在Python中,可以使用内置的print函数、pprint模块、json模块、以及第三方库如PrettyTable和tabulate来格式化输出字典。其中,使用内置print函数和pprint模块是最常见的方法。pprint模块提供了一种简单且可读性高的方式来输出复杂的字典对象。
一、使用内置的print函数
内置的print函数是最基本的输出方式。可以使用print函数结合f-string、str()或repr()等方法来格式化输出字典。
1、使用f-string
f-string是Python 3.6引入的格式化字符串的方式,能够使代码更简洁易读。
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
print(f"My dictionary: {my_dict}")
这种方法简单直接,但对于复杂嵌套的字典结构,其可读性较差。
2、使用str()或repr()
使用str()或repr()方法可以将字典转换为字符串进行输出。
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
print("My dictionary: " + str(my_dict))
print("My dictionary: " + repr(my_dict))
str()方法输出更友好,而repr()方法输出更接近原始数据格式。
二、使用pprint模块
pprint模块是Python内置模块,专门用于格式化复杂的嵌套数据结构。
1、pprint函数
pprint函数可以自动调整输出格式,使其更易读。
import pprint
my_dict = {"name": "Alice", "age": 25, "city": "New York", "hobbies": ["reading", "hiking", "coding"]}
pprint.pprint(my_dict)
这种方法对于复杂的嵌套字典非常有效,输出结果整齐、美观。
2、PrettyPrinter类
PrettyPrinter类提供了更多的自定义选项,比如指定缩进、宽度等。
from pprint import PrettyPrinter
my_dict = {"name": "Alice", "age": 25, "city": "New York", "hobbies": ["reading", "hiking", "coding"]}
pp = PrettyPrinter(indent=4)
pp.pprint(my_dict)
这种方法可以根据需求调整输出格式,使其更加灵活。
三、使用json模块
json模块可以将字典转换为JSON格式字符串进行输出,这种方法输出的结果更加结构化。
1、json.dumps函数
json.dumps函数可以将字典转换为JSON格式字符串,并可以指定缩进级别。
import json
my_dict = {"name": "Alice", "age": 25, "city": "New York", "hobbies": ["reading", "hiking", "coding"]}
json_str = json.dumps(my_dict, indent=4)
print(json_str)
这种方法输出的结果层次分明,易于阅读。
四、使用第三方库
除了内置模块,还可以使用一些第三方库来格式化输出字典,比如PrettyTable和tabulate。
1、PrettyTable
PrettyTable库可以用来生成美观的表格,适用于字典的表格化输出。
from prettytable import PrettyTable
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
table = PrettyTable()
table.field_names = ["Key", "Value"]
for key, value in my_dict.items():
table.add_row([key, value])
print(table)
这种方法适合将字典输出为表格格式,使得数据更加直观。
2、tabulate
tabulate库提供了多种表格格式,可以方便地将字典转换为表格输出。
from tabulate import tabulate
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
table = tabulate(my_dict.items(), headers=["Key", "Value"])
print(table)
这种方法同样适合将字典输出为表格格式,并且可以选择不同的表格样式。
总结
在Python中,字典格式化输出的方法有很多种,可以根据实际需求选择合适的方法。内置的print函数、pprint模块、json模块、以及第三方库如PrettyTable和tabulate都可以实现字典的格式化输出。对于一般情况,使用pprint模块是最简单高效的方式。对于需要结构化输出的情况,可以使用json模块。而对于需要表格化输出的情况,可以使用PrettyTable或tabulate库。
相关问答FAQs:
如何在Python中对字典进行美化输出?
在Python中,可以使用json
模块中的dumps
方法来美化字典的输出。通过设置indent
参数,可以使输出的字典更易读。例如:
import json
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
formatted_output = json.dumps(my_dict, indent=4)
print(formatted_output)
这段代码将输出一个格式化的字典,其中每个键值对都在新的一行,并且缩进清晰。
Python中有哪些其他方法可以格式化字典输出?
除了使用json
模块,Python的pprint
模块也可以帮助格式化输出字典。pprint
提供了一个简单的方式来输出复杂的嵌套结构,使其更加易于理解。示例如下:
from pprint import pprint
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York', 'hobbies': ['reading', 'traveling']}
pprint(my_dict)
这将以更具可读性的方式输出字典,尤其是在处理嵌套字典或列表时,非常有效。
在字典中如何格式化特定键的输出?
如果需要对字典中特定键的值进行格式化输出,可以使用字符串格式化方法。例如,可以提取特定键的值,并以自定义格式打印:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
print(f"Name: {my_dict['name']}, Age: {my_dict['age']}, City: {my_dict['city']}")
这种方式灵活性高,可以根据需要调整输出格式,使其更加符合特定场景的需求。
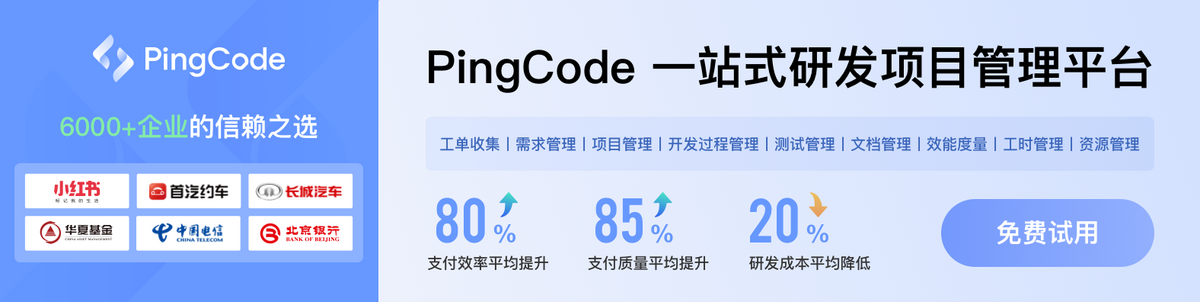