在Python程序中查找关键词的方法包括:使用内置字符串方法、正则表达式、外部库。
使用内置字符串方法是一种直接且易理解的方式。Python的字符串方法find()
、index()
、和count()
可以帮助你在字符串中查找子字符串的位置和出现次数。例如,如果你需要查找一个关键词在一个字符串中的位置,可以使用find()
方法,它会返回关键词的第一个字符的索引,如果关键词不存在,则返回-1。
text = "This is a sample text with sample keyword."
keyword = "sample"
position = text.find(keyword)
if position != -1:
print(f"Keyword '{keyword}' found at position {position}")
else:
print(f"Keyword '{keyword}' not found")
一、内置字符串方法
Python提供了多种内置字符串方法,这些方法可以帮助我们在字符串中查找关键词。
1、find()方法
find()
方法用于查找子字符串在字符串中的位置。它返回子字符串第一次出现的位置,如果未找到则返回-1。
text = "This is a sample text with sample keyword."
keyword = "sample"
position = text.find(keyword)
if position != -1:
print(f"Keyword '{keyword}' found at position {position}")
else:
print(f"Keyword '{keyword}' not found")
这个例子中,我们在字符串text
中查找关键词sample
的位置。find()
方法返回的是sample
第一次出现的位置。
2、index()方法
index()
方法与find()
方法类似,只是如果子字符串未找到,它会引发一个ValueError
异常。
try:
position = text.index(keyword)
print(f"Keyword '{keyword}' found at position {position}")
except ValueError:
print(f"Keyword '{keyword}' not found")
使用index()
方法可以在未找到关键词时引发异常,从而进行异常处理。
3、count()方法
count()
方法用于计算子字符串在字符串中出现的次数。
count = text.count(keyword)
print(f"Keyword '{keyword}' found {count} times")
这个例子中,我们计算关键词sample
在字符串text
中出现的次数。
二、正则表达式
正则表达式是处理字符串的强大工具。Python的re
模块提供了使用正则表达式的功能。
1、re.search()方法
re.search()
方法用于查找字符串中符合正则表达式的子字符串。如果找到则返回一个匹配对象,否则返回None。
import re
pattern = re.compile(r'sample')
match = pattern.search(text)
if match:
print(f"Keyword '{match.group()}' found at position {match.start()}")
else:
print(f"Keyword not found")
这个例子中,我们使用正则表达式sample
在字符串text
中查找关键词。
2、re.findall()方法
re.findall()
方法返回所有与正则表达式匹配的子字符串列表。
matches = pattern.findall(text)
print(f"Keyword found {len(matches)} times")
这个例子中,我们查找所有匹配的子字符串,并计算它们的数量。
3、re.finditer()方法
re.finditer()
方法返回一个迭代器,迭代器包含所有匹配的匹配对象。
for match in pattern.finditer(text):
print(f"Keyword '{match.group()}' found at position {match.start()}")
使用re.finditer()
可以迭代所有匹配的子字符串,并获取它们的位置。
三、外部库
除了内置方法和正则表达式,Python还有一些外部库可以帮助我们查找关键词。
1、Whoosh
Whoosh是一个快速、功能丰富的全文搜索库。它适用于需要对大量文本数据进行快速搜索的应用。
from whoosh.index import create_in
from whoosh.fields import Schema, TEXT
from whoosh.qparser import QueryParser
创建索引
schema = Schema(content=TEXT)
index = create_in("indexdir", schema)
writer = index.writer()
writer.add_document(content="This is a sample text with sample keyword.")
writer.commit()
搜索关键词
searcher = index.searcher()
query = QueryParser("content", index.schema).parse("sample")
results = searcher.search(query)
for result in results:
print(f"Keyword '{result['content']}' found")
这个例子中,我们使用Whoosh创建一个索引,并搜索关键词sample
。
2、NLTK
自然语言处理工具包(NLTK)是一个强大的文本处理库。它提供了丰富的文本处理功能,包括查找关键词。
import nltk
from nltk.tokenize import word_tokenize
text = "This is a sample text with sample keyword."
keywords = word_tokenize(text)
keyword = "sample"
if keyword in keywords:
print(f"Keyword '{keyword}' found")
else:
print(f"Keyword '{keyword}' not found")
这个例子中,我们使用NLTK的word_tokenize()
方法将文本分词,并查找关键词。
四、实现复杂的关键词查找
在实际应用中,我们可能需要实现更复杂的关键词查找。下面是一些实现复杂关键词查找的方法:
1、模糊匹配
模糊匹配允许我们查找与关键词相似的字符串。Python的difflib
模块提供了模糊匹配的功能。
import difflib
text = "This is a sample text with sample keyword."
keyword = "sampl"
matches = difflib.get_close_matches(keyword, text.split())
print(f"Close matches for '{keyword}': {matches}")
这个例子中,我们使用difflib.get_close_matches()
查找与关键词相似的字符串。
2、关键词高亮
在查找到关键词后,我们可能需要对其进行高亮显示。
import re
text = "This is a sample text with sample keyword."
keyword = "sample"
highlighted_text = re.sub(f"({keyword})", r'\033[1;31m\1\033[0m', text)
print(highlighted_text)
这个例子中,我们使用正则表达式将关键词高亮显示。
3、上下文查找
在查找关键词时,我们可能需要获取关键词的上下文信息。
import re
text = "This is a sample text with sample keyword."
keyword = "sample"
pattern = re.compile(rf'.{{0,10}}{keyword}.{{0,10}}')
matches = pattern.findall(text)
for match in matches:
print(f"Context: {match}")
这个例子中,我们使用正则表达式查找包含关键词的上下文信息。
五、性能优化
在处理大量文本数据时,性能是一个重要的考虑因素。以下是一些优化关键词查找性能的方法:
1、使用索引
创建索引可以显著提高关键词查找的性能。上面提到的Whoosh库就是一个创建索引的例子。
2、分词和倒排索引
分词和倒排索引是搜索引擎中常用的技术。分词将文本分割成单词,倒排索引记录每个单词在文档中的位置。
from collections import defaultdict
text = "This is a sample text with sample keyword."
words = text.split()
index = defaultdict(list)
for position, word in enumerate(words):
index[word].append(position)
keyword = "sample"
if keyword in index:
print(f"Keyword '{keyword}' found at positions {index[keyword]}")
else:
print(f"Keyword '{keyword}' not found")
这个例子中,我们创建了一个倒排索引,并使用它查找关键词的位置。
3、并行处理
对于非常大的文本数据,可以使用并行处理来提高性能。Python的multiprocessing
模块提供了并行处理的功能。
from multiprocessing import Pool
def find_keyword(text, keyword):
if keyword in text:
return text
return None
texts = [
"This is a sample text with sample keyword.",
"Another text without the keyword.",
"Yet another sample text."
]
keyword = "sample"
with Pool() as pool:
results = pool.starmap(find_keyword, [(text, keyword) for text in texts])
results = [result for result in results if result]
print(f"Texts containing '{keyword}': {results}")
这个例子中,我们使用多进程并行查找关键词。
结论
在Python中查找程序中的关键词可以通过多种方法实现,包括内置字符串方法、正则表达式、外部库等。选择合适的方法取决于具体的应用场景和性能要求。通过优化关键词查找的性能,可以在处理大量文本数据时获得更好的效果。
相关问答FAQs:
如何在Python程序中有效查找关键词?
在Python中,可以使用内置的字符串方法如str.find()
和str.index()
来查找关键词。这些方法可以帮助您确定关键词在字符串中的位置。另一个选择是使用正则表达式模块re
,它提供了更多灵活性,尤其适用于复杂的搜索需求。
是否可以在文件中查找关键词?
当然可以!您可以打开文件并读取其内容,然后使用字符串方法或正则表达式来查找关键词。示例代码如下:
with open('yourfile.txt', 'r') as file:
content = file.read()
if 'keyword' in content:
print("关键词存在于文件中")
这种方式适用于文本文件的简单搜索。
如何处理大小写不敏感的关键词查找?
进行不区分大小写的关键词查找时,可以将字符串转换为统一的大小写。使用str.lower()
或str.upper()
方法,可以确保关键词的查找不受大小写影响。示例:
content = "Hello World"
keyword = "hello"
if keyword.lower() in content.lower():
print("找到关键词!")
这种技巧在处理用户输入或不规范数据时特别有用。
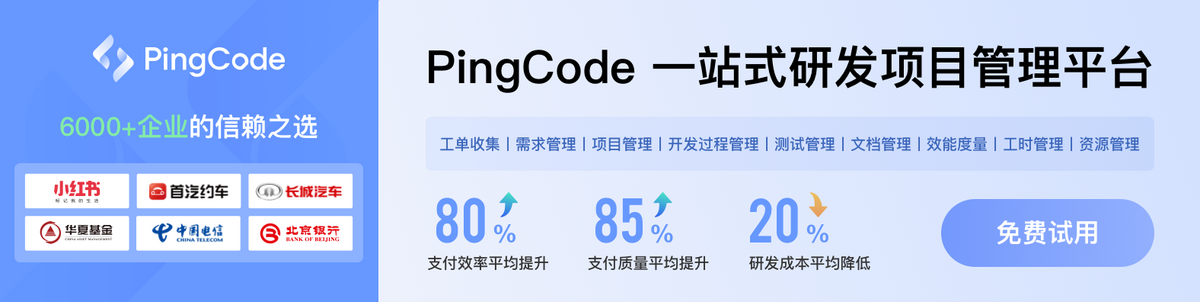