在Python3中,让输入为空的方法有:使用默认值、使用条件判断、使用try-except块。 详细方法如下:
- 使用默认值:在函数参数中设置默认值,如果用户未提供输入,则使用默认值。
- 使用条件判断:检查输入是否为空,如果为空则执行相应的操作。
- 使用try-except块:捕获异常来处理空输入的情况。
接下来,我们将详细介绍每种方法的实现方式,并提供具体的代码示例和应用场景。
一、使用默认值
使用默认值是最简单的方法之一。在定义函数时,可以为参数设置一个默认值。当函数被调用而没有提供该参数时,默认值将被使用。
def greet(name=""):
if name:
print(f"Hello, {name}!")
else:
print("Hello, World!")
greet() # 输出: Hello, World!
greet("Alice") # 输出: Hello, Alice!
在上面的代码中,name
参数具有默认值""
(空字符串)。如果调用greet
函数时没有提供name
参数,函数会使用默认值并输出“Hello, World!”。
二、使用条件判断
在处理用户输入时,可以使用条件判断来检查输入是否为空。如果输入为空,则执行特定操作。
name = input("Enter your name: ").strip()
if not name:
print("You did not enter a name.")
else:
print(f"Hello, {name}!")
在这个示例中,使用了strip()
方法去除输入两端的空白字符,然后通过if not name
来判断输入是否为空。如果输入为空,程序将提示用户未输入名称。
三、使用try-except块
在某些情况下,捕获异常可以帮助我们处理空输入的情况。例如,处理整数输入时,可以使用try-except
块来捕获输入为空或输入非整数时的异常。
try:
age = int(input("Enter your age: ").strip())
print(f"You are {age} years old.")
except ValueError:
print("Invalid input. Please enter a valid age.")
在这个示例中,int()
函数尝试将用户输入转换为整数。如果输入为空或输入的不是整数,将引发ValueError
异常,并执行except
块中的代码,提示用户输入无效。
四、结合多种方法
在实际应用中,可能需要结合多种方法来处理复杂的输入场景。下面是一个综合示例,展示了如何处理多个输入字段,并使用默认值、条件判断和try-except
块来确保输入的有效性。
def get_user_info():
name = input("Enter your name (default: 'Guest'): ").strip() or "Guest"
try:
age = int(input("Enter your age: ").strip())
except ValueError:
age = None
if age is None:
print(f"Hello, {name}! Your age is not provided.")
else:
print(f"Hello, {name}! You are {age} years old.")
get_user_info()
在这个示例中,name
字段使用默认值"Guest"
,而age
字段使用try-except
块来处理可能的异常。如果用户未提供年龄或输入无效,程序将提示年龄未提供。
五、处理更多复杂输入
在一些复杂的输入场景中,可能需要更加详细的输入验证和处理逻辑。例如,处理多个输入字段并确保每个字段都符合特定的条件。下面是一个示例,展示了如何处理多个字段的输入,并进行详细的验证和处理。
def get_user_info():
name = input("Enter your name (default: 'Guest'): ").strip() or "Guest"
while True:
age_input = input("Enter your age: ").strip()
if not age_input:
print("Age cannot be empty. Please enter a valid age.")
continue
try:
age = int(age_input)
if age < 0:
print("Age cannot be negative. Please enter a valid age.")
continue
break
except ValueError:
print("Invalid input. Please enter a valid age.")
email = input("Enter your email (optional): ").strip()
if not email:
email = None
print(f"Hello, {name}!")
print(f"Age: {age}")
if email:
print(f"Email: {email}")
else:
print("Email: Not provided")
get_user_info()
在这个示例中,age
字段使用了一个while
循环来确保输入有效。如果输入为空、负数或无效,将提示用户重新输入。email
字段是可选的,如果用户未提供,则设置为None
。
六、总结
在Python3中,处理空输入的方法有多种,包括使用默认值、条件判断和try-except
块。根据具体的应用场景,可以选择适合的方法来确保输入的有效性。在复杂的输入场景中,可能需要结合多种方法来处理不同类型的输入和进行详细的验证。
通过这些方法,可以有效地处理用户输入,确保程序的健壮性和用户体验的提升。在实际开发中,应根据具体需求选择最适合的方法,并进行充分的测试,确保程序能够正确处理各种输入情况。
相关问答FAQs:
如何在Python3中判断用户输入是否为空?
在Python3中,可以使用input()
函数获取用户输入,并通过简单的条件判断来检查输入是否为空。具体来说,可以将用户输入的结果与空字符串进行比较,例如:
user_input = input("请输入内容: ")
if user_input == "":
print("您没有输入任何内容。")
通过这种方式,可以轻松判断用户是否提供了有效的输入。
如何处理Python3中空输入的异常情况?
在处理用户输入时,空输入可能会导致程序出现意想不到的行为。为了增强程序的健壮性,可以加入异常处理机制。在获取输入后,可以使用try
和except
块来捕获可能的错误情况,例如:
try:
user_input = input("请输入内容: ")
if not user_input.strip():
raise ValueError("输入不能为空。")
except ValueError as e:
print(e)
这样,当用户输入为空或只包含空格时,程序能够优雅地处理这种情况,而不会崩溃。
在Python3中如何设置输入默认值以避免空输入?
为了确保用户不会提交空输入,可以在input()
函数中设置一个默认值。例如,如果用户直接按回车键,可以使用一个条件语句为输入提供默认值:
user_input = input("请输入内容 (默认值为 '默认输入'): ") or '默认输入'
print(f"您输入的内容是: {user_input}")
这种方法不仅可以避免空输入,还能提供更友好的用户体验。
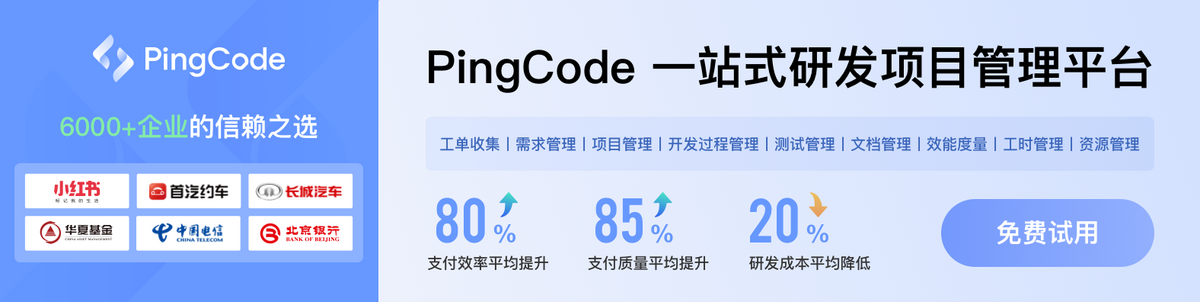