在Python中使用打印机打印代码的方法有多种:使用操作系统命令、使用第三方库如 win32print
、将代码保存为文件后打印。本文将详细介绍如何在Python中实现这些方法,并列举具体操作步骤。
一、使用操作系统命令打印代码
操作系统命令是最简单、直接的方法之一。我们可以通过Python的 subprocess
模块来调用操作系统的打印命令。
import subprocess
def print_file(file_path):
# 对于Windows系统,可以使用 "notepad /p" 命令
subprocess.run(['notepad', '/p', file_path])
示例用法
print_file('example.py')
在上述代码中,我们通过 subprocess.run
方法调用了 Windows 系统的 notepad
命令,并使用 /p
参数进行打印。此方法简单快捷,适用于打印单个文件。
二、使用第三方库 win32print
对于需要更多控制的情况,可以使用 win32print
库。它提供了更多的打印选项和功能。
安装 pywin32
库
首先需要安装 pywin32
库:
pip install pywin32
打印代码示例
import win32print
import win32ui
from PIL import Image, ImageWin
def print_file(file_path):
# 打开打印机
printer_name = win32print.GetDefaultPrinter()
hprinter = win32print.OpenPrinter(printer_name)
# 开始打印任务
job = win32print.StartDocPrinter(hprinter, 1, ("Python Printing", None, "RAW"))
win32print.StartPagePrinter(hprinter)
# 读取文件内容
with open(file_path, 'r') as file:
lines = file.readlines()
# 打印每一行
for line in lines:
win32print.WritePrinter(hprinter, line.encode('utf-8'))
# 结束打印任务
win32print.EndPagePrinter(hprinter)
win32print.EndDocPrinter(hprinter)
win32print.ClosePrinter(hprinter)
示例用法
print_file('example.py')
在上述代码中,我们使用 win32print
库打开默认打印机,开始打印任务,并逐行读取并打印文件内容。最后,我们结束打印任务并关闭打印机。
三、将代码保存为文件后打印
有时我们可能需要先将代码保存为文件,然后再打印。这种方法适用于需要先对代码进行处理或格式化的情况。
保存代码为文件
def save_code_to_file(code, file_path):
with open(file_path, 'w') as file:
file.write(code)
示例代码
example_code = """
def hello_world():
print("Hello, World!")
"""
保存为文件
save_code_to_file(example_code, 'example.py')
打印文件
保存文件后,可以使用上述方法之一进行打印。
四、设置打印格式
打印格式对于代码的可读性非常重要。我们可以通过调整字体、行间距等参数来优化打印效果。
使用 win32ui
设置打印格式
import win32print
import win32ui
from PIL import Image, ImageWin
def print_file_with_format(file_path):
# 打开打印机
printer_name = win32print.GetDefaultPrinter()
hprinter = win32print.OpenPrinter(printer_name)
# 开始打印任务
job = win32print.StartDocPrinter(hprinter, 1, ("Python Printing", None, "RAW"))
win32print.StartPagePrinter(hprinter)
# 设置字体和格式
hdc = win32ui.CreateDC()
hdc.CreatePrinterDC(printer_name)
hdc.StartDoc("Printing Document")
hdc.StartPage()
# 设置字体
font = win32ui.CreateFont({
"name": "Arial",
"height": 10,
"weight": 400
})
hdc.SelectObject(font)
# 读取文件内容
with open(file_path, 'r') as file:
lines = file.readlines()
# 打印每一行
y = 0
for line in lines:
hdc.TextOut(0, y, line.strip())
y += 12 # 行间距
# 结束打印任务
hdc.EndPage()
hdc.EndDoc()
win32print.EndPagePrinter(hprinter)
win32print.EndDocPrinter(hprinter)
win32print.ClosePrinter(hprinter)
示例用法
print_file_with_format('example.py')
上述代码中,我们使用 win32ui
库设置了打印字体和行间距,并通过 TextOut
方法逐行打印文件内容。这样可以确保打印出来的代码格式整齐、可读性高。
五、处理不同操作系统的差异
不同操作系统的打印命令和方法存在差异,我们需要根据操作系统选择合适的方法。
检测操作系统
import os
def get_os():
if os.name == 'nt':
return 'Windows'
elif os.name == 'posix':
return 'Linux/Mac'
else:
return 'Unknown'
示例用法
current_os = get_os()
print(f"Current operating system: {current_os}")
针对不同操作系统的打印方法
import subprocess
def print_file(file_path):
current_os = get_os()
if current_os == 'Windows':
subprocess.run(['notepad', '/p', file_path])
elif current_os == 'Linux/Mac':
subprocess.run(['lp', file_path])
else:
print("Unsupported operating system")
示例用法
print_file('example.py')
在上述代码中,我们根据操作系统选择不同的打印命令。Windows 使用 notepad /p
,而 Linux 和 Mac 使用 lp
命令。
六、处理大型文件的打印
对于大型文件,我们需要考虑分段打印和内存管理,以避免占用过多资源。
分段读取文件
def print_large_file(file_path):
current_os = get_os()
if current_os == 'Windows':
printer_name = win32print.GetDefaultPrinter()
hprinter = win32print.OpenPrinter(printer_name)
job = win32print.StartDocPrinter(hprinter, 1, ("Python Printing", None, "RAW"))
win32print.StartPagePrinter(hprinter)
with open(file_path, 'r') as file:
for line in file:
win32print.WritePrinter(hprinter, line.encode('utf-8'))
win32print.EndPagePrinter(hprinter)
win32print.EndDocPrinter(hprinter)
win32print.ClosePrinter(hprinter)
elif current_os == 'Linux/Mac':
subprocess.run(['lp', file_path])
else:
print("Unsupported operating system")
示例用法
print_large_file('large_example.py')
在上述代码中,我们逐行读取并打印大型文件,以减少内存占用。
七、总结
本文详细介绍了在Python中使用打印机打印代码的多种方法,包括使用操作系统命令、第三方库 win32print
、将代码保存为文件后打印,并设置打印格式和处理不同操作系统的差异。通过这些方法,我们可以灵活、高效地实现代码打印。希望本文对您有所帮助。
相关问答FAQs:
如何在Python中选择打印机进行打印?
在Python中,您可以使用win32print
模块来选择打印机。通过调用win32print.EnumPrinters
函数,您可以获取系统中所有可用打印机的列表。接着,选择您要使用的打印机,并在打印文档时指定其名称。
可以通过Python打印哪些类型的文档?
Python能够打印多种类型的文档,包括文本文件、PDF、图像和其他格式。使用合适的库(如reportlab
用于PDF生成,Pillow
用于图像处理)可以帮助您将这些文件转换为可打印的格式,并利用打印机进行输出。
如何在打印时设置打印选项?
在Python中,可以通过win32print
模块设置打印选项,例如纸张大小、打印方向和复印数量。使用DEVMODE
结构体可以自定义这些选项。确保在发送打印任务之前正确配置这些参数,以达到所需的打印效果。
如何处理打印错误或异常?
在打印过程中,可能会遇到各种错误,例如打印机未连接、纸张卡住或驱动程序问题。通过try-except语句捕获这些异常,您可以实施相应的错误处理逻辑,比如重新连接打印机、提示用户更换纸张或检查驱动程序设置。
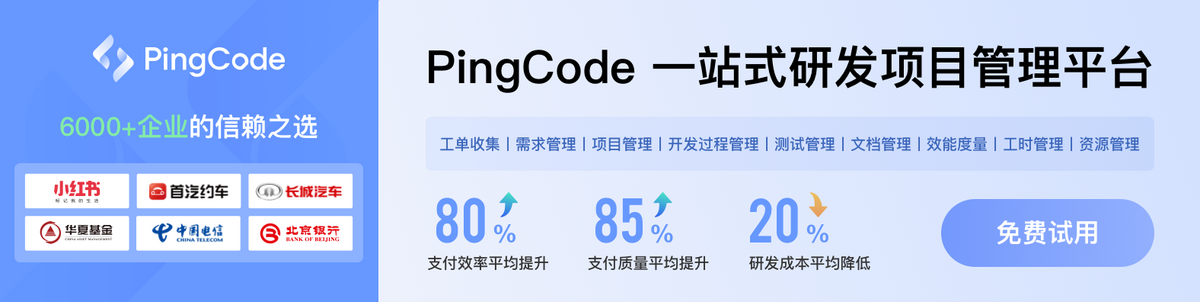