在Python中,绘制折线图的主要方法有:使用Matplotlib库、使用Pandas库、使用Seaborn库。这些方法各有优劣,可以根据具体需求进行选择。以下将详细介绍如何使用Matplotlib库绘制折线图。
一、使用Matplotlib库
Matplotlib是一个广泛使用的绘图库,功能强大且灵活,适用于各种类型的图表绘制。以下是使用Matplotlib库绘制折线图的详细步骤。
1、安装Matplotlib库
在开始使用Matplotlib绘制折线图之前,需要确保已安装该库。可以使用pip命令安装:
pip install matplotlib
2、导入必要的库
在绘制折线图之前,需要导入Matplotlib库及其他可能需要的库,如NumPy等:
import matplotlib.pyplot as plt
import numpy as np
3、准备数据
在绘制折线图之前,需要准备好数据。数据可以是从文件读取、数据库查询、API获取或手动定义的列表或数组。以下是一个简单的示例数据:
x = np.arange(0, 10, 1)
y = np.sin(x)
4、创建折线图
使用Matplotlib库的plot
函数可以轻松绘制折线图:
plt.plot(x, y)
plt.title('Simple Line Plot')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
plt.show()
5、添加更多元素
Matplotlib提供了丰富的功能,可以添加多条折线、注释、图例等。以下是一个添加多条折线和图例的示例:
y2 = np.cos(x)
plt.plot(x, y, label='sin(x)')
plt.plot(x, y2, label='cos(x)')
plt.title('Multiple Line Plot')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
plt.legend()
plt.show()
二、使用Pandas库
Pandas库不仅是一个强大的数据分析工具,还集成了Matplotlib的绘图功能,可以方便地绘制折线图。
1、安装Pandas库
确保已安装Pandas库:
pip install pandas
2、导入必要的库
导入Pandas库及其他必要的库:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
3、准备数据
Pandas库通常使用DataFrame来存储数据。以下是一个简单的示例数据:
data = {'x': np.arange(0, 10, 1), 'y': np.sin(np.arange(0, 10, 1))}
df = pd.DataFrame(data)
4、创建折线图
使用Pandas库的plot
函数可以轻松绘制折线图:
df.plot(x='x', y='y', title='Pandas Line Plot')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
plt.show()
三、使用Seaborn库
Seaborn是基于Matplotlib的高级绘图库,提供了更美观和易用的绘图接口。
1、安装Seaborn库
确保已安装Seaborn库:
pip install seaborn
2、导入必要的库
导入Seaborn库及其他必要的库:
import seaborn as sns
import numpy as np
import matplotlib.pyplot as plt
3、准备数据
Seaborn库通常使用Pandas DataFrame来存储数据。以下是一个简单的示例数据:
data = {'x': np.arange(0, 10, 1), 'y': np.sin(np.arange(0, 10, 1))}
df = pd.DataFrame(data)
4、创建折线图
使用Seaborn库的lineplot
函数可以轻松绘制折线图:
sns.lineplot(x='x', y='y', data=df)
plt.title('Seaborn Line Plot')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
plt.show()
5、添加更多元素
Seaborn库还提供了丰富的功能,可以添加更多元素,如多条折线、图例等。以下是一个添加多条折线和图例的示例:
data = {'x': np.tile(np.arange(0, 10, 1), 2),
'y': np.concatenate([np.sin(np.arange(0, 10, 1)), np.cos(np.arange(0, 10, 1))]),
'line': ['sin']*10 + ['cos']*10}
df = pd.DataFrame(data)
sns.lineplot(x='x', y='y', hue='line', data=df)
plt.title('Seaborn Multiple Line Plot')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
plt.show()
6、总结
在Python中绘制折线图的方法有很多,其中最常用的是Matplotlib、Pandas和Seaborn库。Matplotlib功能强大且灵活,适用于各种类型的图表绘制;Pandas集成了Matplotlib的绘图功能,适合数据分析和绘图结合的场景;Seaborn基于Matplotlib,提供了更美观和易用的绘图接口。根据具体需求选择合适的库,可以更高效地完成折线图的绘制工作。
相关问答FAQs:
在Python中绘制折线图需要哪些基本的库和工具?
在Python中,绘制折线图通常使用Matplotlib库,这是一个强大的绘图库。用户还可以使用NumPy来处理数据,Pandas用于数据分析和处理。确保在开始之前安装这些库,可以使用pip install matplotlib numpy pandas
命令。
如何使用Matplotlib绘制简单的折线图?
创建一个简单的折线图非常容易。首先,导入Matplotlib,并准备好要绘制的数据。接着,使用plt.plot()
函数来绘制数据。最后,调用plt.show()
来展示图形。例如:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y)
plt.title("简单折线图")
plt.xlabel("X轴")
plt.ylabel("Y轴")
plt.show()
如何自定义折线图的样式和颜色?
用户可以通过传递参数自定义折线图的样式和颜色。在plt.plot()
函数中,可以添加参数如color
、linestyle
和marker
来改变线条颜色、样式以及数据点的标记。例如:
plt.plot(x, y, color='blue', linestyle='--', marker='o')
通过这些参数,用户可以创建更加个性化和美观的折线图,使其更符合需求。
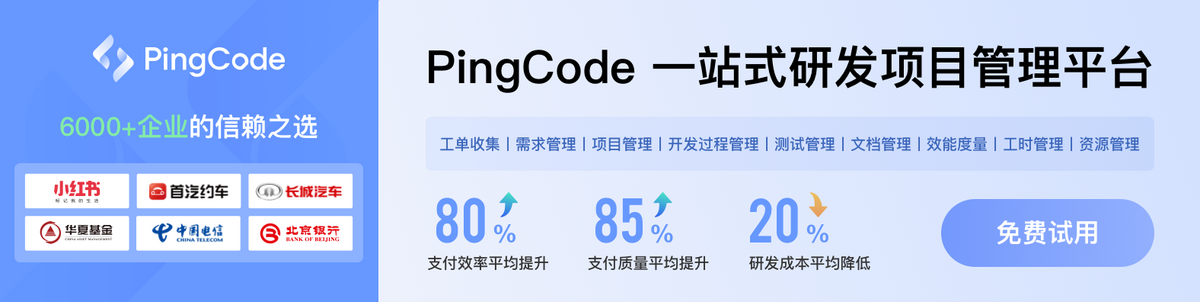