Python编程如何做出选择题
Python编程做出选择题可以通过使用控制流、列表、字典、函数等多种方式来实现、可以选择使用简单的命令行交互,也可以使用图形用户界面(GUI)库如Tkinter来创建更复杂的选择题界面。下面将详细描述如何使用这几种方法来实现选择题。
一、使用基本的控制流和列表
使用Python的控制流语句以及列表,可以很容易地实现一个简单的选择题系统。这种方法适用于命令行界面的选择题。
1、定义选择题
首先,我们需要定义选择题的内容,包括问题、选项以及正确答案。可以使用字典或列表来存储这些信息:
questions = [
{
"question": "What is the capital of France?",
"options": ["A. Berlin", "B. Madrid", "C. Paris", "D. Rome"],
"answer": "C"
},
{
"question": "Which planet is known as the Red Planet?",
"options": ["A. Earth", "B. Mars", "C. Jupiter", "D. Saturn"],
"answer": "B"
}
]
2、显示问题并获取用户输入
接下来,我们需要循环遍历每个问题,显示给用户并获取用户的选择:
def ask_question(question):
print(question["question"])
for option in question["options"]:
print(option)
answer = input("Please enter your answer (A/B/C/D): ").strip().upper()
return answer
def check_answer(question, answer):
return question["answer"] == answer
score = 0
for question in questions:
user_answer = ask_question(question)
if check_answer(question, user_answer):
print("Correct!")
score += 1
else:
print(f"Wrong! The correct answer is {question['answer']}")
print(f"Your final score is {score}/{len(questions)}")
二、使用函数和模块化设计
为了使代码更具可读性和可维护性,可以将代码分成多个函数,每个函数负责一个特定的任务。例如:
1、分离输入和输出逻辑
通过将输入和输出逻辑分离,可以更容易地进行单元测试和修改:
def get_user_input(prompt):
return input(prompt).strip().upper()
def display_question(question):
print(question["question"])
for option in question["options"]:
print(option)
def display_result(score, total):
print(f"Your final score is {score}/{total}")
2、主流程控制
将主流程控制逻辑放在一个函数中,使得代码结构更加清晰:
def main():
score = 0
for question in questions:
display_question(question)
user_answer = get_user_input("Please enter your answer (A/B/C/D): ")
if check_answer(question, user_answer):
print("Correct!")
score += 1
else:
print(f"Wrong! The correct answer is {question['answer']}")
display_result(score, len(questions))
if __name__ == "__main__":
main()
三、使用图形用户界面(GUI)
使用Tkinter等GUI库,可以创建一个图形化界面的选择题系统。这种方法适用于需要更友好用户体验的选择题应用。
1、安装Tkinter
Tkinter通常是Python标准库的一部分,因此不需要额外安装。如果没有,可以使用以下命令进行安装:
pip install tk
2、创建基本的GUI框架
首先,创建一个基本的Tkinter窗口:
import tkinter as tk
class QuizApp:
def __init__(self, root):
self.root = root
self.root.title("Quiz Application")
self.current_question = 0
self.score = 0
self.question_label = tk.Label(root, text="", wraplength=400)
self.question_label.pack()
self.options = []
for i in range(4):
btn = tk.Button(root, text="", command=lambda i=i: self.check_answer(i))
btn.pack(fill='x')
self.options.append(btn)
self.load_question()
def load_question(self):
question = questions[self.current_question]
self.question_label.config(text=question["question"])
for i, option in enumerate(question["options"]):
self.options[i].config(text=option)
def check_answer(self, index):
question = questions[self.current_question]
if question["options"][index].startswith(question["answer"]):
self.score += 1
self.current_question += 1
if self.current_question < len(questions):
self.load_question()
else:
self.show_result()
def show_result(self):
for btn in self.options:
btn.pack_forget()
self.question_label.config(text=f"Your final score is {self.score}/{len(questions)}")
if __name__ == "__main__":
root = tk.Tk()
app = QuizApp(root)
root.mainloop()
四、添加更多功能
可以在现有的选择题系统中添加更多功能,例如计时器、题库管理、成绩保存等,以增强系统的实用性和用户体验。
1、添加计时器
可以通过使用threading
模块或者Tkinter的after
方法来实现计时功能:
import threading
class QuizApp:
def __init__(self, root):
self.root = root
self.root.title("Quiz Application")
self.current_question = 0
self.score = 0
self.time_left = 30 # seconds
self.question_label = tk.Label(root, text="", wraplength=400)
self.question_label.pack()
self.timer_label = tk.Label(root, text=f"Time left: {self.time_left}s")
self.timer_label.pack()
self.options = []
for i in range(4):
btn = tk.Button(root, text="", command=lambda i=i: self.check_answer(i))
btn.pack(fill='x')
self.options.append(btn)
self.load_question()
self.start_timer()
def load_question(self):
question = questions[self.current_question]
self.question_label.config(text=question["question"])
for i, option in enumerate(question["options"]):
self.options[i].config(text=option)
def check_answer(self, index):
question = questions[self.current_question]
if question["options"][index].startswith(question["answer"]):
self.score += 1
self.current_question += 1
if self.current_question < len(questions):
self.load_question()
else:
self.show_result()
def show_result(self):
for btn in self.options:
btn.pack_forget()
self.question_label.config(text=f"Your final score is {self.score}/{len(questions)}")
self.timer_label.pack_forget()
def start_timer(self):
def countdown():
while self.time_left > 0:
self.time_left -= 1
self.timer_label.config(text=f"Time left: {self.time_left}s")
time.sleep(1)
self.show_result()
timer_thread = threading.Thread(target=countdown)
timer_thread.start()
if __name__ == "__main__":
root = tk.Tk()
app = QuizApp(root)
root.mainloop()
2、题库管理
可以将选择题存储在外部文件中(如JSON格式),并在程序启动时加载:
import json
def load_questions(file_path):
with open(file_path, 'r') as file:
return json.load(file)
questions = load_questions('questions.json')
五、总结
使用Python编程实现选择题可以有多种方式,从简单的命令行交互到复杂的图形用户界面,再到添加计时器和题库管理等功能。通过使用函数和模块化设计,可以使代码更加清晰和易于维护。无论是初学者还是有经验的程序员,都可以根据自己的需求选择合适的方法来实现选择题系统。
相关问答FAQs:
如何在Python中创建选择题的基本结构?
在Python中,可以使用列表或字典来存储选择题的选项和答案。例如,您可以使用字典来存储问题和其对应的选项,以及正确答案。通过循环遍历这些问题,可以让用户逐个回答,从而实现选择题的基本功能。
如何处理用户的选择并给出反馈?
可以通过输入函数获取用户的选择,并与正确答案进行比较。利用条件语句,您可以提供用户反馈,告知他们选择是否正确。此外,您还可以记录用户的得分,通过简单的计数器来统计正确答案的数量。
如何设计更复杂的选择题,例如多选题?
对于多选题,您可以使用列表来存储用户的多个选择。在获取用户输入时,可以要求他们输入多个选项的编号。通过将用户的输入与正确答案进行比较,可以判断哪些选项是正确的。您还可以设计逻辑来处理部分正确的情况,以提高题目的灵活性和趣味性。
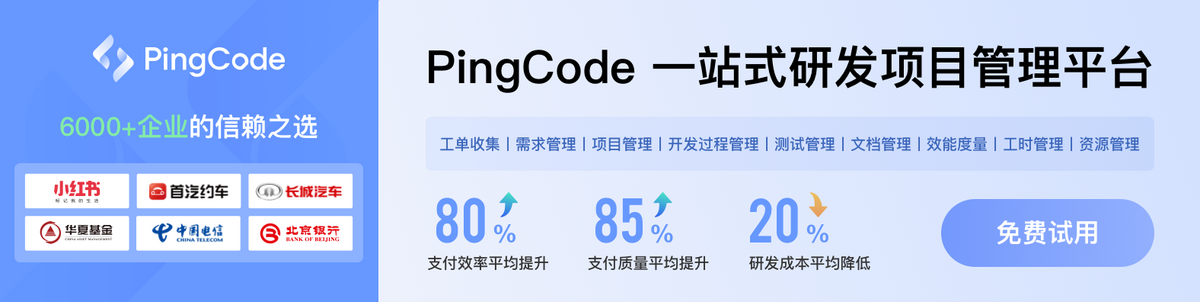