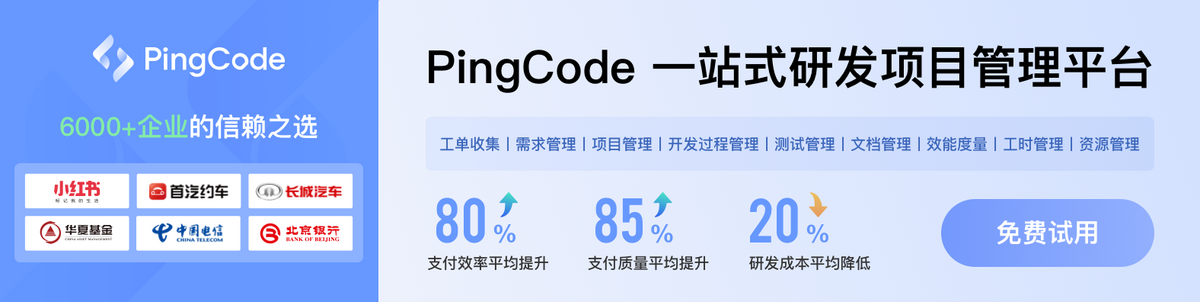