用Python查找指定字符并计数的方法有多种:使用字符串方法、正则表达式、列表解析等。 在本文中,我们将详细讨论如何使用这些方法来查找和计数字符串中的指定字符,并提供具体的代码示例。
一、字符串方法
Python字符串方法提供了一些简单且高效的方式来查找和计数字符。以下是一些常用的方法:
count()
方法- 使用循环和条件语句
find()
和rfind()
方法
1. count()
方法
count()
方法是查找和计数字符串中特定字符的最直接和简单的方法。它返回子字符串在字符串中出现的次数。
text = "Hello, World! Hello, Python!"
char = "o"
count = text.count(char)
print(f"The character '{char}' appears {count} times.")
2. 使用循环和条件语句
这种方法涉及遍历字符串的每个字符,并使用条件语句检查字符是否匹配。
text = "Hello, World! Hello, Python!"
char = "o"
count = 0
for c in text:
if c == char:
count += 1
print(f"The character '{char}' appears {count} times.")
3. find()
和 rfind()
方法
find()
方法返回子字符串在字符串中第一次出现的索引,如果子字符串不在字符串中,则返回 -1。rfind()
方法从右侧开始查找。
text = "Hello, World! Hello, Python!"
char = "o"
index = text.find(char)
count = 0
while index != -1:
count += 1
index = text.find(char, index + 1)
print(f"The character '{char}' appears {count} times.")
二、正则表达式
正则表达式提供了一种强大且灵活的方法来查找和计数字符串中的特定字符或模式。
使用 re
模块
Python 的 re
模块提供了各种功能强大的正则表达式操作。
import re
text = "Hello, World! Hello, Python!"
char = "o"
pattern = re.compile(char)
matches = pattern.findall(text)
count = len(matches)
print(f"The character '{char}' appears {count} times.")
三、列表解析
列表解析是一种简洁且高效的方式来遍历字符串并计数特定字符。
使用列表解析计数
text = "Hello, World! Hello, Python!"
char = "o"
count = sum([1 for c in text if c == char])
print(f"The character '{char}' appears {count} times.")
四、结合多种方法
在实际应用中,有时需要结合多种方法来实现更复杂的需求。例如,查找多个字符或模式,并统计其出现次数。
查找多个字符
text = "Hello, World! Hello, Python!"
chars = "lo"
counts = {char: text.count(char) for char in chars}
for char, count in counts.items():
print(f"The character '{char}' appears {count} times.")
查找特定模式
import re
text = "Hello, World! Hello, Python!"
pattern = re.compile(r"o.")
matches = pattern.findall(text)
count = len(matches)
print(f"The pattern 'o.' appears {count} times.")
五、优化和性能考虑
在处理大文本或需要高性能的应用中,选择合适的方法和优化代码是非常重要的。
使用内置方法
内置方法如 count()
通常是最快的,因为它们是用 C 语言实现的并进行了优化。
避免不必要的遍历
尽可能避免在循环中重复计算或查找。例如,可以提前计算索引或使用缓存。
text = "Hello, World! Hello, Python!"
char = "o"
count = text.count(char)
print(f"The character '{char}' appears {count} times.")
六、实践案例
以下是一些实际案例,展示了如何在不同场景中使用上述方法。
案例一:查找文件中的特定字符
file_path = "example.txt"
char = "e"
count = 0
with open(file_path, "r") as file:
for line in file:
count += line.count(char)
print(f"The character '{char}' appears {count} times in the file.")
案例二:统计网页内容中的特定字符
import requests
url = "https://www.example.com"
char = "a"
response = requests.get(url)
content = response.text
count = content.count(char)
print(f"The character '{char}' appears {count} times in the webpage.")
通过以上方法,我们可以灵活地查找和计数字符串中的指定字符,根据不同的需求和场景选择合适的方法,以获得最佳性能和结果。
相关问答FAQs:
如何在Python中查找字符串中的特定字符?
在Python中,您可以使用字符串的find()
方法或index()
方法来查找特定字符的位置。这两个方法都可以返回字符在字符串中的索引。find()
方法在未找到字符时返回-1,而index()
方法会引发ValueError
异常。示例代码如下:
text = "Hello, world!"
position = text.find('o')
print(position) # 输出: 4
用Python如何统计字符串中某个字符的出现次数?
要统计某个字符在字符串中出现的次数,可以使用字符串的count()
方法。该方法会返回指定字符在字符串中出现的次数,非常简单易用。例如:
text = "Hello, world!"
count = text.count('o')
print(count) # 输出: 2
在处理大文本时,如何提高查找和计数字符的效率?
在处理大文本时,可以考虑使用正则表达式或字典来优化查找和计数的效率。使用re
模块中的findall()
方法可以快速找到所有匹配的字符,并通过返回的列表长度来获取计数。此外,您还可以遍历文本并使用字典记录每个字符的出现次数。这样可以在一次遍历中完成查找和计数操作。例如:
import re
text = "Hello, world!"
matches = re.findall('o', text)
print(len(matches)) # 输出: 2
或者使用字典:
text = "Hello, world!"
char_count = {}
for char in text:
char_count[char] = char_count.get(char, 0) + 1
print(char_count.get('o', 0)) # 输出: 2
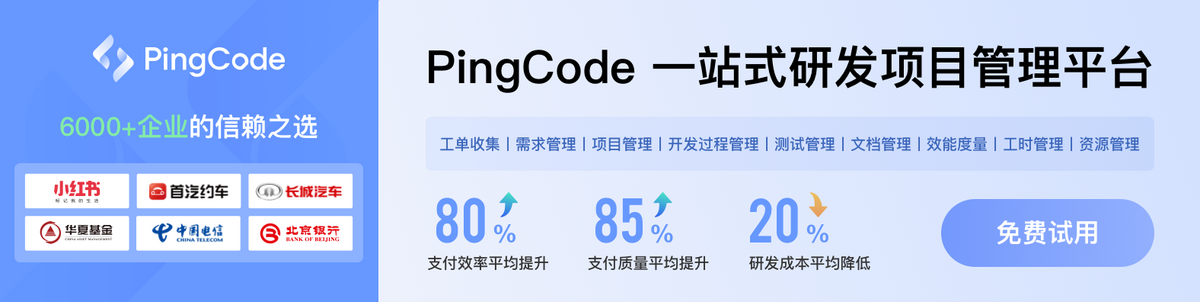