Python将文件内容转成字典的方法有很多种,常见的方法包括:使用json
模块处理JSON文件、使用yaml
模块处理YAML文件、手动解析格式化文本、使用configparser
模块处理配置文件。 以下是详细的描述和示例代码。
一、使用 json
模块处理 JSON 文件
1、读取 JSON 文件并转成字典
读取 JSON 文件是将文件内容转成字典最常见的方法之一。Python 提供了内置的 json
模块来处理 JSON 格式的数据。首先,将 JSON 文件的内容读取到一个字符串中,然后使用 json.loads
函数将字符串解析为字典。
import json
def read_json_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
data = json.load(file)
return data
示例用法
file_path = 'data.json'
data_dict = read_json_file(file_path)
print(data_dict)
二、使用 yaml
模块处理 YAML 文件
1、读取 YAML 文件并转成字典
YAML 是一种人类可读的数据序列化标准,常用于配置文件。可以使用 PyYAML
库来处理 YAML 文件。首先,将 YAML 文件内容读取到一个字符串中,然后使用 yaml.safe_load
函数将字符串解析为字典。
import yaml
def read_yaml_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
data = yaml.safe_load(file)
return data
示例用法
file_path = 'config.yaml'
data_dict = read_yaml_file(file_path)
print(data_dict)
三、手动解析格式化文本文件
1、读取键值对格式的文本文件
有些文本文件包含键值对格式的数据,可以手动解析这些文件并将其转换为字典。下面是一个解析简单键值对格式文件的示例:
def read_kv_file(file_path):
data_dict = {}
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
key, value = line.strip().split('=')
data_dict[key] = value
return data_dict
示例用法
file_path = 'settings.txt'
data_dict = read_kv_file(file_path)
print(data_dict)
四、使用 configparser
模块处理配置文件
1、读取 INI 配置文件
INI 文件是一种常见的配置文件格式,可以使用 Python 内置的 configparser
模块来解析。首先,读取配置文件内容,然后使用 configparser
模块将其转换为字典。
import configparser
def read_ini_file(file_path):
config = configparser.ConfigParser()
config.read(file_path)
data_dict = {section: dict(config.items(section)) for section in config.sections()}
return data_dict
示例用法
file_path = 'config.ini'
data_dict = read_ini_file(file_path)
print(data_dict)
五、处理 CSV 文件
1、读取 CSV 文件并转成字典列表
CSV 文件是另一种常见的文件格式,可以使用 csv
模块来处理。可以将 CSV 文件的每一行解析为字典,并将这些字典存储在一个列表中。
import csv
def read_csv_file(file_path):
data_list = []
with open(file_path, 'r', encoding='utf-8') as file:
reader = csv.DictReader(file)
for row in reader:
data_list.append(row)
return data_list
示例用法
file_path = 'data.csv'
data_dict_list = read_csv_file(file_path)
print(data_dict_list)
六、处理 XML 文件
1、读取 XML 文件并转成字典
XML 是一种常见的数据交换格式,可以使用 xml.etree.ElementTree
模块来解析 XML 文件,并将其转换为字典。
import xml.etree.ElementTree as ET
def xml_to_dict(element):
if len(element) == 0:
return element.text
return {child.tag: xml_to_dict(child) for child in element}
def read_xml_file(file_path):
tree = ET.parse(file_path)
root = tree.getroot()
return {root.tag: xml_to_dict(root)}
示例用法
file_path = 'data.xml'
data_dict = read_xml_file(file_path)
print(data_dict)
七、处理自定义格式文件
有些文件格式可能不是标准格式,需要手动解析。以下是一个解析自定义格式文件的示例:
def read_custom_file(file_path):
data_dict = {}
with open(file_path, 'r', encoding='utf-8') as file:
lines = file.readlines()
for line in lines:
key, value = parse_custom_format(line)
data_dict[key] = value
return data_dict
def parse_custom_format(line):
# 自定义解析逻辑
key, value = line.strip().split(':')
return key, value
示例用法
file_path = 'custom_format.txt'
data_dict = read_custom_file(file_path)
print(data_dict)
八、总结
将文件内容转换为字典的方式多种多样,选择适合的方式可以大大简化工作。常见的方法包括使用 json
模块处理 JSON 文件、使用 yaml
模块处理 YAML 文件、手动解析格式化文本、使用 configparser
模块处理配置文件,以及处理 CSV 和 XML 文件。 根据实际需求选择合适的方法,可以提高开发效率和代码可读性。
相关问答FAQs:
如何将文本文件中的每一行转换为字典的键值对?
可以使用Python的内置函数读取文件内容,并通过遍历每一行来构建字典。假设每行包含一个键和一个值,可以使用split()方法将其分开。例如,文件内容为:
name: John
age: 30
city: New York
可以使用以下代码将其转换为字典:
data_dict = {}
with open('file.txt', 'r') as file:
for line in file:
key, value = line.strip().split(': ')
data_dict[key] = value
如何处理包含嵌套结构的JSON文件并将其转换为字典?
如果文件是JSON格式,可以使用Python的json模块轻松将其转换为字典。读取JSON文件时,可以使用load()方法。示例如下:
import json
with open('file.json', 'r') as file:
data_dict = json.load(file)
此方法会自动处理嵌套结构,使数据更加易于操作。
如果文件内容格式不规则,如何安全地转换为字典?
对于格式不规则的文件,建议使用异常处理机制来确保程序的健壮性。通过try-except结构捕获可能出现的错误,避免程序因单行格式错误而崩溃。代码示例:
data_dict = {}
with open('file.txt', 'r') as file:
for line in file:
try:
key, value = line.strip().split(': ')
data_dict[key] = value
except ValueError:
print(f"Skipping line due to format error: {line.strip()}")
这种方式可以确保文件转换的完整性,并及时处理错误。
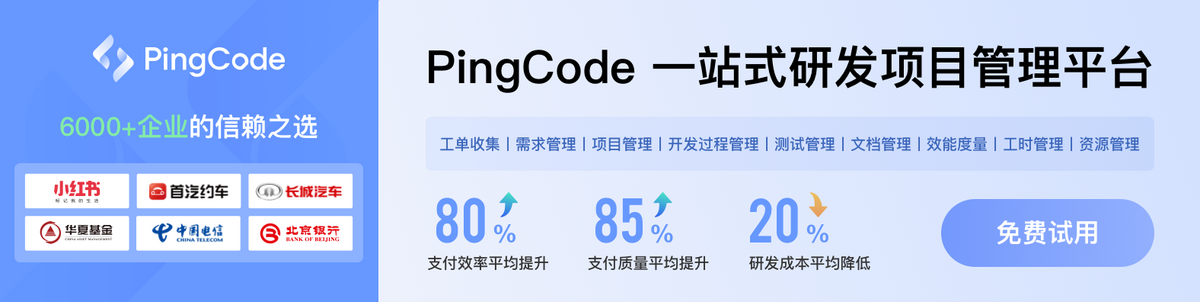