在Python中把坐标数据赋值给代码的方法有多种,包括直接赋值、使用列表或元组、使用字典,以及使用自定义类。其中,使用列表和元组是最常见的方式。接下来我们将详细介绍这些方法,并展示如何在代码中使用它们。
一、直接赋值
最简单的方法就是直接将坐标数据赋值给变量。这种方法适用于坐标数量较少且结构简单的情况。
x = 10
y = 20
z = 30
print(f"Coordinates: ({x}, {y}, {z})")
二、使用列表或元组
列表和元组是Python中常用的数据结构,可以用来存储多个坐标值。列表是可变的,而元组是不可变的。
使用列表
coordinates = [10, 20, 30]
访问坐标数据
x = coordinates[0]
y = coordinates[1]
z = coordinates[2]
print(f"Coordinates: ({x}, {y}, {z})")
使用元组
coordinates = (10, 20, 30)
访问坐标数据
x, y, z = coordinates
print(f"Coordinates: ({x}, {y}, {z})")
三、使用字典
字典是一种键值对的数据结构,可以用来存储带有标签的坐标数据。
coordinates = {'x': 10, 'y': 20, 'z': 30}
访问坐标数据
x = coordinates['x']
y = coordinates['y']
z = coordinates['z']
print(f"Coordinates: ({x}, {y}, {z})")
四、使用自定义类
如果你需要对坐标数据进行更复杂的操作,可以定义一个自定义类来存储和处理坐标数据。
class Coordinates:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __str__(self):
return f"Coordinates: ({self.x}, {self.y}, {self.z})"
创建坐标对象
coords = Coordinates(10, 20, 30)
print(coords)
五、更多坐标操作
除了赋值之外,处理坐标数据常常需要进行各种操作,如计算距离、变换坐标系等。以下是一些常见的坐标操作示例。
计算两点之间的距离
import math
def calculate_distance(coord1, coord2):
return math.sqrt((coord1[0] - coord2[0]) <strong> 2 + (coord1[1] - coord2[1]) </strong> 2 + (coord1[2] - coord2[2]) 2)
point1 = (10, 20, 30)
point2 = (40, 50, 60)
distance = calculate_distance(point1, point2)
print(f"Distance between points: {distance}")
坐标变换
def translate_coordinates(coord, translation):
return (coord[0] + translation[0], coord[1] + translation[1], coord[2] + translation[2])
point = (10, 20, 30)
translation = (5, -5, 10)
new_point = translate_coordinates(point, translation)
print(f"New coordinates after translation: {new_point}")
六、将坐标数据存储在文件中
在实际应用中,坐标数据通常需要存储在文件中,以便后续读取和处理。以下是如何将坐标数据存储在文本文件和CSV文件中的示例。
存储在文本文件中
coordinates = [(10, 20, 30), (40, 50, 60), (70, 80, 90)]
with open('coordinates.txt', 'w') as file:
for coord in coordinates:
file.write(f"{coord[0]} {coord[1]} {coord[2]}\n")
print("Coordinates saved to coordinates.txt")
存储在CSV文件中
import csv
coordinates = [(10, 20, 30), (40, 50, 60), (70, 80, 90)]
with open('coordinates.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['x', 'y', 'z'])
for coord in coordinates:
writer.writerow(coord)
print("Coordinates saved to coordinates.csv")
七、从文件中读取坐标数据
从文本文件读取
coordinates = []
with open('coordinates.txt', 'r') as file:
for line in file:
x, y, z = map(int, line.split())
coordinates.append((x, y, z))
print(f"Coordinates read from file: {coordinates}")
从CSV文件读取
import csv
coordinates = []
with open('coordinates.csv', 'r') as file:
reader = csv.reader(file)
next(reader) # Skip header row
for row in reader:
x, y, z = map(int, row)
coordinates.append((x, y, z))
print(f"Coordinates read from file: {coordinates}")
八、使用第三方库处理坐标数据
在实际应用中,使用第三方库可以简化坐标数据的处理。以下是如何使用NumPy和Pandas处理坐标数据的示例。
使用NumPy
import numpy as np
coordinates = np.array([[10, 20, 30], [40, 50, 60], [70, 80, 90]])
访问坐标数据
print(f"First coordinate: {coordinates[0]}")
print(f"X-values: {coordinates[:, 0]}")
使用Pandas
import pandas as pd
data = {'x': [10, 20, 30], 'y': [40, 50, 60], 'z': [70, 80, 90]}
df = pd.DataFrame(data)
访问坐标数据
print(df)
print(f"First row: {df.iloc[0]}")
print(f"X-values: {df['x']}")
九、可视化坐标数据
可视化是处理坐标数据的重要部分,它可以帮助我们更直观地理解数据。以下是使用Matplotlib库进行坐标数据可视化的示例。
绘制2D坐标数据
import matplotlib.pyplot as plt
coordinates = [(10, 20), (30, 40), (50, 60)]
x_values = [coord[0] for coord in coordinates]
y_values = [coord[1] for coord in coordinates]
plt.scatter(x_values, y_values)
plt.xlabel('X')
plt.ylabel('Y')
plt.title('2D Coordinates')
plt.show()
绘制3D坐标数据
from mpl_toolkits.mplot3d import Axes3D
coordinates = [(10, 20, 30), (40, 50, 60), (70, 80, 90)]
x_values = [coord[0] for coord in coordinates]
y_values = [coord[1] for coord in coordinates]
z_values = [coord[2] for coord in coordinates]
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(x_values, y_values, z_values)
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
plt.title('3D Coordinates')
plt.show()
十、总结
在Python中处理坐标数据的方法多种多样,从简单的直接赋值到使用复杂的自定义类和第三方库,都能满足不同需求。使用列表、元组、字典等内置数据结构可以快速上手,而使用NumPy、Pandas等第三方库则能提供更强大的功能和更高的效率。无论选择哪种方法,都需要根据具体应用场景选择最合适的方式。希望本文能为你在Python中处理坐标数据提供一些有用的参考和指导。
相关问答FAQs:
如何在Python中处理坐标数据并赋值?
在Python中,可以使用列表、元组或字典等数据结构来存储坐标数据。例如,使用列表可以将坐标点表示为coordinates = [(x1, y1), (x2, y2), ...]
。如果需要更复杂的结构,可以使用字典,例如coordinates = {'point1': (x1, y1), 'point2': (x2, y2)}
。这样可以方便地访问和管理坐标数据。
有什么库可以帮助我更方便地处理坐标数据?
Python提供了一些优秀的库来处理坐标数据,例如NumPy和Pandas。NumPy可以用来进行高效的数值计算,适合处理大规模的坐标数据。Pandas则适合对表格数据进行操作,通过DataFrame可以轻松处理坐标数据的增删改查。
如何将坐标数据从文件中读取并赋值到Python变量?
可以使用Python的内置文件操作功能来读取坐标数据。如果坐标数据存储在文本文件中,可以使用open()
函数打开文件,然后使用readlines()
或read()
方法读取数据。将读取到的字符串进行分割和转换后,就可以赋值给Python变量,例如coordinates = [tuple(map(float, line.split(','))) for line in file.readlines()]
。这样可以将每一行的坐标数据转换为浮点数并存入列表中。
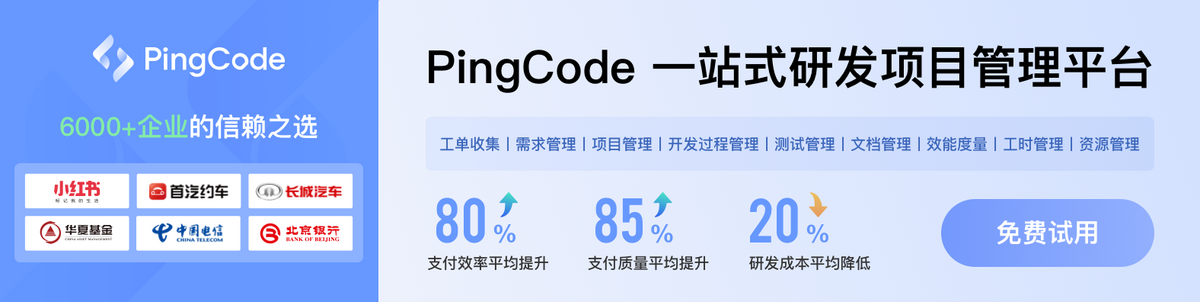