Python可以通过多线程、多进程、异步编程等方式来同时执行两个函数。 其中,多线程适用于I/O密集型任务、多进程适用于CPU密集型任务、异步编程适用于处理大量并发的异步I/O操作。下面将详细介绍每种方法及其实现方式。
一、线程(Threading)
Python的threading
模块提供了一个简单的方法来创建和管理线程。线程是轻量级的,并且适用于I/O密集型任务。
1. 创建线程:
要创建线程,你需要创建一个Thread
对象,并传递目标函数和它的参数。
import threading
def func1():
print("Function 1")
def func2():
print("Function 2")
thread1 = threading.Thread(target=func1)
thread2 = threading.Thread(target=func2)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
在上面的代码中,thread1
和thread2
是两个线程,它们分别执行func1
和func2
。通过调用start()
方法,线程开始执行。join()
方法确保主线程等待子线程执行完毕。
2. 使用线程执行I/O密集型任务:
线程适用于I/O密集型任务,例如网络请求、文件读写等。以下是一个示例,展示了如何使用线程来并发执行两个网络请求:
import threading
import requests
def fetch_url(url):
response = requests.get(url)
print(f"Fetched {url} with status code {response.status_code}")
url1 = "http://example.com"
url2 = "http://example.org"
thread1 = threading.Thread(target=fetch_url, args=(url1,))
thread2 = threading.Thread(target=fetch_url, args=(url2,))
thread1.start()
thread2.start()
thread1.join()
thread2.join()
二、进程(Multiprocessing)
Python的multiprocessing
模块提供了一个简单的方法来创建和管理进程。进程是重量级的,适用于CPU密集型任务。
1. 创建进程:
要创建进程,你需要创建一个Process
对象,并传递目标函数和它的参数。
import multiprocessing
def func1():
print("Function 1")
def func2():
print("Function 2")
process1 = multiprocessing.Process(target=func1)
process2 = multiprocessing.Process(target=func2)
process1.start()
process2.start()
process1.join()
process2.join()
在上面的代码中,process1
和process2
是两个进程,它们分别执行func1
和func2
。通过调用start()
方法,进程开始执行。join()
方法确保主进程等待子进程执行完毕。
2. 使用进程执行CPU密集型任务:
进程适用于CPU密集型任务,例如计算密集型操作。以下是一个示例,展示了如何使用进程来并发执行两个计算密集型任务:
import multiprocessing
def compute_square(numbers):
result = [n 2 for n in numbers]
print(f"Squares: {result}")
def compute_cube(numbers):
result = [n 3 for n in numbers]
print(f"Cubes: {result}")
numbers = [1, 2, 3, 4, 5]
process1 = multiprocessing.Process(target=compute_square, args=(numbers,))
process2 = multiprocessing.Process(target=compute_cube, args=(numbers,))
process1.start()
process2.start()
process1.join()
process2.join()
三、异步编程(Asyncio)
Python的asyncio
模块提供了一个简单的方法来创建和管理异步任务。异步编程适用于处理大量并发的异步I/O操作。
1. 使用异步函数:
要创建异步函数,你需要在函数定义前加上async
关键字,并使用await
关键字等待异步操作。
import asyncio
async def func1():
print("Function 1")
async def func2():
print("Function 2")
async def main():
task1 = asyncio.create_task(func1())
task2 = asyncio.create_task(func2())
await task1
await task2
asyncio.run(main())
在上面的代码中,func1
和func2
是异步函数,它们分别执行。通过调用asyncio.create_task()
方法,创建异步任务。await
关键字用于等待任务完成。
2. 使用异步编程执行异步I/O操作:
异步编程适用于处理大量并发的异步I/O操作,例如网络请求。以下是一个示例,展示了如何使用异步编程来并发执行两个网络请求:
import asyncio
import aiohttp
async def fetch_url(session, url):
async with session.get(url) as response:
print(f"Fetched {url} with status code {response.status}")
async def main():
async with aiohttp.ClientSession() as session:
url1 = "http://example.com"
url2 = "http://example.org"
task1 = asyncio.create_task(fetch_url(session, url1))
task2 = asyncio.create_task(fetch_url(session, url2))
await task1
await task2
asyncio.run(main())
四、总结
Python可以通过多线程、多进程、异步编程等方式来同时执行两个函数。多线程适用于I/O密集型任务,多进程适用于CPU密集型任务,异步编程适用于处理大量并发的异步I/O操作。选择合适的方法取决于具体的任务需求和应用场景。
相关问答FAQs:
如何在Python中实现多线程来同时执行两个函数?
在Python中,可以使用threading
模块来实现多线程,从而实现同时执行多个函数。首先,您需要定义要并行执行的函数,然后创建线程对象,并启动这些线程。例如:
import threading
def function_one():
print("Function One is executing.")
def function_two():
print("Function Two is executing.")
# 创建线程
thread1 = threading.Thread(target=function_one)
thread2 = threading.Thread(target=function_two)
# 启动线程
thread1.start()
thread2.start()
# 等待线程完成
thread1.join()
thread2.join()
这样,function_one
和function_two
就会同时执行。
在Python中使用异步编程实现函数并发执行的方式是什么?
异步编程是另一种实现函数并发执行的方式。使用asyncio
库可以让你定义异步函数并使用事件循环来调度它们。以下是一个简单的示例:
import asyncio
async def function_one():
print("Function One is executing.")
async def function_two():
print("Function Two is executing.")
async def main():
await asyncio.gather(function_one(), function_two())
# 运行事件循环
asyncio.run(main())
在这个例子中,function_one
和function_two
将被并发执行。
在执行两个函数时,是否会影响程序的性能和响应速度?
执行多个函数会占用更多的系统资源,特别是在使用多线程或异步编程时。如果这两个函数是IO密集型操作,如文件读取或网络请求,使用异步编程可以显著提高程序的响应速度。然而,如果函数是CPU密集型的,使用多线程可能不会带来明显的性能提升,因为Python的全局解释器锁(GIL)限制了多线程的并发性能。在这种情况下,考虑使用multiprocessing
模块来充分利用多核CPU资源。
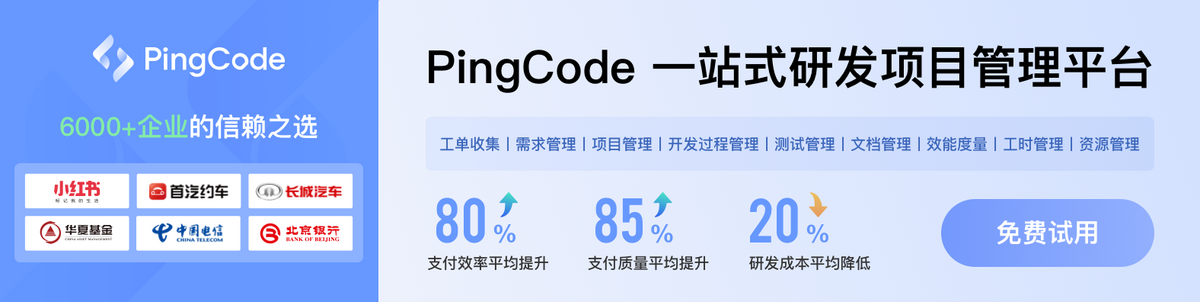