使用Python合并五个数字的步骤包括:使用算术操作、使用字符串拼接、使用列表和列表推导式、使用内置函数、使用第三方库。 其中,使用字符串拼接方法是比较简单且常用的方法。
例如,假设我们有五个数字:1, 2, 3, 4, 5。我们可以通过将这些数字转化为字符串并连接在一起的方法来合并它们。具体步骤如下:
- 将数字转化为字符串。
- 使用字符串拼接操作将它们连接在一起。
- 最后得到合并后的结果。
下面我们详细解释每种方法。
一、使用算术操作
这种方法适用于特定情况下的数字合并,例如将五个数字组合成一个数(比如12345)。
# 使用算术操作合并数字
def combine_numbers_with_arithmetic(numbers):
result = 0
for number in numbers:
result = result * 10 + number
return result
numbers = [1, 2, 3, 4, 5]
combined_number = combine_numbers_with_arithmetic(numbers)
print(combined_number) # 输出: 12345
在这里,我们通过将之前的结果乘以10来“腾出”一个位置,然后加上新的数字,从而实现数字的合并。
二、使用字符串拼接
字符串拼接是最直接的方法,适用于需要将数字合并成字符串的情况。
# 使用字符串拼接合并数字
def combine_numbers_with_string(numbers):
combined_string = ''.join(map(str, numbers))
return combined_string
numbers = [1, 2, 3, 4, 5]
combined_string = combine_numbers_with_string(numbers)
print(combined_string) # 输出: "12345"
这里,我们使用 map
函数将每个数字转换为字符串,然后使用 join
方法将它们连接在一起。
三、使用列表和列表推导式
列表推导式是一种简洁的方法,可以在一行代码中完成数字的合并。
# 使用列表推导式合并数字
def combine_numbers_with_list_comprehension(numbers):
return ''.join([str(number) for number in numbers])
numbers = [1, 2, 3, 4, 5]
combined_string = combine_numbers_with_list_comprehension(numbers)
print(combined_string) # 输出: "12345"
列表推导式提供了一种更清晰、更加Pythonic的方式来处理数字的合并。
四、使用内置函数
Python提供了一些强大的内置函数,可以帮助我们简化代码。
# 使用内置函数合并数字
def combine_numbers_with_builtin_functions(numbers):
return ''.join(map(str, numbers))
numbers = [1, 2, 3, 4, 5]
combined_string = combine_numbers_with_builtin_functions(numbers)
print(combined_string) # 输出: "12345"
这种方法与字符串拼接类似,但更加简洁。
五、使用第三方库
对于复杂的合并需求,可能需要使用第三方库。这里以NumPy为例。
import numpy as np
使用NumPy合并数字
def combine_numbers_with_numpy(numbers):
return ''.join(np.char.mod('%d', numbers))
numbers = np.array([1, 2, 3, 4, 5])
combined_string = combine_numbers_with_numpy(numbers)
print(combined_string) # 输出: "12345"
NumPy库提供了强大的数组处理功能,使得合并操作更加高效。
结论
通过上述几种方法,我们可以轻松地用Python合并五个数字。无论是通过算术操作、字符串拼接、列表推导式、内置函数还是第三方库,都能有效地实现这一操作。根据具体需求选择合适的方法,可以提高代码的可读性和执行效率。
相关问答FAQs:
如何在Python中合并多个数字为一个字符串?
在Python中,可以使用str()
函数将数字转换为字符串,然后使用join()
方法合并它们。例如,可以将五个数字放在一个列表中,使用join()
将它们合并为一个字符串。示例代码如下:
numbers = [1, 2, 3, 4, 5]
result = ''.join(str(num) for num in numbers)
print(result) # 输出: 12345
是否可以使用Python中的其他方法合并数字?
除了join()
方法外,Python还支持使用字符串格式化和f-string来合并数字。例如,可以使用f-string
如下实现:
a, b, c, d, e = 1, 2, 3, 4, 5
result = f"{a}{b}{c}{d}{e}"
print(result) # 输出: 12345
合并数字时如何处理不同的数据类型?
在合并不同数据类型的数字时,确保所有元素都是字符串类型。如果存在非数字类型,可以使用str()
函数进行转换。例如:
numbers = [1, '2', 3.0, 4, 'five']
result = ''.join(str(num) for num in numbers if isinstance(num, (int, float, str)))
print(result) # 输出: 1234five
这种方式能够有效处理不同类型的数据并合并成一个字符串。
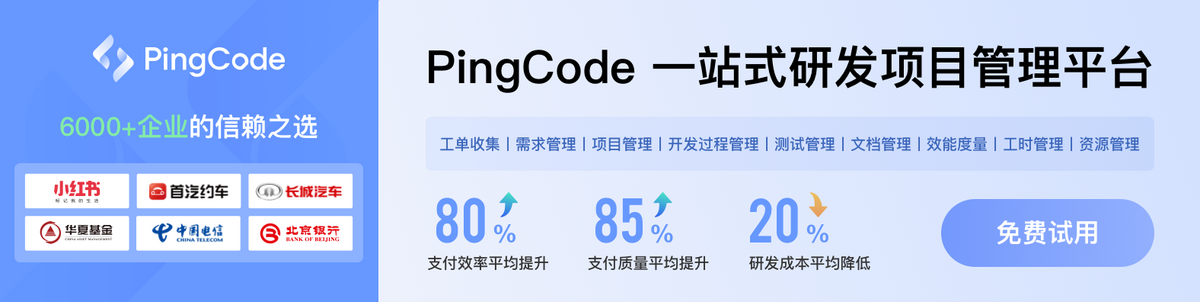