要用Python抓取材料价格,可以使用网页抓取技术、API调用和数据解析技术。通过Python中的Requests库获取网页内容、BeautifulSoup库解析网页结构、Selenium库模拟浏览器操作、API获取材料价格。下面将详细介绍这些方法的使用。
一、网页抓取技术
网页抓取技术是从网页上提取数据的一种方法。Python提供了许多库来帮助我们实现这一目标,其中最常用的是Requests库和BeautifulSoup库。
1、使用Requests库获取网页内容
Requests库是Python中用于发送HTTP请求的库,它可以轻松地获取网页的HTML内容。以下是一个简单的示例:
import requests
url = 'https://www.example.com/material-prices'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print('Failed to retrieve the webpage')
在上面的代码中,我们首先导入Requests库,然后使用requests.get()
方法发送一个GET请求以获取网页内容。如果请求成功(状态码为200),我们将HTML内容存储在html_content
变量中。
2、使用BeautifulSoup库解析网页结构
BeautifulSoup库是一种用于解析HTML和XML文档的库。它提供了许多方便的方法来查找和提取网页中的数据。以下是一个示例:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
material_prices = soup.find_all('div', class_='material-price')
for price in material_prices:
material_name = price.find('span', class_='material-name').text
material_cost = price.find('span', class_='material-cost').text
print(f'{material_name}: {material_cost}')
在上面的代码中,我们首先将HTML内容传递给BeautifulSoup对象,并指定解析器为html.parser
。然后,我们使用find_all()
方法查找所有包含材料价格的div
标签,并遍历每个标签以提取材料名称和价格。
二、API调用
许多网站提供API供用户获取数据。通过API调用,我们可以直接获取材料价格数据,而无需解析网页内容。
1、查找API并获取API密钥
首先,我们需要查找提供材料价格数据的API。例如,某些材料供应商或价格监控平台可能提供API。通常,我们需要注册并获取API密钥,以便能够调用API。
2、使用Requests库调用API
一旦我们获得了API密钥,就可以使用Requests库来调用API并获取数据。以下是一个示例:
import requests
api_url = 'https://api.example.com/material-prices'
api_key = 'your_api_key_here'
headers = {
'Authorization': f'Bearer {api_key}'
}
response = requests.get(api_url, headers=headers)
if response.status_code == 200:
data = response.json()
for item in data['materials']:
material_name = item['name']
material_cost = item['price']
print(f'{material_name}: {material_cost}')
else:
print('Failed to retrieve the API data')
在上面的代码中,我们首先定义API的URL和API密钥,然后使用requests.get()
方法发送GET请求,并在请求头中包含API密钥。如果请求成功(状态码为200),我们将响应数据解析为JSON格式,并遍历每个材料项以提取材料名称和价格。
三、使用Selenium库模拟浏览器操作
在某些情况下,材料价格数据可能通过动态加载的JavaScript生成,这时我们需要使用Selenium库来模拟浏览器操作,获取动态加载的内容。
1、安装Selenium和WebDriver
首先,我们需要安装Selenium库和相应的WebDriver。例如,使用Chrome浏览器时,可以安装ChromeDriver。
pip install selenium
然后,下载并安装ChromeDriver,并将其路径添加到系统环境变量中。
2、使用Selenium获取动态加载的内容
以下是一个示例,展示如何使用Selenium来获取动态加载的材料价格数据:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
设置ChromeDriver路径
service = Service(ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
url = 'https://www.example.com/material-prices'
driver.get(url)
等待页面加载完成
driver.implicitly_wait(10)
material_prices = driver.find_elements(By.CLASS_NAME, 'material-price')
for price in material_prices:
material_name = price.find_element(By.CLASS_NAME, 'material-name').text
material_cost = price.find_element(By.CLASS_NAME, 'material-cost').text
print(f'{material_name}: {material_cost}')
driver.quit()
在上面的代码中,我们首先导入Selenium库和ChromeDriver管理器,然后启动Chrome浏览器并访问目标网址。接着,我们使用find_elements()
方法查找所有包含材料价格的元素,并提取材料名称和价格。最后,关闭浏览器。
四、数据解析与存储
获取材料价格数据后,我们通常需要对数据进行解析和存储,以便后续使用。以下是一些常见的数据解析和存储方法。
1、解析JSON数据
如果我们通过API获取的数据是JSON格式,可以使用Python的内置json
库来解析数据。例如:
import json
response_data = '{"materials": [{"name": "Steel", "price": "1000"}, {"name": "Aluminum", "price": "1500"}]}'
data = json.loads(response_data)
for item in data['materials']:
material_name = item['name']
material_cost = item['price']
print(f'{material_name}: {material_cost}')
在上面的代码中,我们使用json.loads()
方法将JSON字符串解析为Python字典,并遍历每个材料项以提取材料名称和价格。
2、存储数据到CSV文件
我们可以使用Python的csv
库将材料价格数据存储到CSV文件中。例如:
import csv
materials = [
{'name': 'Steel', 'price': '1000'},
{'name': 'Aluminum', 'price': '1500'}
]
with open('material_prices.csv', mode='w', newline='') as file:
writer = csv.DictWriter(file, fieldnames=['name', 'price'])
writer.writeheader()
for material in materials:
writer.writerow(material)
在上面的代码中,我们首先定义材料价格数据,然后使用csv.DictWriter()
方法创建CSV写入器,并将数据写入CSV文件。
3、存储数据到数据库
我们还可以将材料价格数据存储到数据库中。以下是一个示例,展示如何使用SQLite数据库存储数据:
import sqlite3
创建数据库连接
conn = sqlite3.connect('material_prices.db')
cursor = conn.cursor()
创建表
cursor.execute('''
CREATE TABLE IF NOT EXISTS materials (
id INTEGER PRIMARY KEY,
name TEXT,
price TEXT
)
''')
插入数据
materials = [
('Steel', '1000'),
('Aluminum', '1500')
]
cursor.executemany('INSERT INTO materials (name, price) VALUES (?, ?)', materials)
提交更改并关闭连接
conn.commit()
conn.close()
在上面的代码中,我们首先创建数据库连接和游标,然后创建一个名为materials
的表,并插入材料价格数据。最后,提交更改并关闭数据库连接。
五、综合实例
为了更好地理解如何使用Python抓取材料价格,我们将上述方法综合起来,展示一个完整的实例。
import requests
from bs4 import BeautifulSoup
import csv
import sqlite3
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
使用Requests库获取网页内容
def get_html_content(url):
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
raise Exception('Failed to retrieve the webpage')
使用BeautifulSoup库解析网页结构
def parse_html_content(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
material_prices = soup.find_all('div', class_='material-price')
materials = []
for price in material_prices:
material_name = price.find('span', class_='material-name').text
material_cost = price.find('span', class_='material-cost').text
materials.append({'name': material_name, 'price': material_cost})
return materials
使用Selenium库获取动态加载的内容
def get_dynamic_content(url):
service = Service(ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
driver.get(url)
driver.implicitly_wait(10)
material_prices = driver.find_elements(By.CLASS_NAME, 'material-price')
materials = []
for price in material_prices:
material_name = price.find_element(By.CLASS_NAME, 'material-name').text
material_cost = price.find_element(By.CLASS_NAME, 'material-cost').text
materials.append({'name': material_name, 'price': material_cost})
driver.quit()
return materials
存储数据到CSV文件
def save_to_csv(materials, filename):
with open(filename, mode='w', newline='') as file:
writer = csv.DictWriter(file, fieldnames=['name', 'price'])
writer.writeheader()
for material in materials:
writer.writerow(material)
存储数据到数据库
def save_to_db(materials, db_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('''
CREATE TABLE IF NOT EXISTS materials (
id INTEGER PRIMARY KEY,
name TEXT,
price TEXT
)
''')
cursor.executemany('INSERT INTO materials (name, price) VALUES (?, ?)', [(m['name'], m['price']) for m in materials])
conn.commit()
conn.close()
主函数
def main():
url = 'https://www.example.com/material-prices'
try:
html_content = get_html_content(url)
materials = parse_html_content(html_content)
except Exception as e:
print(f'Failed to retrieve and parse static content: {e}')
try:
materials = get_dynamic_content(url)
except Exception as e:
print(f'Failed to retrieve dynamic content: {e}')
return
save_to_csv(materials, 'material_prices.csv')
save_to_db(materials, 'material_prices.db')
print('Data has been successfully saved to CSV and database.')
if __name__ == '__main__':
main()
在这个综合实例中,我们首先尝试使用Requests库获取网页内容,并使用BeautifulSoup库解析网页结构。如果失败,我们将使用Selenium库获取动态加载的内容。然后,我们将材料价格数据存储到CSV文件和SQLite数据库中。
通过上述步骤,我们可以使用Python抓取材料价格,并将数据存储到文件或数据库中,以便后续分析和使用。这种方法可以应用于各种材料价格抓取场景,帮助我们自动化数据采集过程。
相关问答FAQs:
如何使用Python抓取特定材料的实时价格?
要抓取特定材料的实时价格,您可以使用Python中的requests
库来发送HTTP请求,结合BeautifulSoup
库解析网页内容。您需要找到提供材料价格的网页,确保该网页允许抓取(遵循robots.txt规定)。通过分析网页的HTML结构,您可以找到价格信息所在的标签,并提取所需数据。
抓取材料价格时,应该注意哪些法律和道德问题?
在抓取材料价格时,应确保遵循网站的使用条款和条件,避免对其服务器造成过大负担。此外,尊重网站的robots.txt文件,确保不抓取禁止的内容。保持数据的合法使用,避免侵犯版权或个人隐私,以维护良好的网络道德。
如何处理抓取过程中遇到的反爬虫机制?
如果在抓取过程中遇到反爬虫机制,可以尝试多种策略来规避这些限制。例如,使用User-Agent
伪装成浏览器请求,设定请求间隔以模拟人类行为,或者使用代理IP来分散请求来源。此外,可以考虑利用一些自动化工具如Selenium
来处理更复杂的网页抓取情况。
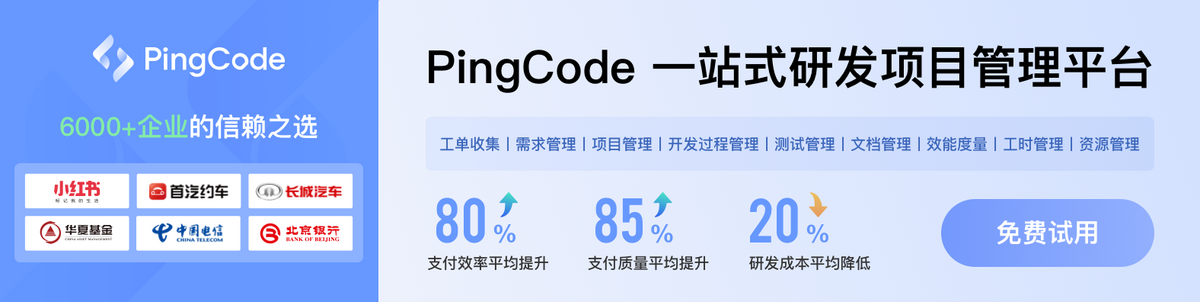