Python下载网上图片格式的方法有:使用requests库、使用urllib库、使用PIL库、使用BeautifulSoup库。下面将详细介绍其中的一个方法,即使用requests库下载图片。
使用requests库下载图片是最常见、也是最简单的方法之一。requests库是一个简单易用的HTTP请求库,可以用来下载网页内容,包括图片。通过requests库,可以轻松地发送HTTP请求并获取响应,然后将响应内容保存为图片文件。下面是具体的步骤:
- 安装requests库:首先需要安装requests库,可以使用pip进行安装。命令如下:
pip install requests
- 导入requests库:在Python脚本中导入requests库。
import requests
- 发送HTTP请求:使用requests库发送HTTP GET请求,获取图片的响应内容。
response = requests.get('图片URL')
- 保存图片文件:将响应内容保存为图片文件,可以使用二进制写入模式将响应内容写入文件。
with open('图片保存路径', 'wb') as file:
file.write(response.content)
以下是完整的示例代码:
import requests
图片URL
image_url = 'https://example.com/image.jpg'
发送HTTP GET请求
response = requests.get(image_url)
保存图片文件
with open('downloaded_image.jpg', 'wb') as file:
file.write(response.content)
print("图片下载完成")
一、安装和导入requests库
在开始下载图片之前,首先需要确保已经安装了requests库。如果未安装requests库,可以使用以下命令进行安装:
pip install requests
安装完成后,在Python脚本中导入requests库:
import requests
二、发送HTTP请求获取图片
使用requests库发送HTTP GET请求,获取图片的响应内容。这里需要指定图片的URL,然后使用requests.get()方法发送请求,并获取响应对象。
image_url = 'https://example.com/image.jpg'
response = requests.get(image_url)
三、检查响应状态码
在保存图片之前,可以先检查响应的状态码,以确保请求成功。通常状态码为200表示请求成功。
if response.status_code == 200:
# 请求成功
pass
else:
# 请求失败
print(f"请求失败,状态码:{response.status_code}")
四、保存图片文件
将响应内容保存为图片文件,可以使用二进制写入模式将响应内容写入文件。这里使用with open()语句,可以确保文件在写入完成后自动关闭。
if response.status_code == 200:
with open('downloaded_image.jpg', 'wb') as file:
file.write(response.content)
print("图片下载完成")
else:
print(f"请求失败,状态码:{response.status_code}")
五、处理异常情况
在实际应用中,还需要考虑到可能出现的异常情况,例如网络连接错误、请求超时等。可以使用try-except语句进行异常处理。
try:
response = requests.get(image_url)
response.raise_for_status() # 检查请求是否成功
with open('downloaded_image.jpg', 'wb') as file:
file.write(response.content)
print("图片下载完成")
except requests.RequestException as e:
print(f"请求出现错误:{e}")
六、使用urllib库下载图片
除了requests库,还可以使用Python内置的urllib库来下载图片。urllib库提供了处理URL的功能,可以用来发送HTTP请求并获取响应内容。
- 导入urllib库:在Python脚本中导入urllib.request模块。
import urllib.request
- 发送HTTP请求:使用urllib.request.urlopen()方法发送HTTP GET请求,获取图片的响应内容。
response = urllib.request.urlopen('图片URL')
- 保存图片文件:将响应内容保存为图片文件,可以使用二进制写入模式将响应内容写入文件。
with open('图片保存路径', 'wb') as file:
file.write(response.read())
以下是完整的示例代码:
import urllib.request
图片URL
image_url = 'https://example.com/image.jpg'
发送HTTP GET请求
response = urllib.request.urlopen(image_url)
保存图片文件
with open('downloaded_image.jpg', 'wb') as file:
file.write(response.read())
print("图片下载完成")
七、使用PIL库处理图片
PIL(Python Imaging Library)是一个强大的图像处理库,可以用来处理和操作图片。PIL的一个分支是Pillow,Pillow是一个更加现代化、易用的版本。
- 安装Pillow库:首先需要安装Pillow库,可以使用pip进行安装。命令如下:
pip install pillow
- 导入Pillow库:在Python脚本中导入Pillow库中的Image模块。
from PIL import Image
import requests
from io import BytesIO
- 发送HTTP请求:使用requests库发送HTTP GET请求,获取图片的响应内容。
response = requests.get('图片URL')
- 打开图片:使用BytesIO将响应内容转为二进制流,然后使用Pillow库的Image模块打开图片。
image = Image.open(BytesIO(response.content))
- 保存图片文件:使用Image模块的save()方法将图片保存为文件。
image.save('图片保存路径')
以下是完整的示例代码:
from PIL import Image
import requests
from io import BytesIO
图片URL
image_url = 'https://example.com/image.jpg'
发送HTTP GET请求
response = requests.get(image_url)
打开图片
image = Image.open(BytesIO(response.content))
保存图片文件
image.save('downloaded_image.jpg')
print("图片下载完成")
八、使用BeautifulSoup库解析网页获取图片URL
在某些情况下,图片URL可能嵌入在网页中,需要先解析网页内容,提取出图片URL。BeautifulSoup是一个用于解析HTML和XML的库,可以用来提取网页中的图片URL。
- 安装BeautifulSoup库:首先需要安装BeautifulSoup库,可以使用pip进行安装。命令如下:
pip install beautifulsoup4
- 导入BeautifulSoup库:在Python脚本中导入BeautifulSoup库和requests库。
from bs4 import BeautifulSoup
import requests
- 发送HTTP请求:使用requests库发送HTTP GET请求,获取网页的响应内容。
response = requests.get('网页URL')
- 解析网页内容:使用BeautifulSoup解析网页内容,提取出图片URL。
soup = BeautifulSoup(response.text, 'html.parser')
image_url = soup.find('img')['src']
- 下载图片:使用requests库下载提取出的图片URL。
image_response = requests.get(image_url)
with open('downloaded_image.jpg', 'wb') as file:
file.write(image_response.content)
以下是完整的示例代码:
from bs4 import BeautifulSoup
import requests
网页URL
page_url = 'https://example.com/page.html'
发送HTTP GET请求
response = requests.get(page_url)
解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
image_url = soup.find('img')['src']
下载图片
image_response = requests.get(image_url)
with open('downloaded_image.jpg', 'wb') as file:
file.write(image_response.content)
print("图片下载完成")
九、使用多线程下载图片
在下载大量图片时,可以使用多线程加快下载速度。Python的threading库可以用来实现多线程下载。
- 导入threading库:在Python脚本中导入threading库和requests库。
import threading
import requests
- 定义下载函数:定义一个下载函数,用于下载图片。
def download_image(image_url, save_path):
response = requests.get(image_url)
with open(save_path, 'wb') as file:
file.write(response.content)
print(f"{save_path} 下载完成")
- 创建线程并启动:创建多个线程,每个线程下载一张图片。
image_urls = ['https://example.com/image1.jpg', 'https://example.com/image2.jpg']
threads = []
for i, image_url in enumerate(image_urls):
save_path = f'downloaded_image_{i+1}.jpg'
thread = threading.Thread(target=download_image, args=(image_url, save_path))
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
以下是完整的示例代码:
import threading
import requests
def download_image(image_url, save_path):
response = requests.get(image_url)
with open(save_path, 'wb') as file:
file.write(response.content)
print(f"{save_path} 下载完成")
image_urls = ['https://example.com/image1.jpg', 'https://example.com/image2.jpg']
threads = []
for i, image_url in enumerate(image_urls):
save_path = f'downloaded_image_{i+1}.jpg'
thread = threading.Thread(target=download_image, args=(image_url, save_path))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
十、总结
通过上述方法,可以使用Python轻松实现下载网上图片的功能。requests库是最常见和易用的选择,可以通过简单的HTTP请求获取图片内容并保存。对于需要解析网页获取图片URL的情况,可以结合BeautifulSoup库进行处理。而在下载大量图片时,可以使用多线程加快下载速度。此外,还可以使用Pillow库对图片进行进一步的处理和操作。
无论选择哪种方法,都需要注意处理异常情况,如网络连接错误、请求超时等,以确保程序的稳定性和可靠性。通过合理的异常处理和优化,可以提高图片下载的效率和稳定性。希望本文对您在Python下载图片方面有所帮助。
相关问答FAQs:
如何使用Python下载特定格式的图片?
要使用Python下载特定格式的图片(如JPEG、PNG等),可以使用requests
库结合PIL
(Pillow)库。首先,确保安装这两个库。可以通过命令行运行pip install requests Pillow
来安装。接下来,使用requests.get()
方法获取图片链接,然后使用PIL.Image
保存为所需格式。
在下载图片时,如何处理网络错误和异常?
在下载图片时,网络错误和异常是常见问题。使用try-except
语句可以有效捕获这些错误。例如,您可以捕获requests.exceptions.RequestException
来处理网络问题,确保程序在遇到问题时不会崩溃,并给出适当的错误提示。
如何批量下载网上的图片?
批量下载网上的图片可以通过循环遍历图片链接列表来实现。您可以将所有图片的URL存储在一个列表中,然后使用for
循环逐个下载。在下载每张图片时,可以使用上述的错误处理机制,确保每次下载都能正常进行,并能够处理可能出现的异常情况。
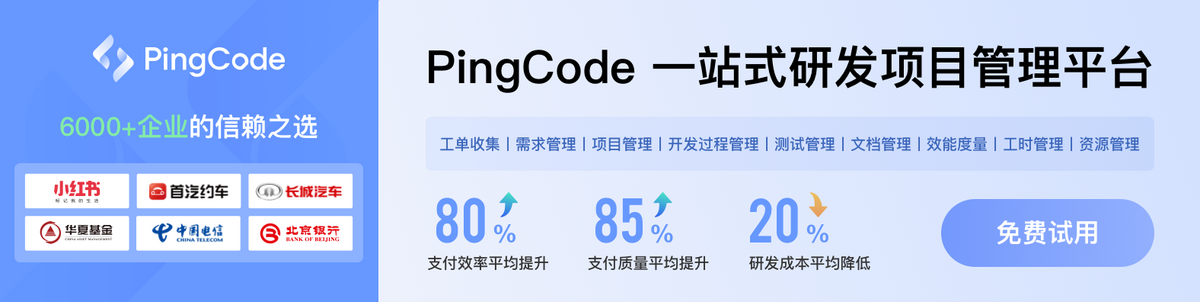