在Python中将文件导入字典的核心方法包括:读取文件内容、解析内容、将内容转换为字典。下面详细介绍一种常见的方式,即通过读取JSON文件导入字典。
一、读取文件内容
首先,需要读取文件内容。假设我们有一个JSON文件data.json
,内容如下:
{
"name": "John",
"age": 30,
"city": "New York"
}
可以使用Python的内置open()
函数和json
库来读取该文件的内容并导入字典。
import json
with open('data.json', 'r') as file:
data = json.load(file)
在这个例子中,json.load(file)
会将文件内容解析为一个字典,并将结果存储在变量data
中。
二、解析内容
如果文件内容不是JSON格式,而是其他格式(例如CSV、XML等),则需要使用相应的库进行解析。例如,假设我们有一个CSV文件data.csv
,内容如下:
name,age,city
John,30,New York
可以使用csv
库来读取该文件并将其内容转换为字典。
import csv
with open('data.csv', mode='r') as file:
csv_reader = csv.DictReader(file)
data = [row for row in csv_reader]
在这个例子中,csv.DictReader(file)
会将CSV文件的每一行转换为一个字典,并将所有这些字典存储在一个列表中。
三、将内容转换为字典
如果文件内容是纯文本格式,需要手动解析内容并将其转换为字典。例如,假设我们有一个纯文本文件data.txt
,内容如下:
name: John
age: 30
city: New York
可以使用以下代码将其内容导入字典:
data = {}
with open('data.txt', 'r') as file:
for line in file:
key, value = line.strip().split(': ')
data[key] = value
在这个例子中,line.strip().split(': ')
会将每一行分割为键和值,并将它们存储在字典data
中。
四、错误处理
在实际操作中,读取文件可能会遇到各种错误,例如文件不存在、文件格式不正确等。因此,添加适当的错误处理是很重要的。例如:
import json
try:
with open('data.json', 'r') as file:
data = json.load(file)
except FileNotFoundError:
print("File not found.")
except json.JSONDecodeError:
print("Error decoding JSON.")
在这个例子中,FileNotFoundError
用于处理文件不存在的情况,json.JSONDecodeError
用于处理JSON格式错误的情况。
五、完整示例
以下是一个完整的示例,演示如何将不同格式的文件内容导入字典:
import json
import csv
def read_json(file_path):
try:
with open(file_path, 'r') as file:
return json.load(file)
except FileNotFoundError:
print("File not found.")
except json.JSONDecodeError:
print("Error decoding JSON.")
return None
def read_csv(file_path):
try:
with open(file_path, mode='r') as file:
csv_reader = csv.DictReader(file)
return [row for row in csv_reader]
except FileNotFoundError:
print("File not found.")
return None
def read_txt(file_path):
data = {}
try:
with open(file_path, 'r') as file:
for line in file:
key, value = line.strip().split(': ')
data[key] = value
return data
except FileNotFoundError:
print("File not found.")
return None
Example usage
json_data = read_json('data.json')
csv_data = read_csv('data.csv')
txt_data = read_txt('data.txt')
print(json_data)
print(csv_data)
print(txt_data)
六、优化和扩展
在实际应用中,可以进一步优化和扩展这些函数,例如,添加对不同编码格式的支持、处理更复杂的文件结构等。例如,添加对UTF-8编码的支持:
def read_file(file_path, encoding='utf-8'):
try:
with open(file_path, 'r', encoding=encoding) as file:
# Read and process file content
pass
except FileNotFoundError:
print("File not found.")
此外,还可以根据文件类型自动选择合适的读取方式:
def read_file(file_path):
if file_path.endswith('.json'):
return read_json(file_path)
elif file_path.endswith('.csv'):
return read_csv(file_path)
elif file_path.endswith('.txt'):
return read_txt(file_path)
else:
print("Unsupported file format.")
return None
七、总结
通过以上方法,可以轻松地将不同格式的文件内容导入字典。在实际应用中,根据具体需求选择合适的解析方法,并结合错误处理和优化策略,可以确保程序的稳定性和可维护性。
相关问答FAQs:
如何将文本文件的数据转换为Python字典?
在Python中,可以使用open()
函数读取文本文件,然后利用split()
方法将每一行的数据分割成键值对,再将其存入字典。例如,假设文件内容如下:
name: Alice
age: 30
city: New York
可以通过以下代码将其转为字典:
data_dict = {}
with open('file.txt', 'r') as file:
for line in file:
key, value = line.strip().split(': ')
data_dict[key] = value
如何处理包含嵌套结构的文件以生成字典?
对于包含嵌套结构的文件(如JSON格式),可以使用Python的json
模块来轻松实现导入。假设文件内容为:
{
"name": "Alice",
"age": 30,
"address": {
"city": "New York",
"zip": "10001"
}
}
可以通过以下代码导入为字典:
import json
with open('file.json', 'r') as file:
data_dict = json.load(file)
这样就可以直接得到一个包含嵌套字典的Python字典。
如何确保导入字典时的数据完整性?
在导入文件至字典时,确保数据完整性可以通过异常处理来实现。使用try
和except
块捕获潜在的错误,如文件未找到或格式错误。例如:
try:
with open('file.txt', 'r') as file:
# 处理文件内容
except FileNotFoundError:
print("文件未找到,请确认路径是否正确。")
except Exception as e:
print(f"发生错误: {e}")
这种方式有助于在导入过程中对任何意外情况进行有效的管理。
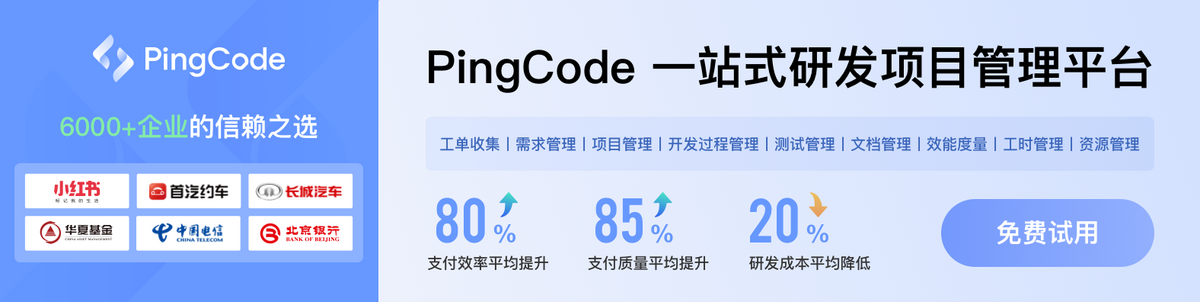