Python如何定义一个循环数,可以使用while循环、for循环、递归等方法,下面将详细描述如何使用这些方法来定义一个循环数。
Python 提供了多种循环的方式,其中最常用的是 while
循环和 for
循环。使用这些循环结构可以轻松地定义和处理循环数。接下来,我们将详细介绍如何使用 while
循环和 for
循环来定义一个循环数,并会涉及到递归方法。
一、使用 while
循环定义循环数
while
循环是 Python 中的一种基本循环结构,它会重复执行一个代码块,直到条件不再满足为止。使用 while
循环可以很方便地定义一个循环数。
示例1:使用 while
循环定义循环数
def while_loop_example(n):
count = 0
while count < n:
print(f"Current count is: {count}")
count += 1
调用函数
while_loop_example(5)
在这个例子中,函数 while_loop_example
接收一个参数 n
,并使用 while
循环从 0 计数到 n-1。每次循环,都会打印当前计数值,直到 count
等于 n
。
示例2:使用 while
循环实现无限循环
有时候我们需要一个无限循环,直到满足某个退出条件。在这种情况下,我们可以使用 while True
结构。
def infinite_while_loop():
count = 0
while True:
print(f"Current count is: {count}")
count += 1
if count >= 5:
break
调用函数
infinite_while_loop()
在这个例子中,while True
创建了一个无限循环,直到 count
达到 5 时退出循环。
二、使用 for
循环定义循环数
for
循环是 Python 中的另一种基本循环结构,通常用于迭代序列(如列表、元组、字符串)或其他可迭代对象。使用 for
循环也可以方便地定义一个循环数。
示例1:使用 for
循环定义循环数
def for_loop_example(n):
for count in range(n):
print(f"Current count is: {count}")
调用函数
for_loop_example(5)
在这个例子中,函数 for_loop_example
使用 for
循环迭代从 0 到 n-1 的范围,并在每次循环中打印当前计数值。
示例2:使用 for
循环遍历列表
def iterate_list_example(my_list):
for item in my_list:
print(f"Current item is: {item}")
调用函数
iterate_list_example([1, 2, 3, 4, 5])
在这个例子中,函数 iterate_list_example
使用 for
循环遍历一个列表,并在每次循环中打印当前列表项。
三、使用递归定义循环数
递归是一种函数调用自身的编程技巧。在某些情况下,使用递归也可以实现循环数的定义。
示例1:使用递归实现循环数
def recursive_example(n, count=0):
if count < n:
print(f"Current count is: {count}")
recursive_example(n, count + 1)
调用函数
recursive_example(5)
在这个例子中,函数 recursive_example
使用递归方式实现了循环数的定义。函数接受两个参数 n
和 count
,如果 count
小于 n
,则打印当前计数值并递归调用自身,直到 count
等于 n
。
四、综合应用
下面是一个综合应用的例子,展示了如何结合 while
循环和 for
循环来定义和处理循环数。
示例:结合使用 while
和 for
循环
def combined_loops_example(n):
count = 0
while count < n:
print(f"While loop - current count is: {count}")
count += 1
print("Switching to for loop")
for count in range(n):
print(f"For loop - current count is: {count}")
调用函数
combined_loops_example(5)
在这个例子中,函数 combined_loops_example
首先使用 while
循环从 0 计数到 n-1,然后切换到 for
循环从 0 计数到 n-1。
示例:结合使用递归和 for
循环
def recursive_and_for_loop_example(n):
def recursive_part(count):
if count < n:
print(f"Recursive part - current count is: {count}")
recursive_part(count + 1)
recursive_part(0)
print("Switching to for loop")
for count in range(n):
print(f"For loop - current count is: {count}")
调用函数
recursive_and_for_loop_example(5)
在这个例子中,函数 recursive_and_for_loop_example
首先使用递归从 0 计数到 n-1,然后切换到 for
循环从 0 计数到 n-1。
通过以上示例,我们展示了如何使用 while
循环、for
循环和递归来定义循环数。根据实际需求选择合适的循环方式,可以帮助我们更高效地解决问题。
相关问答FAQs:
循环数是什么,如何在Python中定义它?
循环数是指一个数字,其各个数字的排列可以形成一个循环的数字序列。要在Python中定义一个循环数,首先需要将数字转换为字符串,然后检查其各个数字的排列组合是否能形成一个有效的循环。可以通过使用字符串切片和循环来实现这一点。
在Python中如何实现循环的逻辑?
在Python中,可以使用for
循环或while
循环来实现循环的逻辑。for
循环适用于已知次数的循环,而while
循环则适用于需要根据条件不断重复的情况。为了实现循环数的检查,可以将数字转为字符串并使用循环来比较各个排列。
如何提高循环数的识别效率?
若要提高循环数的识别效率,可以使用集合来存储已识别的循环数,以减少重复计算。此外,使用数学方法,例如数字的最大公约数,也可以帮助快速判断两个数字是否具有循环关系。对于较大的数字,可以考虑优化算法以减少计算时间。
在Python中有哪些常见的循环数的应用场景?
循环数在密码学、数论以及某些算法的优化中都有应用。在数据分析和处理时,识别循环数可以帮助检测周期性数据模式。在游戏开发中,循环数也可以用于生成独特的角色或关卡设计。
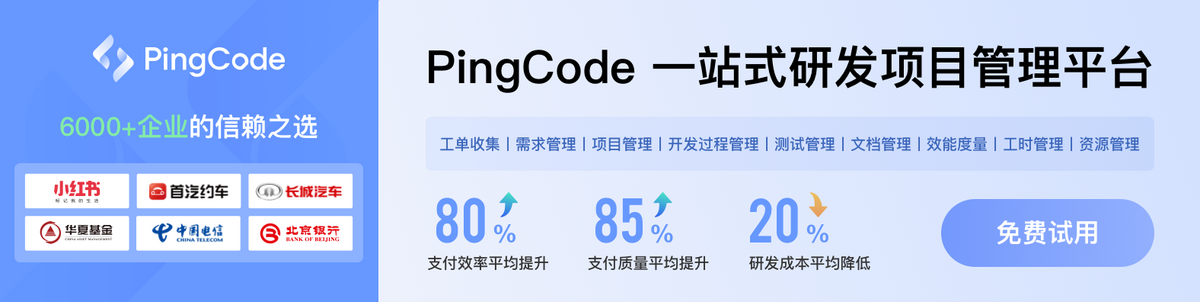