查看Python中是否有某个函数的方法有很多:使用hasattr()
函数、使用dir()
函数、查看模块的源代码、使用inspect
模块。 在这里,我们将详细描述其中的一种方法,即使用hasattr()
函数来检查对象或模块中是否存在某个函数。
一、使用 hasattr()
函数
hasattr()
是 Python 内置函数之一,它用于检查某个对象是否具有指定的属性或方法。它的使用方法非常简单,只需要传入两个参数:对象和属性名(以字符串形式)。
示例代码
import math
检查 math 模块中是否存在 sin 函数
result = hasattr(math, 'sin')
print(f"Does math module have 'sin' function? {result}")
检查 math 模块中是否存在 non_existing_function 函数
result = hasattr(math, 'non_existing_function')
print(f"Does math module have 'non_existing_function'? {result}")
在上面的示例代码中,我们导入了 math
模块,并使用 hasattr()
函数来检查 math
模块中是否存在 sin
函数和 non_existing_function
函数。运行结果会显示 sin
函数存在,而 non_existing_function
函数不存在。
二、使用 dir()
函数
dir()
函数返回指定对象的所有属性和方法的列表。通过检查返回的列表,可以确定某个函数是否存在于对象或模块中。
示例代码
import math
获取 math 模块的所有属性和方法
attributes = dir(math)
检查 'sin' 函数是否在属性列表中
if 'sin' in attributes:
print("math module has 'sin' function")
else:
print("math module does not have 'sin' function")
检查 'non_existing_function' 函数是否在属性列表中
if 'non_existing_function' in attributes:
print("math module has 'non_existing_function' function")
else:
print("math module does not have 'non_existing_function' function")
在上面的示例代码中,我们使用 dir()
函数获取 math
模块的所有属性和方法的列表,并检查 sin
函数和 non_existing_function
函数是否在该列表中。
三、查看模块的源代码
有时候,直接查看模块的源代码是最直观的方法。大多数模块都有详细的文档和注释,阅读这些文档和注释可以帮助我们了解模块的功能和特性。
示例
以 math
模块为例,可以通过以下方式查看其源代码:
- 打开 Python 解释器。
- 导入
math
模块。 - 使用
help()
函数查看模块的文档。
import math
help(math)
四、使用 inspect
模块
Python 的 inspect
模块提供了多种检查对象和模块的工具,可以用来获取详细的函数信息。
示例代码
import inspect
import math
检查 math 模块中是否存在 sin 函数
is_function = inspect.isfunction(math.sin)
print(f"Is 'sin' a function in math module? {is_function}")
尝试检查 non_existing_function 函数(会抛出 AttributeError)
try:
is_function = inspect.isfunction(math.non_existing_function)
print(f"Is 'non_existing_function' a function in math module? {is_function}")
except AttributeError:
print("math module does not have 'non_existing_function' function")
在上面的示例代码中,我们使用 inspect.isfunction()
函数来检查 math
模块中的 sin
函数是否为函数类型。对于不存在的函数 non_existing_function
,会抛出 AttributeError
异常。
五、使用 getattr()
函数
getattr()
是另一个 Python 内置函数,它用于获取对象的属性。如果指定的属性不存在,可以返回一个默认值,避免抛出异常。
示例代码
import math
获取 math 模块中的 sin 函数,如果不存在则返回 None
sin_function = getattr(math, 'sin', None)
if sin_function:
print("math module has 'sin' function")
else:
print("math module does not have 'sin' function")
获取 math 模块中的 non_existing_function 函数,如果不存在则返回 None
non_existing_function = getattr(math, 'non_existing_function', None)
if non_existing_function:
print("math module has 'non_existing_function' function")
else:
print("math module does not have 'non_existing_function' function")
在上面的示例代码中,我们使用 getattr()
函数获取 math
模块中的 sin
函数和 non_existing_function
函数。如果指定的函数不存在,则返回 None
。
六、使用 try-except
语句
在某些情况下,可以使用 try-except
语句来捕获异常,检查函数是否存在。
示例代码
import math
尝试调用 math 模块中的 sin 函数
try:
sin_function = math.sin
print("math module has 'sin' function")
except AttributeError:
print("math module does not have 'sin' function")
尝试调用 math 模块中的 non_existing_function 函数
try:
non_existing_function = math.non_existing_function
print("math module has 'non_existing_function' function")
except AttributeError:
print("math module does not have 'non_existing_function' function")
在上面的示例代码中,我们使用 try-except
语句尝试访问 math
模块中的 sin
函数和 non_existing_function
函数。如果指定的函数不存在,则捕获 AttributeError
异常。
七、使用第三方工具
有一些第三方工具和库可以帮助我们检查模块和对象的属性和方法,例如 pydoc
和 IPython
。
示例代码
import pydoc
使用 pydoc 查看 math 模块的文档
pydoc.doc(math)
在上面的示例代码中,我们使用 pydoc
模块查看 math
模块的文档,了解其属性和方法。
总结
检查Python中是否存在某个函数的方法有很多,常见的方法包括:使用hasattr()
函数、使用dir()
函数、查看模块的源代码、使用inspect
模块、使用getattr()
函数、使用try-except
语句、使用第三方工具。 通过这些方法,可以轻松检查模块和对象中的函数和属性,帮助我们更好地理解和使用Python模块。
相关问答FAQs:
如何在Python中检查某个函数是否存在?
要检查Python中是否存在某个函数,可以使用内置的globals()
函数或locals()
函数来查看当前作用域中的所有函数和变量。通过这种方式,您可以判断特定函数名是否被定义。例如,您可以使用'function_name' in globals()
来检查全局命名空间中是否存在该函数。
在模块中查找特定函数的最佳方法是什么?
如果您想在特定模块中查找函数,可以使用dir()
函数获取模块中的所有属性和方法。结合getattr()
函数,您可以判断特定函数是否在模块中。例如,使用import module_name
导入模块后,'function_name' in dir(module_name)
可以帮助您判断该函数是否存在。
如何处理未定义函数的情况?
当尝试调用一个未定义的函数时,Python会抛出NameError
。为了避免程序中断,您可以使用try-except
结构来捕获此错误。这样,您可以在函数不存在时提供默认值或采取其他措施,确保程序的平稳运行。
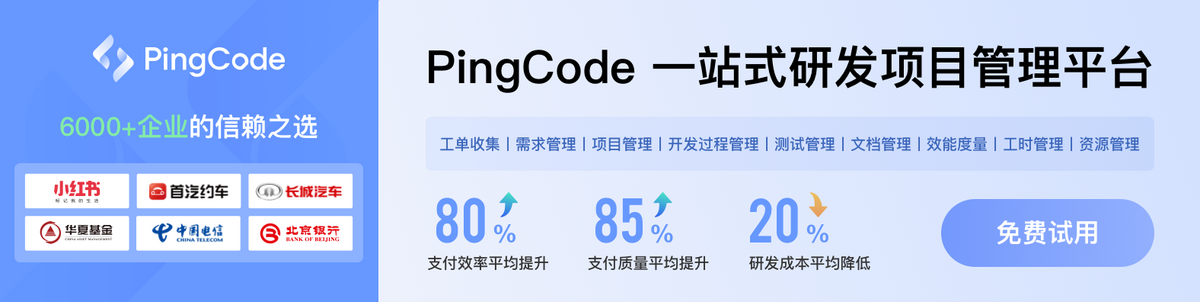