要用Python爬取微信数据,可以使用Selenium、Requests、BeautifulSoup等工具,结合微信公众平台的API、模拟登录、解析网页内容等方法。以下是详细描述:
-
微信公众平台的API是最直接的方法之一,可以通过获取公众号的API接口来抓取数据,详细描述:微信公众平台提供了许多API接口,可以用来获取公众号文章、用户信息等数据。使用这些API时,需要先注册一个微信公众平台账号,并通过审核获取接口权限。通过这些API可以编写脚本自动化获取数据。
-
模拟登录是爬取私人数据的关键步骤,详细描述:在爬取私人微信数据时,通常需要模拟用户登录微信账号。可以使用Selenium工具来模拟浏览器操作,自动完成登录过程。Selenium可以控制浏览器打开微信网页,输入用户名和密码,点击登录按钮,获取登录后的页面内容。
-
解析网页内容可以帮助获取特定的数据,详细描述:在获取到需要的网页内容后,使用BeautifulSoup或lxml等HTML解析库来解析网页结构,提取出需要的数据。通过遍历HTML节点,可以获取文章标题、发布日期、阅读量、点赞数等信息。
-
处理动态加载的数据,详细描述:有些数据在网页初次加载时并不会显示,而是通过JavaScript动态加载的。可以使用Selenium或其他工具来等待页面完全加载后,再进行数据提取。或者使用浏览器的开发者工具,找到动态加载的接口,直接请求这些接口获取数据。
-
数据存储与处理,详细描述:在获取到微信数据后,可以将其存储到数据库(如MySQL、MongoDB)或文件(如CSV、JSON)中,便于后续的数据分析与处理。可以编写数据处理脚本,对数据进行清洗、整理、分析,生成可视化报表或图表。
一、微信公众平台的API
微信公众平台提供了多种API接口,可以获取公众号的各种数据。使用这些API时,需要先注册一个微信公众平台账号,通过审核后才能获取接口权限。以下是一些常用的API接口:
- 获取公众号文章列表:通过这个接口,可以获取公众号发布的所有文章列表,包括文章标题、摘要、发布时间等信息。
- 获取文章内容:通过这个接口,可以获取指定文章的详细内容,包括正文、图片、视频等。
- 获取用户信息:通过这个接口,可以获取公众号的用户信息,包括用户的昵称、头像、性别、地区等。
使用API接口时,需要先获取access_token,这是访问所有API接口的凭证。获取access_token的方法如下:
import requests
def get_access_token(appid, appsecret):
url = f"https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid={appid}&secret={appsecret}"
response = requests.get(url)
data = response.json()
return data['access_token']
获取到access_token后,可以使用其他接口获取数据。例如,获取公众号文章列表:
def get_article_list(access_token, offset=0, count=10):
url = f"https://api.weixin.qq.com/cgi-bin/material/batchget_material?access_token={access_token}"
payload = {
"type": "news",
"offset": offset,
"count": count
}
response = requests.post(url, json=payload)
data = response.json()
return data['item']
二、模拟登录
在爬取私人微信数据时,需要模拟用户登录微信账号。可以使用Selenium工具来模拟浏览器操作,自动完成登录过程。以下是使用Selenium模拟登录微信网页版的步骤:
- 安装Selenium和浏览器驱动(如ChromeDriver)
- 编写Selenium脚本,打开微信网页版,输入用户名和密码,点击登录按钮
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
def login_wechat(username, password):
driver = webdriver.Chrome() # 或者使用其他浏览器驱动
driver.get("https://wx.qq.com/")
# 输入用户名
username_input = driver.find_element(By.XPATH, '//*[@id="username"]')
username_input.send_keys(username)
# 输入密码
password_input = driver.find_element(By.XPATH, '//*[@id="password"]')
password_input.send_keys(password)
# 点击登录按钮
login_button = driver.find_element(By.XPATH, '//*[@id="login_button"]')
login_button.click()
# 等待页面加载完成
time.sleep(5)
# 获取登录后的页面内容
page_content = driver.page_source
driver.quit()
return page_content
三、解析网页内容
在获取到需要的网页内容后,可以使用BeautifulSoup或lxml等HTML解析库来解析网页结构,提取出需要的数据。以下是使用BeautifulSoup解析网页内容的示例:
from bs4 import BeautifulSoup
def parse_wechat_page(page_content):
soup = BeautifulSoup(page_content, 'html.parser')
# 获取文章标题
titles = [title.text for title in soup.find_all('h3', class_='weui_media_title')]
# 获取文章摘要
summaries = [summary.text for summary in soup.find_all('p', class_='weui_media_desc')]
# 获取文章链接
links = [link['href'] for link in soup.find_all('a', class_='weui_media_title')]
return titles, summaries, links
通过上述步骤,可以获取到微信页面中的文章标题、摘要、链接等信息。可以根据需要进一步提取其他数据。
四、处理动态加载的数据
有些数据在网页初次加载时并不会显示,而是通过JavaScript动态加载的。可以使用Selenium或其他工具来等待页面完全加载后,再进行数据提取。以下是使用Selenium处理动态加载数据的示例:
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
def wait_for_element(driver, by, value, timeout=10):
element = WebDriverWait(driver, timeout).until(
EC.presence_of_element_located((by, value))
)
return element
def get_dynamic_data():
driver = webdriver.Chrome()
driver.get("https://wx.qq.com/")
# 等待动态加载的元素出现
element = wait_for_element(driver, By.XPATH, '//*[@id="dynamic_element"]')
# 获取动态加载的数据
data = element.text
driver.quit()
return data
五、数据存储与处理
在获取到微信数据后,可以将其存储到数据库(如MySQL、MongoDB)或文件(如CSV、JSON)中,便于后续的数据分析与处理。以下是将数据存储到MySQL数据库的示例:
import mysql.connector
def save_to_mysql(data):
conn = mysql.connector.connect(
host="localhost",
user="your_username",
password="your_password",
database="your_database"
)
cursor = conn.cursor()
for item in data:
sql = "INSERT INTO articles (title, summary, link) VALUES (%s, %s, %s)"
cursor.execute(sql, (item['title'], item['summary'], item['link']))
conn.commit()
cursor.close()
conn.close()
通过上述步骤,可以将微信数据存储到MySQL数据库中。可以编写数据处理脚本,对数据进行清洗、整理、分析,生成可视化报表或图表。
六、示例代码
以下是一个完整的示例代码,展示了如何使用Python爬取微信数据,包括获取access_token、获取文章列表、模拟登录、解析网页内容、处理动态加载数据、存储到数据库等步骤:
import requests
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from bs4 import BeautifulSoup
import mysql.connector
import time
def get_access_token(appid, appsecret):
url = f"https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid={appid}&secret={appsecret}"
response = requests.get(url)
data = response.json()
return data['access_token']
def get_article_list(access_token, offset=0, count=10):
url = f"https://api.weixin.qq.com/cgi-bin/material/batchget_material?access_token={access_token}"
payload = {
"type": "news",
"offset": offset,
"count": count
}
response = requests.post(url, json=payload)
data = response.json()
return data['item']
def login_wechat(username, password):
driver = webdriver.Chrome()
driver.get("https://wx.qq.com/")
username_input = driver.find_element(By.XPATH, '//*[@id="username"]')
username_input.send_keys(username)
password_input = driver.find_element(By.XPATH, '//*[@id="password"]')
password_input.send_keys(password)
login_button = driver.find_element(By.XPATH, '//*[@id="login_button"]')
login_button.click()
time.sleep(5)
page_content = driver.page_source
driver.quit()
return page_content
def parse_wechat_page(page_content):
soup = BeautifulSoup(page_content, 'html.parser')
titles = [title.text for title in soup.find_all('h3', class_='weui_media_title')]
summaries = [summary.text for summary in soup.find_all('p', class_='weui_media_desc')]
links = [link['href'] for link in soup.find_all('a', class_='weui_media_title')]
return titles, summaries, links
def wait_for_element(driver, by, value, timeout=10):
element = WebDriverWait(driver, timeout).until(
EC.presence_of_element_located((by, value))
)
return element
def get_dynamic_data():
driver = webdriver.Chrome()
driver.get("https://wx.qq.com/")
element = wait_for_element(driver, By.XPATH, '//*[@id="dynamic_element"]')
data = element.text
driver.quit()
return data
def save_to_mysql(data):
conn = mysql.connector.connect(
host="localhost",
user="your_username",
password="your_password",
database="your_database"
)
cursor = conn.cursor()
for item in data:
sql = "INSERT INTO articles (title, summary, link) VALUES (%s, %s, %s)"
cursor.execute(sql, (item['title'], item['summary'], item['link']))
conn.commit()
cursor.close()
conn.close()
def main():
appid = "your_appid"
appsecret = "your_appsecret"
access_token = get_access_token(appid, appsecret)
articles = get_article_list(access_token)
for article in articles:
print(f"Title: {article['title']}, Link: {article['url']}")
username = "your_username"
password = "your_password"
page_content = login_wechat(username, password)
titles, summaries, links = parse_wechat_page(page_content)
for title, summary, link in zip(titles, summaries, links):
print(f"Title: {title}, Summary: {summary}, Link: {link}")
dynamic_data = get_dynamic_data()
print(f"Dynamic Data: {dynamic_data}")
data_to_save = [{"title": title, "summary": summary, "link": link} for title, summary, link in zip(titles, summaries, links)]
save_to_mysql(data_to_save)
if __name__ == "__main__":
main()
通过上述示例代码,可以实现用Python爬取微信数据的完整流程,包括获取access_token、获取文章列表、模拟登录、解析网页内容、处理动态加载数据、存储到数据库等步骤。可以根据具体需求调整代码,实现更多功能。
相关问答FAQs:
如何使用Python爬取微信数据的基本步骤是什么?
爬取微信数据的基本步骤包括:首先,安装必要的Python库,如requests和BeautifulSoup,这些库可以帮助你发送HTTP请求并解析HTML内容。接着,了解微信的网页结构,识别出你想要获取的数据所在的HTML标签。然后,通过编写Python代码,使用requests库获取网页内容,并利用BeautifulSoup解析数据。最后,提取所需的信息并保存到本地文件或数据库中。
爬取微信数据时需要注意哪些法律和道德问题?
在爬取微信数据时,用户需要遵循相关法律法规,例如数据保护法和隐私政策。此外,微信平台有明确的使用条款,禁止未经允许的自动化访问和数据采集。在实施爬虫之前,了解并遵守这些条款是至关重要的,以免引发法律纠纷。
有哪些常用的Python库可以帮助爬取微信数据?
在爬取微信数据时,常用的Python库包括requests、BeautifulSoup、Scrapy和Selenium。requests用于发送网络请求,获取网页内容;BeautifulSoup用于解析HTML页面,提取数据;Scrapy是一个强大的爬虫框架,可以处理更复杂的爬取任务;而Selenium则可以模拟浏览器行为,适合处理需要登录或动态加载内容的网站。选择合适的库可以大大提高爬虫的效率和效果。
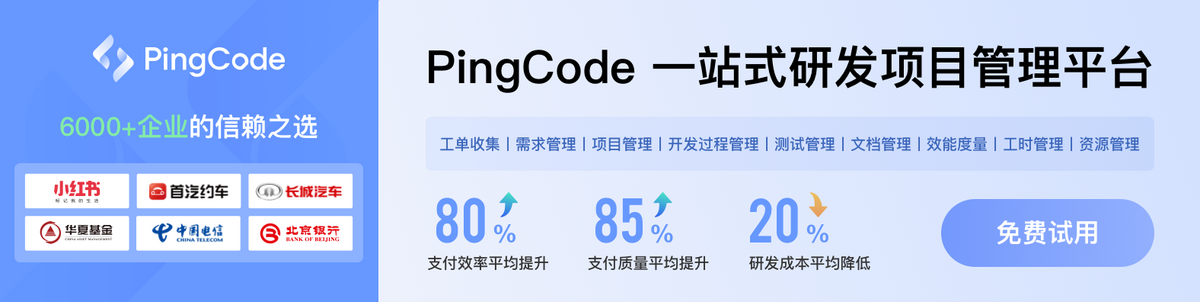