Python最终结果如何取整?
在Python中,最终结果取整的方式有多种,主要包括使用内置函数round()
, math.floor()
, math.ceil()
和类型转换等方式。这些方法各有其特点和适用场景,例如:round()
函数可以四舍五入取整、math.floor()
函数向下取整、math.ceil()
函数向上取整、类型转换直接截断小数部分。下面我们将详细介绍这些方法以及它们的具体用法和注意事项。
一、使用round()
函数取整
round()
函数是Python内置的一个函数,专门用于对浮点数进行四舍五入取整。
1、基本用法
round()
函数的基本用法如下:
result = round(3.14159)
print(result) # 输出: 3
在这个例子中,round()
函数将浮点数3.14159四舍五入为整数3。
2、保留指定小数位数
round()
函数还可以保留指定的小数位数,通过传入第二个参数来实现:
result = round(3.14159, 2)
print(result) # 输出: 3.14
在这个例子中,round()
函数将浮点数3.14159四舍五入到小数点后两位,结果为3.14。
二、使用math.floor()
函数取整
math.floor()
函数用于向下取整,即返回不大于输入值的最大整数。
1、基本用法
首先需要导入math
模块,然后使用math.floor()
函数:
import math
result = math.floor(3.14159)
print(result) # 输出: 3
在这个例子中,math.floor()
函数将浮点数3.14159向下取整为整数3。
2、处理负数
math.floor()
函数也适用于负数:
result = math.floor(-3.14159)
print(result) # 输出: -4
在这个例子中,math.floor()
函数将浮点数-3.14159向下取整为整数-4。
三、使用math.ceil()
函数取整
math.ceil()
函数用于向上取整,即返回不小于输入值的最小整数。
1、基本用法
首先需要导入math
模块,然后使用math.ceil()
函数:
import math
result = math.ceil(3.14159)
print(result) # 输出: 4
在这个例子中,math.ceil()
函数将浮点数3.14159向上取整为整数4。
2、处理负数
math.ceil()
函数也适用于负数:
result = math.ceil(-3.14159)
print(result) # 输出: -3
在这个例子中,math.ceil()
函数将浮点数-3.14159向上取整为整数-3。
四、使用类型转换取整
类型转换是另一种取整的方法,通过将浮点数转换为整数类型来实现。
1、使用int()
函数
int()
函数可以将浮点数直接转换为整数,结果是直接截断小数部分:
result = int(3.14159)
print(result) # 输出: 3
在这个例子中,int()
函数将浮点数3.14159转换为整数3。
2、注意负数处理
对于负数,int()
函数也是直接截断小数部分:
result = int(-3.14159)
print(result) # 输出: -3
在这个例子中,int()
函数将浮点数-3.14159转换为整数-3。
五、使用decimal.Decimal
模块取整
decimal
模块提供了更高精度的浮点数操作,其中的Decimal
类型也可以进行取整操作。
1、基本用法
首先需要导入decimal
模块,然后使用Decimal
类型的quantize()
方法:
from decimal import Decimal, ROUND_HALF_UP
result = Decimal('3.14159').quantize(Decimal('1'), rounding=ROUND_HALF_UP)
print(result) # 输出: 3
在这个例子中,Decimal
类型的quantize()
方法将浮点数3.14159四舍五入为整数3。
2、其他取整方式
Decimal
类型的quantize()
方法还支持其他取整方式,例如向上取整、向下取整等:
from decimal import Decimal, ROUND_FLOOR, ROUND_CEILING
result_floor = Decimal('3.14159').quantize(Decimal('1'), rounding=ROUND_FLOOR)
result_ceiling = Decimal('3.14159').quantize(Decimal('1'), rounding=ROUND_CEILING)
print(result_floor) # 输出: 3
print(result_ceiling) # 输出: 4
在这个例子中,ROUND_FLOOR
表示向下取整,ROUND_CEILING
表示向上取整。
六、使用numpy
模块取整
numpy
模块是Python中用于科学计算的一个重要库,其中也提供了一些取整函数。
1、使用numpy.floor()
函数
numpy.floor()
函数用于向下取整:
import numpy as np
result = np.floor(3.14159)
print(result) # 输出: 3.0
在这个例子中,numpy.floor()
函数将浮点数3.14159向下取整为整数3.0。
2、使用numpy.ceil()
函数
numpy.ceil()
函数用于向上取整:
import numpy as np
result = np.ceil(3.14159)
print(result) # 输出: 4.0
在这个例子中,numpy.ceil()
函数将浮点数3.14159向上取整为整数4.0。
七、使用pandas
模块取整
pandas
模块是Python中用于数据分析的一个重要库,其中也提供了一些取整函数。
1、使用pandas.Series.round()
方法
pandas.Series.round()
方法用于对Series
对象进行取整:
import pandas as pd
s = pd.Series([3.14159, 2.71828, 1.61803])
result = s.round()
print(result)
输出:
0 3
1 3
2 2
dtype: int64
在这个例子中,pandas.Series.round()
方法将Series
对象中的所有浮点数四舍五入取整。
2、使用pandas.DataFrame.round()
方法
pandas.DataFrame.round()
方法用于对DataFrame
对象进行取整:
import pandas as pd
df = pd.DataFrame({'A': [3.14159, 2.71828], 'B': [1.61803, 0.57721]})
result = df.round()
print(result)
输出:
A B
0 3 2
1 2 1
在这个例子中,pandas.DataFrame.round()
方法将DataFrame
对象中的所有浮点数四舍五入取整。
八、总结
在Python中,取整的方法有很多种,每种方法都有其特点和适用场景。round()
函数适用于四舍五入取整、math.floor()
函数适用于向下取整、math.ceil()
函数适用于向上取整、类型转换适用于直接截断小数部分、decimal
模块适用于高精度取整、numpy
模块适用于科学计算、pandas
模块适用于数据分析。在实际应用中,可以根据具体需求选择合适的取整方法。
相关问答FAQs:
如何在Python中实现向上取整和向下取整?
在Python中,可以使用math.ceil()
函数来实现向上取整,而使用math.floor()
函数可以实现向下取整。例如,math.ceil(3.2)
将返回4,而math.floor(3.8)
将返回3。确保在使用这些函数时,首先导入math
模块。
Python中有什么方法可以进行四舍五入?
在Python中,可以使用内置的round()
函数进行四舍五入。该函数的基本用法是round(number, ndigits)
,其中number
是要四舍五入的数,ndigits
是要保留的小数位数。如果未指定ndigits
,则默认四舍五入到最近的整数。
如何在Python中处理负数的取整?
处理负数时,math.floor()
会返回小于或等于该数的最大整数,而math.ceil()
将返回大于或等于该数的最小整数。例如,math.floor(-3.2)
会返回-4,而math.ceil(-3.8)
将返回-3。这与正数的取整方式有所不同,使用时请注意。
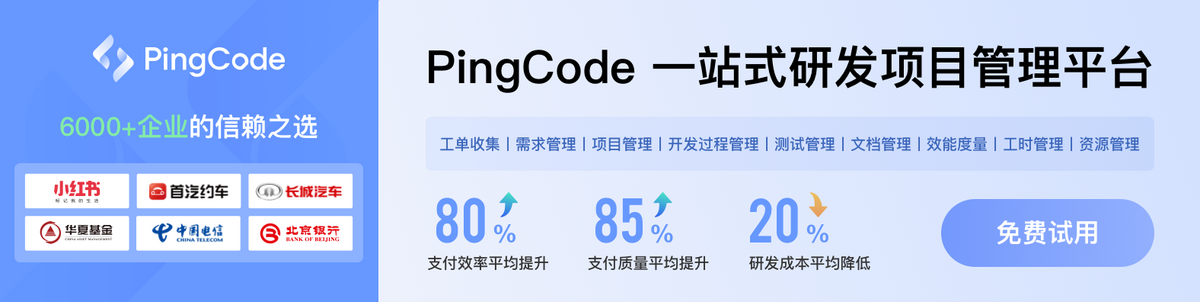