Python爬取B站网页代码的方法包括使用requests库获取网页内容、使用BeautifulSoup库解析HTML、模拟用户行为、处理异步加载内容等。通过使用requests库获取网页内容是最简单的方式,但如果网页内容是通过JavaScript动态加载的,则需要借助Selenium库来模拟浏览器行为。
一、使用requests库获取网页内容
requests库是一个简单易用的HTTP库,可以用来发送HTTP请求并获取响应数据。
import requests
url = 'https://www.bilibili.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/85.0.4183.121 Safari/537.36'
}
response = requests.get(url, headers=headers)
print(response.text)
在上面的代码中,通过设置User-Agent来模拟浏览器请求,获取B站主页的HTML内容。
二、使用BeautifulSoup解析HTML
BeautifulSoup是一个用于解析HTML和XML文档的Python库,可以用来提取网页中的有用信息。
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
titles = soup.find_all('title')
for title in titles:
print(title.get_text())
通过使用BeautifulSoup解析获取的HTML内容,可以提取网页中的标题信息。
三、模拟用户行为
某些网页内容需要模拟用户行为才能获取,例如需要点击某个按钮或者滚动页面。这个时候可以使用Selenium库来模拟用户操作。
from selenium import webdriver
url = 'https://www.bilibili.com'
driver = webdriver.Chrome()
driver.get(url)
模拟滚动页面
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
html = driver.page_source
print(html)
driver.quit()
使用Selenium可以打开浏览器窗口,加载页面并执行JavaScript,从而获取动态加载的内容。
四、处理异步加载内容
B站的某些内容是通过异步请求加载的,这时候需要分析网页请求,找到对应的API接口。
url = 'https://api.bilibili.com/x/web-interface/popular?ps=20&pn=1'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/85.0.4183.121 Safari/537.36',
'Referer': 'https://www.bilibili.com/'
}
response = requests.get(url, headers=headers)
data = response.json()
for video in data['data']['list']:
print(video['title'])
在上面的代码中,通过分析B站的API接口,可以直接获取热门视频的标题信息。
五、常见问题与解决方法
1、反爬虫机制
B站有一定的反爬虫机制,可能会限制频繁的请求。可以通过设置请求头、使用代理IP、增加请求间隔等方式来绕过反爬虫机制。
import time
import random
time.sleep(random.uniform(1, 3))
通过增加随机的请求间隔,可以降低被识别为爬虫的风险。
2、验证码问题
某些操作可能会触发验证码,这时候可以使用OCR技术识别验证码,或者手动输入验证码。
from PIL import Image
import pytesseract
image = Image.open('captcha.png')
captcha = pytesseract.image_to_string(image)
print(captcha)
使用pytesseract库可以识别图片中的文字,从而解决验证码问题。
六、总结
通过使用requests库获取网页内容、使用BeautifulSoup解析HTML、模拟用户行为、处理异步加载内容等方法,可以有效地爬取B站的网页代码。同时需要注意绕过反爬虫机制和处理验证码问题。希望本文能够帮助你掌握Python爬取B站网页代码的方法。
七、示例代码整合
以下是一个整合了上述方法的示例代码,展示了如何爬取B站的热门视频标题。
import requests
from bs4 import BeautifulSoup
from selenium import webdriver
import time
import random
def get_html(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/85.0.4183.121 Safari/537.36'
}
response = requests.get(url, headers=headers)
return response.text
def parse_html(html):
soup = BeautifulSoup(html, 'html.parser')
titles = soup.find_all('title')
for title in titles:
print(title.get_text())
def get_dynamic_html(url):
driver = webdriver.Chrome()
driver.get(url)
time.sleep(random.uniform(1, 3))
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
html = driver.page_source
driver.quit()
return html
def get_popular_videos():
url = 'https://api.bilibili.com/x/web-interface/popular?ps=20&pn=1'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/85.0.4183.121 Safari/537.36',
'Referer': 'https://www.bilibili.com/'
}
response = requests.get(url, headers=headers)
data = response.json()
for video in data['data']['list']:
print(video['title'])
if __name__ == '__main__':
url = 'https://www.bilibili.com'
html = get_html(url)
parse_html(html)
dynamic_html = get_dynamic_html(url)
parse_html(dynamic_html)
get_popular_videos()
通过整合上述方法,可以更全面地爬取B站的网页内容。希望本文能够帮助你掌握Python爬取B站网页代码的方法,提升你的爬虫技术水平。
相关问答FAQs:
如何使用Python获取b站的网页源代码?
要获取b站网页的源代码,可以使用Python中的requests库。首先,确保已经安装requests库。接下来,使用requests.get()方法发送请求,并获取响应内容。示例代码如下:
import requests
url = 'https://www.bilibili.com/'
response = requests.get(url)
html_content = response.text
print(html_content)
运行上述代码后,就可以看到b站首页的HTML源代码。
在爬取b站网页时需要注意哪些问题?
在爬取b站网页时,需遵循网站的robots.txt协议,避免对网站造成负担。此外,合理设置请求间隔,防止被视为恶意爬虫。同时,使用适当的User-Agent头部信息,伪装请求,增加爬取成功的几率。
如何解析b站网页代码中的特定信息?
获取到b站网页的源代码后,可以使用BeautifulSoup库进行解析。通过查找特定的HTML标签和属性,可以提取所需的信息。例如,以下代码演示了如何提取b站视频标题:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
titles = soup.find_all('h1', class_='title')
for title in titles:
print(title.get_text())
确保安装了BeautifulSoup库,并根据实际情况调整解析逻辑以适应b站的网页结构。
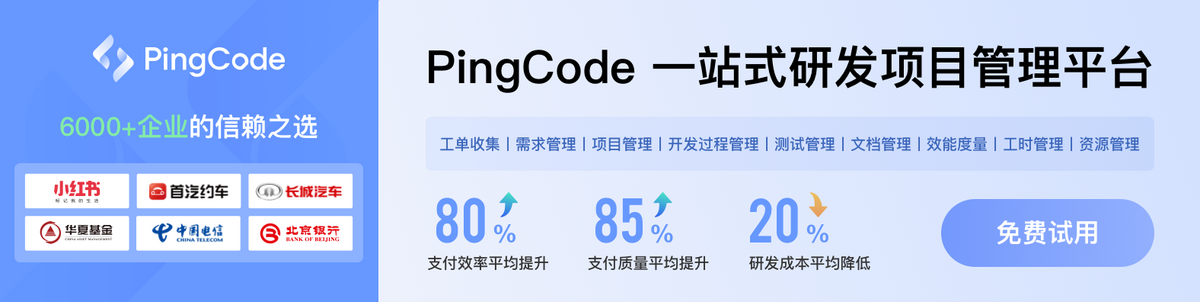