Python窗口之间的跳转可以通过使用GUI库如Tkinter、PyQt、Kivy来实现,通过在不同窗口之间切换、隐藏和显示窗口来实现跳转。其中,Tkinter是Python的标准GUI库,简单易用,适合初学者;PyQt功能强大,适合复杂的GUI应用开发;Kivy适合开发跨平台应用。 下面我们将详细介绍如何使用Tkinter来实现窗口之间的跳转。
一、Tkinter实现窗口跳转
Tkinter是Python内置的标准GUI库,适用于快速开发桌面应用。我们可以使用Tkinter创建多个窗口,并通过按钮触发事件来实现窗口之间的跳转。
1、创建主窗口和子窗口
首先,我们创建一个主窗口和一个子窗口,主窗口包含一个按钮,当点击按钮时,打开子窗口。
import tkinter as tk
def open_child_window():
child_window = tk.Toplevel(root)
child_window.title("子窗口")
label = tk.Label(child_window, text="这是子窗口")
label.pack()
root = tk.Tk()
root.title("主窗口")
button = tk.Button(root, text="打开子窗口", command=open_child_window)
button.pack()
root.mainloop()
在上述代码中,我们定义了一个函数open_child_window
,该函数创建一个新的顶级窗口(子窗口),并在其中放置一个标签。主窗口包含一个按钮,当点击按钮时,调用open_child_window
函数,从而打开子窗口。
2、在多个窗口之间跳转
为了在多个窗口之间跳转,我们可以在每个窗口中放置按钮来关闭当前窗口并打开另一个窗口。
import tkinter as tk
def open_window_a():
window_a = tk.Toplevel(root)
window_a.title("窗口 A")
button = tk.Button(window_a, text="打开窗口 B", command=lambda: open_window_b(window_a))
button.pack()
def open_window_b(previous_window):
previous_window.destroy()
window_b = tk.Toplevel(root)
window_b.title("窗口 B")
button = tk.Button(window_b, text="回到主窗口", command=lambda: open_main_window(window_b))
button.pack()
def open_main_window(previous_window):
previous_window.destroy()
root.deiconify()
root = tk.Tk()
root.title("主窗口")
button = tk.Button(root, text="打开窗口 A", command=lambda: [root.withdraw(), open_window_a()])
button.pack()
root.mainloop()
在上述代码中,我们定义了三个函数:open_window_a
,open_window_b
和open_main_window
,分别用于打开窗口A、窗口B和回到主窗口。在每个函数中,我们先关闭当前窗口,然后打开下一个窗口。
二、PyQt实现窗口跳转
PyQt是一个功能强大的GUI库,适用于开发复杂的桌面应用。我们可以使用PyQt创建多个窗口,并通过信号和槽机制来实现窗口之间的跳转。
1、创建主窗口和子窗口
首先,我们创建一个主窗口和一个子窗口,主窗口包含一个按钮,当点击按钮时,打开子窗口。
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QWidget, QVBoxLayout
class ChildWindow(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("子窗口")
layout = QVBoxLayout()
layout.addWidget(QPushButton("这是子窗口"))
self.setLayout(layout)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("主窗口")
button = QPushButton("打开子窗口")
button.clicked.connect(self.open_child_window)
self.setCentralWidget(button)
def open_child_window(self):
self.child_window = ChildWindow()
self.child_window.show()
app = QApplication(sys.argv)
main_window = MainWindow()
main_window.show()
sys.exit(app.exec_())
在上述代码中,我们定义了一个ChildWindow
类和一个MainWindow
类。MainWindow
类包含一个按钮,当点击按钮时,创建并显示一个ChildWindow
实例。
2、在多个窗口之间跳转
为了在多个窗口之间跳转,我们可以在每个窗口中放置按钮,通过信号和槽机制来关闭当前窗口并打开另一个窗口。
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QWidget, QVBoxLayout
class WindowA(QWidget):
def __init__(self, main_window):
super().__init__()
self.main_window = main_window
self.setWindowTitle("窗口 A")
layout = QVBoxLayout()
button = QPushButton("打开窗口 B")
button.clicked.connect(self.open_window_b)
layout.addWidget(button)
self.setLayout(layout)
def open_window_b(self):
self.close()
self.window_b = WindowB(self.main_window)
self.window_b.show()
class WindowB(QWidget):
def __init__(self, main_window):
super().__init__()
self.main_window = main_window
self.setWindowTitle("窗口 B")
layout = QVBoxLayout()
button = QPushButton("回到主窗口")
button.clicked.connect(self.open_main_window)
layout.addWidget(button)
self.setLayout(layout)
def open_main_window(self):
self.close()
self.main_window.show()
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("主窗口")
button = QPushButton("打开窗口 A")
button.clicked.connect(self.open_window_a)
self.setCentralWidget(button)
def open_window_a(self):
self.hide()
self.window_a = WindowA(self)
self.window_a.show()
app = QApplication(sys.argv)
main_window = MainWindow()
main_window.show()
sys.exit(app.exec_())
在上述代码中,我们定义了WindowA
和WindowB
类,分别表示窗口A和窗口B。在每个类中,我们定义了一个按钮,通过点击按钮来关闭当前窗口并打开下一个窗口。
三、Kivy实现窗口跳转
Kivy是一个用于开发多点触控应用程序的开源Python库,适用于开发跨平台应用。我们可以使用Kivy创建多个窗口,并通过屏幕管理器来实现窗口之间的跳转。
1、创建主窗口和子窗口
首先,我们创建一个主窗口和一个子窗口,主窗口包含一个按钮,当点击按钮时,打开子窗口。
from kivy.app import App
from kivy.uix.screenmanager import ScreenManager, Screen
from kivy.uix.button import Button
class MainWindow(Screen):
def __init__(self, kwargs):
super(MainWindow, self).__init__(kwargs)
button = Button(text="打开子窗口")
button.bind(on_release=self.open_child_window)
self.add_widget(button)
def open_child_window(self, instance):
self.manager.current = 'child'
class ChildWindow(Screen):
def __init__(self, kwargs):
super(ChildWindow, self).__init__(kwargs)
button = Button(text="这是子窗口")
self.add_widget(button)
class MyApp(App):
def build(self):
sm = ScreenManager()
sm.add_widget(MainWindow(name='main'))
sm.add_widget(ChildWindow(name='child'))
return sm
if __name__ == '__main__':
MyApp().run()
在上述代码中,我们定义了MainWindow
和ChildWindow
类,分别表示主窗口和子窗口。使用ScreenManager
来管理和切换屏幕。
2、在多个窗口之间跳转
为了在多个窗口之间跳转,我们可以在每个窗口中放置按钮,通过屏幕管理器来切换屏幕。
from kivy.app import App
from kivy.uix.screenmanager import ScreenManager, Screen
from kivy.uix.button import Button
class WindowA(Screen):
def __init__(self, kwargs):
super(WindowA, self).__init__(kwargs)
button = Button(text="打开窗口 B")
button.bind(on_release=self.open_window_b)
self.add_widget(button)
def open_window_b(self, instance):
self.manager.current = 'window_b'
class WindowB(Screen):
def __init__(self, kwargs):
super(WindowB, self).__init__(kwargs)
button = Button(text="回到主窗口")
button.bind(on_release=self.open_main_window)
self.add_widget(button)
def open_main_window(self, instance):
self.manager.current = 'main'
class MainWindow(Screen):
def __init__(self, kwargs):
super(MainWindow, self).__init__(kwargs)
button = Button(text="打开窗口 A")
button.bind(on_release=self.open_window_a)
self.add_widget(button)
def open_window_a(self, instance):
self.manager.current = 'window_a'
class MyApp(App):
def build(self):
sm = ScreenManager()
sm.add_widget(MainWindow(name='main'))
sm.add_widget(WindowA(name='window_a'))
sm.add_widget(WindowB(name='window_b'))
return sm
if __name__ == '__main__':
MyApp().run()
在上述代码中,我们定义了WindowA
和WindowB
类,分别表示窗口A和窗口B。使用ScreenManager
来管理和切换屏幕,通过按钮绑定的事件来切换屏幕。
总结
在Python中,实现窗口之间的跳转可以使用不同的GUI库,如Tkinter、PyQt和Kivy。每个库都有其独特的特点和适用场景。通过上述示例代码,我们可以掌握如何使用这些库来创建多个窗口,并实现窗口之间的跳转。根据具体需求和应用场景,选择合适的库来开发你的桌面应用。
相关问答FAQs:
在Python中,如何创建多个窗口并实现窗口间的跳转?
可以使用Tkinter库来创建多个窗口。在Tkinter中,每个窗口都是一个独立的Toplevel对象。您可以通过调用不同窗口的withdraw()
和deiconify()
方法来实现窗口间的跳转。比如,您可以在一个窗口中按下按钮后隐藏当前窗口,并显示另一个窗口。
在使用Tkinter时,有哪些技巧可以提高窗口间跳转的用户体验?
在窗口间跳转时,可以添加动画效果或使用过渡效果来提升用户体验。通过设置窗口的透明度或使用geometry()
方法改变窗口尺寸可以使用户感受到更加流畅的界面切换。此外,合理安排按钮的位置和功能,使用户能够快速找到所需的操作,也非常重要。
如何在不同的窗口之间传递数据?
在多个窗口间传递数据可以通过多种方式实现。可以在创建新窗口时,将数据作为参数传递给窗口的初始化函数,或者使用全局变量保存数据。在Tkinter中,也可以使用StringVar
等变量类来实现数据绑定,确保数据在窗口间的同步更新。
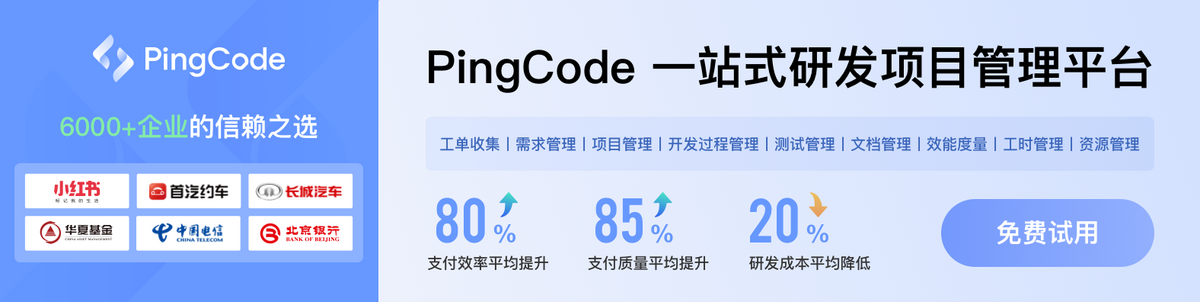