在Python中,可以使用多种方法来检测文件夹是否存在,主要的方法包括os模块和pathlib模块。其中,os.path.exists()、os.path.isdir()、pathlib.Path.exists()都可以用来检测文件夹是否存在。推荐使用pathlib模块,因为它提供了更面向对象的接口、更易读的代码。
下面将详细介绍这几种方法并给出示例代码。
一、os模块
os模块是Python标准库中的一部分,提供了许多与操作系统交互的功能。通过os模块,我们可以轻松地检测文件夹是否存在。
1.1 使用os.path.exists()
os.path.exists()函数用于判断指定路径是否存在。它可以用于检测文件和文件夹的存在。
import os
def check_directory_exists(path):
if os.path.exists(path):
print(f"The directory '{path}' exists.")
else:
print(f"The directory '{path}' does not exist.")
示例
check_directory_exists('/path/to/directory')
1.2 使用os.path.isdir()
os.path.isdir()函数用于判断指定路径是否是一个目录。相比os.path.exists(),它更适合专门用于检测目录的存在。
import os
def check_directory_exists(path):
if os.path.isdir(path):
print(f"The directory '{path}' exists.")
else:
print(f"The directory '{path}' does not exist.")
示例
check_directory_exists('/path/to/directory')
二、pathlib模块
pathlib模块是Python 3.4引入的,用于处理文件系统路径。它提供了面向对象的API,使得代码更易读、更简洁。
2.1 使用pathlib.Path.exists()
pathlib.Path.exists()函数用于判断路径是否存在。它可以用于检测文件和目录的存在。
from pathlib import Path
def check_directory_exists(path):
directory = Path(path)
if directory.exists():
print(f"The directory '{path}' exists.")
else:
print(f"The directory '{path}' does not exist.")
示例
check_directory_exists('/path/to/directory')
2.2 使用pathlib.Path.is_dir()
pathlib.Path.is_dir()函数用于判断路径是否是一个目录。相比pathlib.Path.exists(),它更适合专门用于检测目录的存在。
from pathlib import Path
def check_directory_exists(path):
directory = Path(path)
if directory.is_dir():
print(f"The directory '{path}' exists.")
else:
print(f"The directory '{path}' does not exist.")
示例
check_directory_exists('/path/to/directory')
三、详细解释pathlib模块的优势
pathlib模块提供了面向对象的接口,代码更易读、更简洁。pathlib.Path类封装了许多文件系统操作,使得代码更易于理解和维护。
3.1 更易读的代码
使用pathlib模块,代码更加直观。例如,以下代码使用pathlib模块和os模块分别创建文件夹:
# 使用os模块
import os
def create_directory(path):
if not os.path.exists(path):
os.makedirs(path)
使用pathlib模块
from pathlib import Path
def create_directory(path):
directory = Path(path)
if not directory.exists():
directory.mkdir(parents=True)
可以看到,pathlib模块的代码更加简洁、易读。
3.2 更强的功能
pathlib模块提供了更多的功能,例如可以方便地进行路径拼接、文件操作等。例如:
from pathlib import Path
路径拼接
base_path = Path('/path/to')
full_path = base_path / 'directory'
创建文件夹
full_path.mkdir(parents=True, exist_ok=True)
创建文件
file_path = full_path / 'file.txt'
file_path.touch()
四、实践示例
4.1 检测文件夹是否存在并创建
综合以上内容,我们可以编写一个函数,检测文件夹是否存在,如果不存在则创建它:
from pathlib import Path
def ensure_directory_exists(path):
directory = Path(path)
if not directory.exists():
directory.mkdir(parents=True)
print(f"The directory '{path}' has been created.")
else:
print(f"The directory '{path}' already exists.")
示例
ensure_directory_exists('/path/to/directory')
4.2 结合文件操作
我们可以进一步扩展这个函数,结合文件操作,例如在确保文件夹存在后创建一个文件:
from pathlib import Path
def ensure_directory_and_file_exists(directory_path, file_name):
directory = Path(directory_path)
if not directory.exists():
directory.mkdir(parents=True)
print(f"The directory '{directory_path}' has been created.")
else:
print(f"The directory '{directory_path}' already exists.")
file_path = directory / file_name
if not file_path.exists():
file_path.touch()
print(f"The file '{file_name}' has been created in '{directory_path}'.")
else:
print(f"The file '{file_name}' already exists in '{directory_path}'.")
示例
ensure_directory_and_file_exists('/path/to/directory', 'file.txt')
4.3 处理文件夹和文件的异常情况
在实际应用中,我们还需要考虑异常情况,例如路径不存在、没有权限等。我们可以使用try-except块来处理这些异常:
from pathlib import Path
def ensure_directory_and_file_exists(directory_path, file_name):
try:
directory = Path(directory_path)
if not directory.exists():
directory.mkdir(parents=True)
print(f"The directory '{directory_path}' has been created.")
else:
print(f"The directory '{directory_path}' already exists.")
file_path = directory / file_name
if not file_path.exists():
file_path.touch()
print(f"The file '{file_name}' has been created in '{directory_path}'.")
else:
print(f"The file '{file_name}' already exists in '{directory_path}'.")
except Exception as e:
print(f"An error occurred: {e}")
示例
ensure_directory_and_file_exists('/path/to/directory', 'file.txt')
通过使用try-except块,我们可以捕获并处理可能发生的异常,确保程序的健壮性。
五、总结
在Python中,检测文件夹是否存在可以使用os模块和pathlib模块。推荐使用pathlib模块,因为它提供了更面向对象的接口、更易读的代码。通过pathlib.Path.exists()和pathlib.Path.is_dir()函数,我们可以轻松地检测文件夹是否存在。此外,pathlib模块还提供了更多的功能,使得文件系统操作更加方便、直观。在实际应用中,我们还需要考虑异常情况,通过try-except块来处理可能发生的错误,确保程序的健壮性。希望这篇文章能帮助你更好地理解和使用Python进行文件夹检测和操作。
相关问答FAQs:
如何在Python中检查文件夹的存在性?
在Python中,可以使用os
模块中的path.exists()
或path.isdir()
函数来检测一个文件夹是否存在。以下是一个简单的示例代码:
import os
folder_path = 'your_folder_path_here'
if os.path.exists(folder_path) and os.path.isdir(folder_path):
print("文件夹存在。")
else:
print("文件夹不存在。")
使用Python检查文件夹是否存在的最佳实践是什么?
在检查文件夹存在性时,建议使用try-except
语句来处理潜在的异常情况,例如路径错误或权限问题。这种方法使得代码更加健壮,能够处理各种不确定性。
import os
folder_path = 'your_folder_path_here'
try:
if os.path.exists(folder_path) and os.path.isdir(folder_path):
print("文件夹存在。")
else:
print("文件夹不存在。")
except Exception as e:
print(f"发生错误:{e}")
在Python中,如何创建一个不存在的文件夹?
如果检测到文件夹不存在,可以使用os.makedirs()
函数来创建它。此函数不仅可以创建单个文件夹,还可以创建多层嵌套的文件夹。示例如下:
import os
folder_path = 'your_folder_path_here'
if not os.path.exists(folder_path):
os.makedirs(folder_path)
print("文件夹创建成功。")
else:
print("文件夹已存在。")
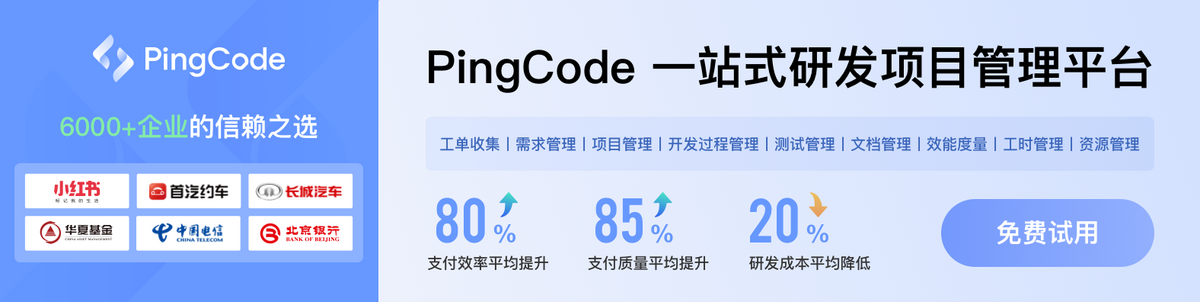