要用Python做出数字倒计时,可以采用以下几种方法:使用time.sleep()
函数、结合tkinter
图形界面库、或是使用pygame
库。最简单的方法是通过time.sleep()
函数来实现倒计时功能,具体步骤包括:导入所需模块、定义倒计时函数、使用循环控制时间、在每次循环中更新显示内容。接下来我们详细介绍一种通过time.sleep()
函数实现数字倒计时的方法。
详细描述:使用time.sleep()
函数
在使用time.sleep()
函数来实现倒计时时,我们需要导入time
模块,并编写一个倒计时函数。该函数将接受一个代表秒数的参数,通过循环逐秒减少该秒数,并在每次循环中打印剩余时间。以下是一个简单的实现示例:
import time
def countdown_timer(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = '{:02d}:{:02d}'.format(mins, secs)
print(timer, end="\r")
time.sleep(1)
seconds -= 1
print("Time's up!")
Example usage
countdown_timer(10)
一、导入所需模块
在编写倒计时程序时,首先需要导入Python标准库中的time
模块。time
模块提供了处理时间的多种方法,其中time.sleep()
函数可以使程序暂停指定的秒数,这对实现倒计时非常有用。
import time
二、定义倒计时函数
定义一个名为countdown_timer
的函数,该函数接受一个整数参数seconds
,表示倒计时的总秒数。在函数内部,通过while
循环来实现倒计时功能。
def countdown_timer(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = '{:02d}:{:02d}'.format(mins, secs)
print(timer, end="\r")
time.sleep(1)
seconds -= 1
print("Time's up!")
三、使用循环控制时间
在while
循环中,每次循环都会调用time.sleep(1)
函数,使程序暂停1秒钟。与此同时,使用divmod
函数将总秒数转换为分钟和秒数,并格式化为MM:SS
的字符串形式。然后,通过print
函数在终端中输出当前剩余时间。
四、在每次循环中更新显示内容
为了在每次循环中更新显示内容,可以使用end="\r"
参数,使输出在同一行中进行覆盖。这种方式可以避免在终端中打印大量无用的行。循环结束后,打印提示信息“Time's up!”。
五、完整示例代码
以下是完整的倒计时程序示例代码:
import time
def countdown_timer(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = '{:02d}:{:02d}'.format(mins, secs)
print(timer, end="\r")
time.sleep(1)
seconds -= 1
print("Time's up!")
Example usage
countdown_timer(10)
六、扩展功能
除了上述基本的倒计时功能,还可以对代码进行扩展,以实现更多功能。例如,可以使用tkinter
库创建图形界面倒计时器,或是使用pygame
库来实现带有声音提示的倒计时器。以下是一个使用tkinter
库实现的倒计时器示例代码:
import tkinter as tk
import time
def countdown_timer(label, seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = '{:02d}:{:02d}'.format(mins, secs)
label.config(text=timer)
time.sleep(1)
seconds -= 1
label.config(text="Time's up!")
def start_timer():
countdown_timer(label, int(entry.get()))
root = tk.Tk()
root.title("Countdown Timer")
entry = tk.Entry(root)
entry.pack()
label = tk.Label(root, font=('Helvetica', 48))
label.pack()
start_button = tk.Button(root, text="Start", command=start_timer)
start_button.pack()
root.mainloop()
在这个示例中,使用tkinter
库创建了一个简单的图形界面倒计时器。用户可以输入倒计时时间,并点击“Start”按钮开始倒计时。
通过上述几种方法,我们可以轻松地使用Python实现数字倒计时功能。根据具体需求,可以选择合适的实现方式,并进行相应的扩展和优化。
相关问答FAQs:
如何用Python创建简单的倒计时程序?
要创建一个简单的倒计时程序,可以使用Python的time
模块。首先,确定倒计时的总秒数,然后使用一个循环来逐秒减少并打印剩余时间。以下是一个基本的示例代码:
import time
def countdown(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = '{:02d}:{:02d}'.format(mins, secs)
print(timer, end='\r')
time.sleep(1)
seconds -= 1
print("时间到!")
countdown(10) # 设置倒计时为10秒
如何在倒计时中添加用户输入的时间?
可以通过input()
函数来获取用户输入的时间。确保将输入的字符串转换为整数,以便在倒计时程序中使用。示例如下:
user_input = int(input("请输入倒计时的秒数: "))
countdown(user_input)
怎样在Python倒计时中增加音效或通知功能?
为了增强倒计时体验,可以在倒计时结束时添加音效或弹出通知。可以使用playsound
库来播放音效,或者使用plyer
库来显示通知。以下是一个示例:
from playsound import playsound
from plyer import notification
def countdown_with_sound(seconds):
while seconds:
# 倒计时代码
seconds -= 1
# 播放音效
playsound('alarm.mp3') # 确保有一个叫 alarm.mp3 的音频文件
# 显示通知
notification.notify(
title='倒计时完成!',
message='时间到!',
timeout=10,
)
countdown_with_sound(10)
这样,你就可以用Python制作出功能丰富的数字倒计时程序。
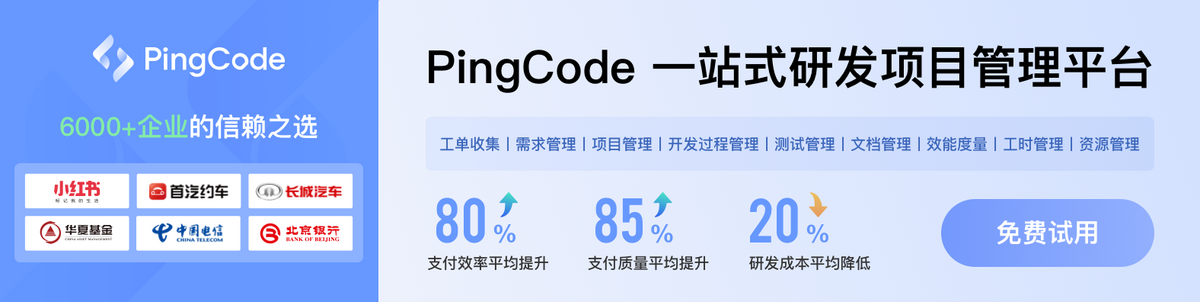