Python下载网络图片的大小
使用requests库、使用PIL库、使用urllib库、使用aiohttp库、使用wget库,这些方法都可以在Python中实现下载网络图片并获取其大小。接下来,我将详细描述其中一种方法的实现过程,以帮助你更好地理解和应用这些技术。
在此示例中,我们将使用requests
库来下载网络图片,并使用PIL
库(Pillow)来获取图片的大小。
import requests
from PIL import Image
from io import BytesIO
def download_image(url):
response = requests.get(url)
if response.status_code == 200:
img = Image.open(BytesIO(response.content))
return img
else:
raise Exception("Failed to download image")
def get_image_size(img):
return img.size
url = "https://example.com/image.jpg"
img = download_image(url)
width, height = get_image_size(img)
print(f"Image size: {width}x{height}")
通过以上代码,我们可以看到如何使用requests
库下载网络图片,并使用PIL
库获取图片的大小。接下来,我们将详细介绍其他几种方法。
一、使用requests库
requests
库是Python中最流行的HTTP库之一。它使得HTTP请求变得非常简单和优雅。我们可以使用requests.get
方法下载网络图片,并将其保存到本地文件系统。
import requests
def download_image(url, save_path):
response = requests.get(url, stream=True)
if response.status_code == 200:
with open(save_path, 'wb') as file:
for chunk in response.iter_content(1024):
file.write(chunk)
else:
raise Exception("Failed to download image")
url = "https://example.com/image.jpg"
save_path = "image.jpg"
download_image(url, save_path)
通过以上代码,我们成功地使用requests
库下载了网络图片并将其保存到本地文件系统。接下来,我们将介绍如何获取图片的大小。
from PIL import Image
def get_image_size(image_path):
img = Image.open(image_path)
return img.size
image_path = "image.jpg"
width, height = get_image_size(image_path)
print(f"Image size: {width}x{height}")
通过以上代码,我们成功地使用PIL
库获取了图片的大小。
二、使用PIL库
PIL
库(Pillow)是Python中的一个图像处理库。它支持打开、操作和保存多种格式的图像。我们可以使用PIL
库打开网络图片,并获取其大小。
from PIL import Image
import requests
from io import BytesIO
def download_image(url):
response = requests.get(url)
if response.status_code == 200:
img = Image.open(BytesIO(response.content))
return img
else:
raise Exception("Failed to download image")
def get_image_size(img):
return img.size
url = "https://example.com/image.jpg"
img = download_image(url)
width, height = get_image_size(img)
print(f"Image size: {width}x{height}")
通过以上代码,我们成功地使用PIL
库下载网络图片并获取其大小。
三、使用urllib库
urllib
库是Python标准库中的一个模块。它提供了一系列用于操作URL的功能。我们可以使用urllib.request.urlretrieve
方法下载网络图片,并将其保存到本地文件系统。
import urllib.request
def download_image(url, save_path):
urllib.request.urlretrieve(url, save_path)
url = "https://example.com/image.jpg"
save_path = "image.jpg"
download_image(url, save_path)
通过以上代码,我们成功地使用urllib
库下载了网络图片并将其保存到本地文件系统。接下来,我们将介绍如何获取图片的大小。
from PIL import Image
def get_image_size(image_path):
img = Image.open(image_path)
return img.size
image_path = "image.jpg"
width, height = get_image_size(image_path)
print(f"Image size: {width}x{height}")
通过以上代码,我们成功地使用PIL
库获取了图片的大小。
四、使用aiohttp库
aiohttp
库是一个异步HTTP客户端/服务器框架。它允许我们在异步编程中使用HTTP请求。我们可以使用aiohttp
库下载网络图片,并将其保存到本地文件系统。
import aiohttp
import asyncio
async def download_image(url, save_path):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
if response.status == 200:
with open(save_path, 'wb') as file:
file.write(await response.read())
else:
raise Exception("Failed to download image")
url = "https://example.com/image.jpg"
save_path = "image.jpg"
asyncio.run(download_image(url, save_path))
通过以上代码,我们成功地使用aiohttp
库下载了网络图片并将其保存到本地文件系统。接下来,我们将介绍如何获取图片的大小。
from PIL import Image
def get_image_size(image_path):
img = Image.open(image_path)
return img.size
image_path = "image.jpg"
width, height = get_image_size(image_path)
print(f"Image size: {width}x{height}")
通过以上代码,我们成功地使用PIL
库获取了图片的大小。
五、使用wget库
wget
库是一个命令行工具,它可以从网络上下载文件。我们可以使用wget.download
方法下载网络图片,并将其保存到本地文件系统。
import wget
def download_image(url, save_path):
wget.download(url, save_path)
url = "https://example.com/image.jpg"
save_path = "image.jpg"
download_image(url, save_path)
通过以上代码,我们成功地使用wget
库下载了网络图片并将其保存到本地文件系统。接下来,我们将介绍如何获取图片的大小。
from PIL import Image
def get_image_size(image_path):
img = Image.open(image_path)
return img.size
image_path = "image.jpg"
width, height = get_image_size(image_path)
print(f"Image size: {width}x{height}")
通过以上代码,我们成功地使用PIL
库获取了图片的大小。
结论
通过以上几种方法,我们可以看到如何使用requests
库、PIL
库、urllib
库、aiohttp
库和wget
库下载网络图片并获取其大小。每种方法都有其优缺点,可以根据具体需求选择合适的方法。希望通过本文的介绍,能够帮助你更好地理解和应用这些技术。
相关问答FAQs:
如何使用Python获取网络图片的大小?
要获取网络图片的大小,可以使用Python的requests库和PIL(Pillow)库。首先,使用requests库下载图片,然后利用Pillow库的Image模块打开图片,从而获取其尺寸。以下是一个简单的示例代码:
import requests
from PIL import Image
from io import BytesIO
url = '图片的URL地址'
response = requests.get(url)
img = Image.open(BytesIO(response.content))
print(f'图片大小: {img.size}') # 输出格式为 (宽度, 高度)
下载网络图片时需要注意哪些事项?
在下载网络图片时,确保遵循版权法和网站的使用条款。在下载之前,最好检查图片的授权信息。此外,处理大文件时应关注网络带宽,避免给服务器带来不必要的负担。
如何处理下载失败的情况?
下载网络图片时,可能会遇到各种错误,如链接失效或网络问题。可以通过捕获异常来处理这些情况。例如,使用try-except语句捕获请求异常,并在下载失败时给予适当的提示或重试机制:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
except requests.exceptions.RequestException as e:
print(f'下载失败: {e}')
在Python中如何优化图片下载的速度?
为了提高下载速度,可以使用多线程或异步编程来并行下载多个图片。使用Python的concurrent.futures库或asyncio库可以有效地实现这一点,从而减少整体下载时间。例如,使用ThreadPoolExecutor来并行下载多个图片:
from concurrent.futures import ThreadPoolExecutor
urls = ['图片URL1', '图片URL2', '图片URL3']
def download_image(url):
response = requests.get(url)
return Image.open(BytesIO(response.content))
with ThreadPoolExecutor() as executor:
images = list(executor.map(download_image, urls))
通过以上方法,可以实现高效的网络图片下载及其大小获取,确保流畅的用户体验。
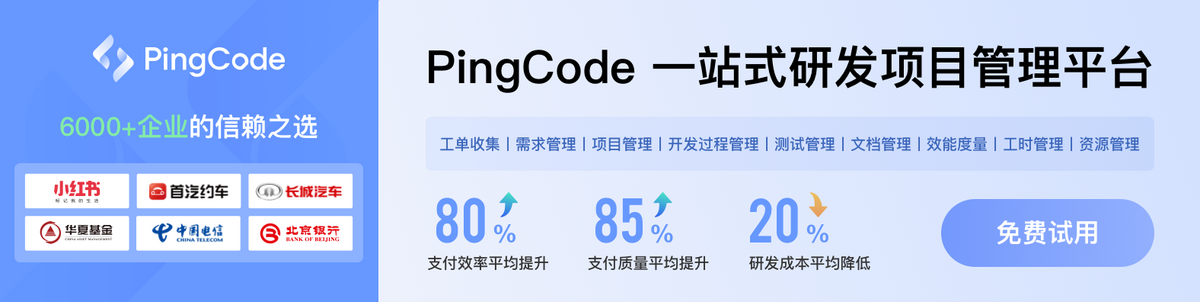