Python遍历文件夹下的图像可以使用os模块、glob模块、以及路径库pathlib,结合文件处理方法进行图像遍历。其中,os模块和glob模块分别提供了基础的操作系统接口和通配符匹配功能,而pathlib库则为路径操作提供了面向对象的接口。
下面将详细介绍如何使用这些方法来遍历文件夹下的图像。
一、使用os模块
os模块提供了一些函数来与操作系统进行交互,其中包括遍历目录结构的功能。下面是使用os模块遍历文件夹下的图像文件的详细步骤:
1. 获取文件列表
可以使用os.listdir()函数来获取指定目录中的文件列表:
import os
def get_image_files(directory):
return [file for file in os.listdir(directory) if file.endswith(('jpg', 'png', 'jpeg'))]
directory = 'path/to/your/image/directory'
image_files = get_image_files(directory)
print(image_files)
2. 递归遍历子目录
如果需要递归遍历子目录,可以使用os.walk()函数:
import os
def get_all_image_files(directory):
image_files = []
for root, dirs, files in os.walk(directory):
for file in files:
if file.endswith(('jpg', 'png', 'jpeg')):
image_files.append(os.path.join(root, file))
return image_files
directory = 'path/to/your/image/directory'
image_files = get_all_image_files(directory)
print(image_files)
二、使用glob模块
glob模块提供了一个函数用于文件通配符匹配,可以使用它来查找符合特定模式的文件。
1. 简单匹配
可以使用glob.glob()函数来匹配指定目录中的图像文件:
import glob
def get_image_files(directory):
image_files = glob.glob(f'{directory}/*.jpg') + glob.glob(f'{directory}/*.png') + glob.glob(f'{directory}/*.jpeg')
return image_files
directory = 'path/to/your/image/directory'
image_files = get_image_files(directory)
print(image_files)
2. 递归匹配
glob模块的glob()函数从Python 3.5开始支持递归匹配,使用通配符:
import glob
def get_all_image_files(directory):
return glob.glob(f'{directory}/<strong>/*.jpg', recursive=True) + glob.glob(f'{directory}/</strong>/*.png', recursive=True) + glob.glob(f'{directory}//*.jpeg', recursive=True)
directory = 'path/to/your/image/directory'
image_files = get_all_image_files(directory)
print(image_files)
三、使用pathlib库
pathlib库提供了一些面向对象的路径操作方法,可以简化文件和目录的操作。
1. 获取文件列表
可以使用pathlib.Path类来获取文件列表:
from pathlib import Path
def get_image_files(directory):
return [str(file) for file in Path(directory).glob('*.jpg')] + [str(file) for file in Path(directory).glob('*.png')] + [str(file) for file in Path(directory).glob('*.jpeg')]
directory = 'path/to/your/image/directory'
image_files = get_image_files(directory)
print(image_files)
2. 递归遍历子目录
可以使用pathlib.Path.rglob()方法来递归遍历子目录:
from pathlib import Path
def get_all_image_files(directory):
return [str(file) for file in Path(directory).rglob('*.jpg')] + [str(file) for file in Path(directory).rglob('*.png')] + [str(file) for file in Path(directory).rglob('*.jpeg')]
directory = 'path/to/your/image/directory'
image_files = get_all_image_files(directory)
print(image_files)
四、结合其他库进行图像处理
在遍历文件夹下的图像后,通常需要对图像进行进一步处理。Python提供了许多图像处理库,如Pillow、OpenCV等。下面是如何结合这些库进行图像处理的示例:
1. 使用Pillow库
Pillow是Python Imaging Library的一个分支,提供了丰富的图像处理功能:
from PIL import Image
import os
def process_image(image_path):
with Image.open(image_path) as img:
img = img.convert('L') # 转换为灰度图像
img.show()
directory = 'path/to/your/image/directory'
image_files = get_all_image_files(directory)
for image_file in image_files:
process_image(image_file)
2. 使用OpenCV库
OpenCV是一个开源的计算机视觉库,提供了强大的图像处理功能:
import cv2
import os
def process_image(image_path):
img = cv2.imread(image_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # 转换为灰度图像
cv2.imshow('Gray Image', gray)
cv2.waitKey(0)
cv2.destroyAllWindows()
directory = 'path/to/your/image/directory'
image_files = get_all_image_files(directory)
for image_file in image_files:
process_image(image_file)
五、性能优化
在处理大量图像时,性能优化是一个重要的考虑因素。可以使用以下方法来提高遍历和处理图像的效率:
1. 多线程
使用多线程可以提高图像处理的速度,尤其是在处理I/O密集型任务时:
import os
import threading
from PIL import Image
def process_image(image_path):
with Image.open(image_path) as img:
img = img.convert('L')
img.show()
def worker(image_files):
for image_file in image_files:
process_image(image_file)
directory = 'path/to/your/image/directory'
image_files = get_all_image_files(directory)
num_threads = 4
chunk_size = len(image_files) // num_threads
threads = []
for i in range(num_threads):
start = i * chunk_size
end = (i + 1) * chunk_size if i != num_threads - 1 else len(image_files)
thread = threading.Thread(target=worker, args=(image_files[start:end],))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2. 多进程
使用多进程可以进一步提高性能,尤其是在处理CPU密集型任务时:
import os
from multiprocessing import Pool
from PIL import Image
def process_image(image_path):
with Image.open(image_path) as img:
img = img.convert('L')
img.show()
directory = 'path/to/your/image/directory'
image_files = get_all_image_files(directory)
with Pool(processes=4) as pool:
pool.map(process_image, image_files)
六、总结
通过以上方法,我们可以使用os模块、glob模块、pathlib库来遍历文件夹下的图像,并结合Pillow、OpenCV等库对图像进行进一步处理。多线程和多进程技术可以显著提高图像处理的效率。
选择合适的方法和库,可以根据具体需求和性能要求来优化图像处理任务。无论是简单的图像遍历,还是复杂的图像处理任务,Python都提供了丰富的工具和库来帮助我们高效地完成这些任务。
相关问答FAQs:
如何使用Python遍历文件夹中的所有图像文件?
要遍历文件夹中的所有图像文件,可以使用os
和glob
库。通过os.listdir()
获取文件夹内的文件列表,或者使用glob.glob()
来匹配特定图像格式(如.jpg
, .png
)。这两种方法都能帮助你轻松找到所需的图像文件。
Python中如何筛选特定类型的图像文件?
在遍历文件夹时,可以使用条件语句来筛选特定类型的图像文件。例如,可以检查文件扩展名是否为.jpg
、.png
等。结合os.path.splitext()
方法,可以有效地获取文件的扩展名,进而筛选出所需格式的图像。
如何提高遍历图像文件的效率?
为了提高遍历图像文件的效率,可以使用多线程或异步编程来并行处理文件。另一个方法是仅在需要时加载图像,而不是一次性加载所有图像,这样可以节省内存并加快处理速度。使用PIL
库中的Image.open()
方法可以在需要时打开图像文件。
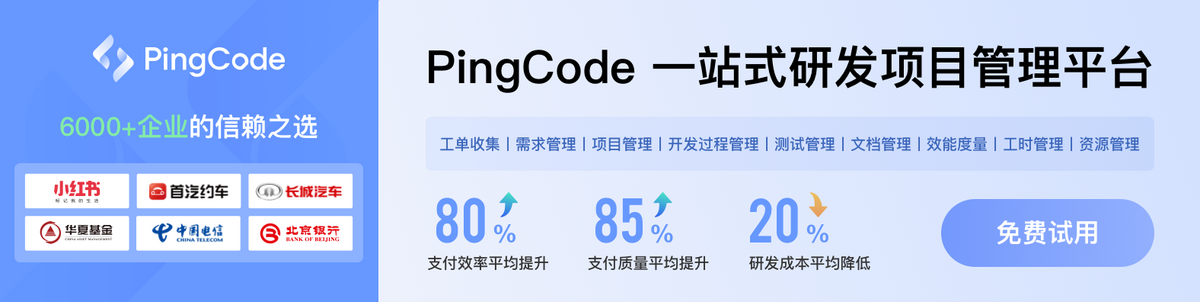