一、安装必要的库和工具、导入数据
在Python中建立语料库,首先需要安装必要的库和工具,如NLTK、spaCy等,并导入相关的数据。NLTK、spaCy、导入数据是核心步骤。NLTK提供了丰富的文本处理工具和语料库资源,而spaCy则以其高效的自然语言处理能力而闻名。导入数据是关键的一步,因为没有数据,语料库就无法建立。在这篇文章中,我们将详细介绍如何安装这些库,并导入并处理数据。
安装必要的库和工具
建立语料库的第一步是安装必要的库和工具。以下是一些常用的库:
-
NLTK:Natural Language Toolkit (NLTK) 是一个强大的 Python 库,支持多种自然语言处理 (NLP) 任务。安装方法如下:
pip install nltk
-
spaCy:spaCy 是一个快速、高效的自然语言处理库,适用于大规模数据处理。安装方法如下:
pip install spacy
-
Pandas:Pandas 是一个数据操作和分析的库,非常适合处理结构化数据。安装方法如下:
pip install pandas
-
BeautifulSoup:用于从网页抓取数据的库。安装方法如下:
pip install beautifulsoup4
-
Requests:用于发送 HTTP 请求的库,常用于从网络获取数据。安装方法如下:
pip install requests
导入数据
导入数据是建立语料库的关键步骤,数据可以来自多种来源,如文件、数据库、API 或网络抓取。以下是一些常见的导入数据的方法:
1. 从本地文件导入数据
可以从文本文件、CSV 文件、Excel 文件等导入数据。例如,从文本文件导入数据:
with open('data.txt', 'r', encoding='utf-8') as file:
data = file.read()
从 CSV 文件导入数据:
import pandas as pd
data = pd.read_csv('data.csv')
2. 从数据库导入数据
可以使用数据库连接库(如 SQLAlchemy)从数据库中导入数据。例如,从 MySQL 数据库导入数据:
from sqlalchemy import create_engine
import pandas as pd
engine = create_engine('mysql://username:password@host:port/database')
data = pd.read_sql('SELECT * FROM table_name', engine)
3. 从 API 导入数据
可以使用 Requests 库从 API 获取数据。例如,从一个 RESTful API 获取数据:
import requests
response = requests.get('https://api.example.com/data')
data = response.json()
4. 从网页抓取数据
可以使用 BeautifulSoup 库从网页抓取数据。例如,从网页抓取数据:
import requests
from bs4 import BeautifulSoup
response = requests.get('https://www.example.com')
soup = BeautifulSoup(response.content, 'html.parser')
data = soup.find_all('p') # 获取所有段落
导入数据后,需要对数据进行预处理,以便后续的语料库构建和分析。
二、数据预处理
数据预处理是建立语料库的一个关键步骤,它包括去除噪音、标记化、词干提取、词性标注、命名实体识别等步骤。去除噪音、标记化、词干提取是核心步骤。去除噪音可以提高数据质量,标记化是将文本分割成单独的词或句子,词干提取是将词语还原为其词干形式。以下是详细介绍每个步骤的内容。
去除噪音
去除噪音是数据预处理的第一步,包括去除标点符号、特殊字符、停用词等。以下是一些常见的去除噪音的方法:
- 去除标点符号和特殊字符:
import re
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
data = [remove_punctuation(sentence) for sentence in data]
- 去除停用词:
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
def remove_stopwords(text):
return ' '.join([word for word in text.split() if word.lower() not in stop_words])
data = [remove_stopwords(sentence) for sentence in data]
标记化
标记化是将文本分割成单独的词或句子。以下是一些常见的标记化方法:
- 词标记化:
from nltk.tokenize import word_tokenize
data = [word_tokenize(sentence) for sentence in data]
- 句子标记化:
from nltk.tokenize import sent_tokenize
data = [sent_tokenize(paragraph) for paragraph in data]
词干提取
词干提取是将词语还原为其词干形式。以下是一些常见的词干提取方法:
- 使用 Porter 词干提取器:
from nltk.stem import PorterStemmer
stemmer = PorterStemmer()
def stem_words(words):
return [stemmer.stem(word) for word in words]
data = [stem_words(sentence) for sentence in data]
- 使用 Lancaster 词干提取器:
from nltk.stem import LancasterStemmer
stemmer = LancasterStemmer()
data = [stem_words(sentence) for sentence in data]
词性标注
词性标注是为每个词分配一个词性标签。以下是一个常见的词性标注方法:
from nltk import pos_tag
data = [pos_tag(sentence) for sentence in data]
命名实体识别
命名实体识别是识别文本中的命名实体(如人名、地名、组织名等)。以下是一个常见的命名实体识别方法:
import spacy
nlp = spacy.load('en_core_web_sm')
def named_entity_recognition(text):
doc = nlp(text)
return [(entity.text, entity.label_) for entity in doc.ents]
data = [named_entity_recognition(sentence) for sentence in data]
通过这些步骤,我们可以对数据进行预处理,为建立语料库做好准备。
三、构建语料库
在完成数据预处理之后,接下来就是构建语料库。创建语料库对象、添加数据到语料库是核心步骤。创建语料库对象可以管理和操作语料库中的数据,而添加数据到语料库是将预处理后的数据存储到语料库中。以下是详细介绍如何构建语料库的内容。
创建语料库对象
可以使用 NLTK 或自定义类来创建语料库对象。以下是一个使用 NLTK 创建语料库对象的示例:
from nltk.corpus import PlaintextCorpusReader
corpus_root = './corpus'
wordlists = PlaintextCorpusReader(corpus_root, '.*')
以下是一个自定义类创建语料库对象的示例:
class Corpus:
def __init__(self):
self.data = []
def add_document(self, document):
self.data.append(document)
def get_documents(self):
return self.data
corpus = Corpus()
添加数据到语料库
将预处理后的数据添加到语料库中。以下是一些常见的方法:
- 使用 NLTK 添加数据到语料库:
import os
if not os.path.exists(corpus_root):
os.makedirs(corpus_root)
for i, document in enumerate(data):
with open(os.path.join(corpus_root, f'doc_{i}.txt'), 'w', encoding='utf-8') as file:
file.write(' '.join(document))
- 使用自定义类添加数据到语料库:
for document in data:
corpus.add_document(document)
保存和加载语料库
保存和加载语料库是管理语料库的重要步骤。以下是一些常见的方法:
- 使用 NLTK 保存和加载语料库:
保存语料库:
import pickle
with open('corpus.pkl', 'wb') as file:
pickle.dump(wordlists, file)
加载语料库:
with open('corpus.pkl', 'rb') as file:
wordlists = pickle.load(file)
- 使用自定义类保存和加载语料库:
保存语料库:
with open('corpus.pkl', 'wb') as file:
pickle.dump(corpus, file)
加载语料库:
with open('corpus.pkl', 'rb') as file:
corpus = pickle.load(file)
通过这些步骤,我们可以成功构建语料库,并进行保存和加载操作。
四、语料库分析
在构建语料库之后,接下来是进行语料库分析。频率分析、共现分析、情感分析是核心步骤。频率分析可以发现文本中常见的词语,共现分析可以发现词语之间的关系,情感分析可以评估文本的情感倾向。以下是详细介绍每个步骤的内容。
频率分析
频率分析是统计文本中词语出现的频率。以下是一些常见的频率分析方法:
- 使用 NLTK 进行频率分析:
from nltk.probability import FreqDist
fdist = FreqDist()
for document in corpus.get_documents():
for word in document:
fdist[word] += 1
print(fdist.most_common(10))
- 使用 Pandas 进行频率分析:
import pandas as pd
words = [word for document in corpus.get_documents() for word in document]
word_counts = pd.Series(words).value_counts()
print(word_counts.head(10))
共现分析
共现分析是分析词语之间的共现关系。以下是一些常见的共现分析方法:
- 使用 NLTK 进行共现分析:
from nltk.collocations import BigramCollocationFinder
from nltk.metrics import BigramAssocMeasures
bigram_finder = BigramCollocationFinder.from_words(words)
bigrams = bigram_finder.nbest(BigramAssocMeasures.likelihood_ratio, 10)
print(bigrams)
- 使用 Pandas 进行共现分析:
from collections import Counter
bigrams = [(words[i], words[i+1]) for i in range(len(words)-1)]
bigram_counts = pd.Series(bigrams).value_counts()
print(bigram_counts.head(10))
情感分析
情感分析是评估文本的情感倾向。以下是一些常见的情感分析方法:
- 使用 NLTK 进行情感分析:
from nltk.sentiment import SentimentIntensityAnalyzer
sia = SentimentIntensityAnalyzer()
sentiments = [sia.polarity_scores(' '.join(document)) for document in corpus.get_documents()]
print(sentiments)
- 使用 TextBlob 进行情感分析:
from textblob import TextBlob
sentiments = [TextBlob(' '.join(document)).sentiment for document in corpus.get_documents()]
print(sentiments)
通过这些步骤,我们可以对语料库进行频率分析、共现分析和情感分析,从而深入理解文本内容。
五、应用与展示
在完成语料库分析之后,接下来是将分析结果应用和展示。可视化分析结果、应用模型是核心步骤。可视化分析结果可以直观展示文本特征,应用模型可以进行预测和分类。以下是详细介绍每个步骤的内容。
可视化分析结果
可视化分析结果是展示分析结果的重要步骤。以下是一些常见的可视化方法:
- 使用 Matplotlib 可视化频率分析结果:
import matplotlib.pyplot as plt
word_counts.head(10).plot(kind='bar')
plt.title('Top 10 Most Common Words')
plt.show()
- 使用 NetworkX 可视化共现分析结果:
import networkx as nx
G = nx.Graph()
G.add_edges_from(bigram_counts.head(10).index)
nx.draw(G, with_labels=True)
plt.title('Top 10 Most Common Bigrams')
plt.show()
应用模型
应用模型是使用分析结果进行预测和分类的重要步骤。以下是一些常见的应用模型方法:
- 使用 scikit-learn 进行文本分类:
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import accuracy_score
vectorizer = CountVectorizer()
X = vectorizer.fit_transform([' '.join(document) for document in corpus.get_documents()])
y = [label for document in corpus.get_documents()]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = MultinomialNB()
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
print(accuracy_score(y_test, y_pred))
- 使用 TensorFlow 进行文本分类:
import tensorflow as tf
from tensorflow.keras.preprocessing.text import Tokenizer
from tensorflow.keras.preprocessing.sequence import pad_sequences
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Embedding, LSTM, Dense
tokenizer = Tokenizer()
tokenizer.fit_on_texts([' '.join(document) for document in corpus.get_documents()])
X = tokenizer.texts_to_sequences([' '.join(document) for document in corpus.get_documents()])
X = pad_sequences(X, padding='post')
y = [label for document in corpus.get_documents()]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = Sequential([
Embedding(input_dim=len(tokenizer.word_index)+1, output_dim=64, input_length=X.shape[1]),
LSTM(64),
Dense(1, activation='sigmoid')
])
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
model.fit(X_train, y_train, epochs=5, validation_data=(X_test, y_test))
通过这些步骤,我们可以将分析结果应用于文本分类和预测,并进行可视化展示。
总结:本文详细介绍了如何在Python中建立语料库,包括安装必要的库和工具、导入数据、数据预处理、构建语料库、语料库分析、应用与展示等步骤。希望通过本文的介绍,读者能够掌握建立语料库的基本方法,并能够在实际项目中应用这些方法进行文本分析和处理。
相关问答FAQs:
如何选择合适的文本数据作为语料库?
在建立语料库时,选择合适的文本数据至关重要。理想的文本数据应具备代表性,涵盖所需研究领域的多样性和复杂性。您可以从公开的数据集、网络爬虫抓取的内容或自己撰写的文本中获取数据。此外,确保数据的清洁和格式一致,这对后续的处理和分析非常重要。
在Python中如何处理和清洗语料库?
处理和清洗语料库通常包括去除标点符号、特殊字符和多余空格,以及进行分词、词干提取和停用词去除等操作。可以使用Python中的nltk
或spacy
库来执行这些任务。具体来说,您可以利用nltk
中的word_tokenize
进行分词,同时使用stopwords
模块去除常见的停用词,确保语料库的质量和有效性。
如何有效地存储和管理语料库?
管理和存储语料库可以选择多种方式,如使用文本文件、数据库或专门的文档管理系统。文本文件简单易用,但对于大规模数据,使用数据库(如SQLite或MongoDB)会更高效。确保为语料库设置合理的目录结构,以便于分类和检索。此外,定期备份和版本控制也是保护语料库的重要措施。
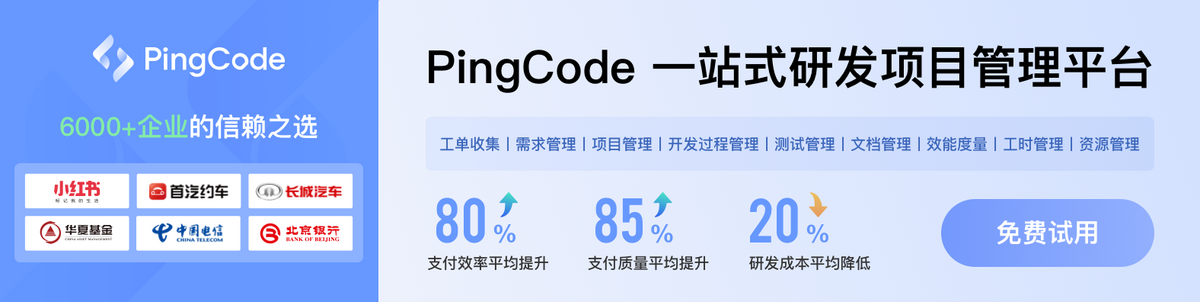